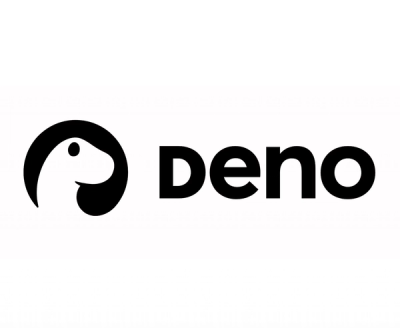
Security News
Deno 2.4 Brings Back deno bundle, Improves Dependency Management and Observability
Deno 2.4 brings back bundling, improves dependency updates and telemetry, and makes the runtime more practical for real-world JavaScript projects.
trie-structure
Advanced tools
An implementation of a Trie
The Trie can either be created string by string or from an array of strings.
import { Trie } from "trie-structure";
const trie = new Trie();
const strings = ["he", "hello", "helios", "woof", "dog", "doom"];
trie.addMany(strings);
const allWords = trie.getAllWords(); // => ["he", "hello", "helios", "woof", "dog", "doom"]
const helPrefixedWords = trie.findWords("he"); // => ["hello", "helios"];
public methods:
class Trie {
public add(word: string): void; // adds the word to the Trie
public addMany(words: string[]): void; // invokes add for each string
public remove(word: string): boolean; // removes the word from the Trie, does not delete the node
public findWords(prefix: string): string[]; // Matches all words by the given prefix
public size(): number; // returns how many full words are in the Trie
public getAllWords(): string[]; // returns an array of all the words in the Trie
public contains(word: string): boolean; // returns true if the given string exists within the tree, may not be a full word
public findNode(prefix: string): TrieNode | undefined; // returns first match
}
The items in the Trie
are stored as TrieNodes
these should not need to be directly referenced
class TrieNode {
public isLeaf: boolean; // defaults to false
public readonly children: Map<string, TrieNode>; // defaults to an empty map
public constructor(public readonly char: string) {}
}
FAQs
A JavaScript implementation of a Trie
The npm package trie-structure receives a total of 1 weekly downloads. As such, trie-structure popularity was classified as not popular.
We found that trie-structure demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.4 brings back bundling, improves dependency updates and telemetry, and makes the runtime more practical for real-world JavaScript projects.
Security News
CVEForecast.org uses machine learning to project a record-breaking surge in vulnerability disclosures in 2025.
Security News
Browserslist-rs now uses static data to reduce binary size by over 1MB, improving memory use and performance for Rust-based frontend tools.