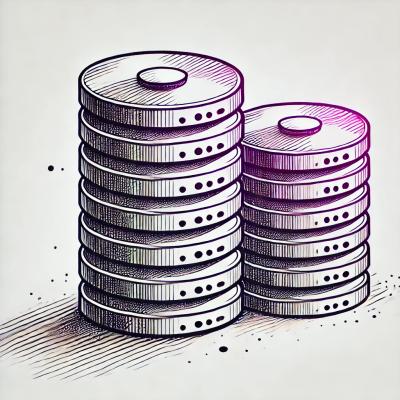
Security News
MCP Community Begins Work on Official MCP Metaregistry
The MCP community is launching an official registry to standardize AI tool discovery and let agents dynamically find and install MCP servers.
truffle-assertions
Advanced tools
Additional assertions and utilities for testing Ethereum smart contracts in Truffle unit tests
This package adds additional assertions that can be used to test Ethereum smart contracts inside Truffle tests.
truffle-assertions can be installed through npm:
npm install truffle-assertions
To use this package, import it at the top of the Truffle test file, and use the functions that are documented below.
const truffleAssert = require('truffle-assertions');
I wrote two tutorials on using this library for checking events and asserting reverts inside smart contract tests:
I also gave a two talks that explain a few different use cases of the library:
The eventEmitted
assertion checks that an event with type eventType
has been emitted by the transaction with result result
. A filter function can be passed along to further specify requirements for the event arguments:
truffleAssert.eventEmitted(result, 'TestEvent', (ev) => {
return ev.param1 === 10 && ev.param2 === ev.param3;
});
Alternatively, a filter object can be passed in place of a function. If an object is passed, this object will be matched against the event's arguments. This object does not need to include all the event's arguments; only the included ones will be used in the comparison.
truffleAssert.eventEmitted(result, 'TestEvent', { param1: 10, param2: 20 });
When the filter
parameter is omitted or set to null, the assertion checks just for event type:
truffleAssert.eventEmitted(result, 'TestEvent');
Optionally, a custom message can be passed to the assertion, which will be displayed alongside the default one:
truffleAssert.eventEmitted(result, 'TestEvent', (ev) => {
return ev.param1 === 10 && ev.param2 === ev.param3;
}, 'TestEvent should be emitted with correct parameters');
The default messages are
`Event of type ${eventType} was not emitted`
`Event filter for ${eventType} returned no results`
Depending on the reason for the assertion failure. The default message also includes a list of events that were emitted in the passed transaction.
The eventNotEmitted
assertion checks that an event with type eventType
has not been emitted by the transaction with result result
. A filter function can be passed along to further specify requirements for the event arguments:
truffleAssert.eventNotEmitted(result, 'TestEvent', (ev) => {
return ev.param1 === 10 && ev.param2 === ev.param3;
});
Alternatively, a filter object can be passed in place of a function. If an object is passed, this object will be matched against the event's arguments. This object does not need to include all the event's arguments; only the included ones will be used in the comparison.
truffleAssert.eventNotEmitted(result, 'TestEvent', { param1: 10, param2: 20 });
When the filter
parameter is omitted or set to null, the assertion checks just for event type:
truffleAssert.eventNotEmitted(result, 'TestEvent');
Optionally, a custom message can be passed to the assertion, which will be displayed alongside the default one:
truffleAssert.eventNotEmitted(result, 'TestEvent', null, 'TestEvent should not be emitted');
The default messages are
`Event of type ${eventType} was emitted`
`Event filter for ${eventType} returned results`
Depending on the reason for the assertion failure. The default message also includes a list of events that were emitted in the passed transaction.
Pretty prints the full list of events with their parameters, that were emitted in transaction with result result
truffleAssert.prettyPrintEmittedEvents(result);
Events emitted in tx 0x7da28cf2bd52016ee91f10ec711edd8aa2716aac3ed453b0def0af59991d5120:
----------------------------------------------------------------------------------------
TestEvent(testAddress = 0xe04893f0a1bdb132d66b4e7279492fcfe602f0eb, testInt: 10)
----------------------------------------------------------------------------------------
There can be times where we only have access to a transaction hash, and not to a transaction result object, such as with the deployment of a new contract instance using Contract.new();
. In these cases we still want to be able to assert that certain events are or aren't emitted.
truffle-assertions
offers the possibility to create a transaction result object from a contract instance and a transaction hash, which can then be used in the other functions that the library offers.
Note: This function assumes that web3 is injected into the tests, which truffle does automatically. If you're not using truffle, you should import web3 manually at the top of your test file.
let contractInstance = await Contract.new();
let result = await truffleAssert.createTransactionResult(contractInstance, contractInstance.transactionHash);
truffleAssert.eventEmitted(result, 'TestEvent');
Asserts that the passed async contract function does not fail.
await truffleAssert.passes(
contractInstance.methodThatShouldPass()
);
Optionally, a custom message can be passed to the assertion, which will be displayed alongside the default one:
await truffleAssert.passes(
contractInstance.methodThatShouldPass(),
'This method should not run out of gas'
);
The default message is
`Failed with ${error}`
Asserts that the passed async contract function fails with a certain ErrorType and reason.
The different error types are defined as follows:
ErrorType = {
REVERT: "revert",
INVALID_OPCODE: "invalid opcode",
OUT_OF_GAS: "out of gas",
INVALID_JUMP: "invalid JUMP"
}
await truffleAssert.fails(
contractInstance.methodThatShouldFail(),
truffleAssert.ErrorType.OUT_OF_GAS
);
A reason can be passed to the assertion, which functions as an extra filter on the revert reason (note that this is only relevant in the case of revert, not for the other ErrorTypes). This functionality requires at least Truffle v0.5.
await truffleAssert.fails(
contractInstance.methodThatShouldFail(),
truffleAssert.ErrorType.REVERT,
"only owner"
);
If the errorType parameter is omitted or set to null, the function just checks for failure, regardless of cause.
await truffleAssert.fails(contractInstance.methodThatShouldFail());
Optionally, a custom message can be passed to the assertion, which will be displayed alongside the default one:
await truffleAssert.fails(
contractInstance.methodThatShouldFail(),
truffleAssert.ErrorType.OUT_OF_GAS,
null,
'This method should run out of gas'
);
The default messages are
'Did not fail'
`Expected to fail with ${errorType}, but failed with: ${error}`
This is an alias for truffleAssert.fails(asyncFn, truffleAssert.ErrorType.REVERT[, reason][, message])
.
await truffleAssert.reverts(
contractInstance.methodThatShouldRevert(),
"only owner"
);
If you use this library inside your own projects and you would like to support its development, you can donate Ξ to 0x6775f0Ee4E63983501DBE7b0385bF84DBd36D69B
.
FAQs
Additional assertions and utilities for testing Ethereum smart contracts in Truffle unit tests
The npm package truffle-assertions receives a total of 1,023 weekly downloads. As such, truffle-assertions popularity was classified as popular.
We found that truffle-assertions demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
The MCP community is launching an official registry to standardize AI tool discovery and let agents dynamically find and install MCP servers.
Research
Security News
Socket uncovers an npm Trojan stealing crypto wallets and BullX credentials via obfuscated code and Telegram exfiltration.
Research
Security News
Malicious npm packages posing as developer tools target macOS Cursor IDE users, stealing credentials and modifying files to gain persistent backdoor access.