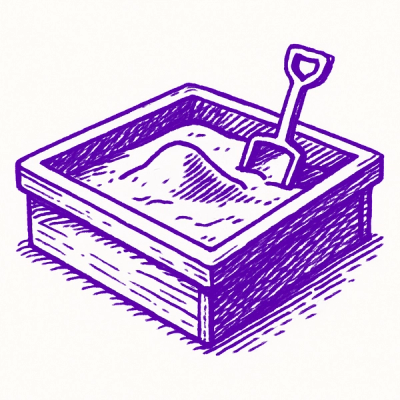
Research
/Security News
Critical Vulnerability in NestJS Devtools: Localhost RCE via Sandbox Escape
A flawed sandbox in @nestjs/devtools-integration lets attackers run code on your machine via CSRF, leading to full Remote Code Execution (RCE).
touch-simulate
Advanced tools
Simulate touch event(A fake one with pageX, pageY, clientX, clientY available) even at desktop browser for testing.
You can have touches by e.touches[0]
e.changedTouches[0]
or e.targetTouches[0]
, they are the same.
Already used for testing my components like iscroll, pull-to-refresh and sweet-sortable
e.clientX
, e.clientY
and many othersnpm i touch-simulate -D
var el = document.getElementById('demo')
var TouchSimulate = require('touch-simulate')
moveable(el)
var touch = new TouchSimulate(el, {
point: true
})
touch.start() // fire touchstart at center of element
.moveRight(150, false) // move right 150px, no touchend event
.wait(100) // wait 100ms
.moveDown(150, false)
.wait(100)
.moveLeft(150, false)
.wait(100)
.moveUp(150) // move up 150px and fire touchend
You can chain the methods, the function call would wait for previous one to be fullfilled, the chainable method list:
start
moveUp
moveDown
moveLeft
moveRight
moveTo
move
wait
Create TouchSimulate instance with element and options
option.speed
set speed of px/s
default 40option.fixTarget
use the real target at the point instead of el
to dispatch the eventoption.point
show a transparent point at the screen, can not use with fixTarget
option.ease
define a ease function for the movemonent, default linear
Set the speed to number
Set the ease function name
Set the touch start el and optional position(default is center)
position could be t
l
r
b
for alias for top, left, right and bottom
position could also be an array, which contains [x, y] for clientX and clientY
This function would throw error if the movemonent not finished
If not started, start at the center of element, or the current position
Move to one direction, return promise which resolved with event of touchend
If end
is set to false, no touchend event is fired.
Insread of move direction, set move monent destination, x and y are clientX and clientY (relative to viewport, regardless of scrollbar)
Use angel
which should be (0 ~ 2*PI) instead of up
down
left
nad right
Helper to emit tap event, return promise, resolved with event of touchend
Helper to return a promise after wait for ms
FAQs
simulate touch event without browser support touch
The npm package touch-simulate receives a total of 2,111 weekly downloads. As such, touch-simulate popularity was classified as popular.
We found that touch-simulate demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
/Security News
A flawed sandbox in @nestjs/devtools-integration lets attackers run code on your machine via CSRF, leading to full Remote Code Execution (RCE).
Product
Customize license detection with Socket’s new license overlays: gain control, reduce noise, and handle edge cases with precision.
Product
Socket now supports Rust and Cargo, offering package search for all users and experimental SBOM generation for enterprise projects.