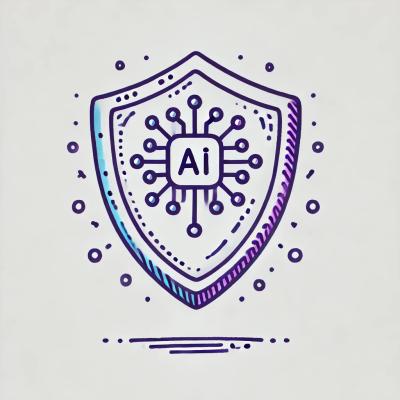
Security News
38% of CISOs Fear They’re Not Moving Fast Enough on AI
CISOs are racing to adopt AI for cybersecurity, but hurdles in budgets and governance may leave some falling behind in the fight against cyber threats.
truncate-utf8-bytes
Advanced tools
The 'truncate-utf8-bytes' npm package is designed to truncate a string to a specified number of bytes, ensuring that the resulting string is valid UTF-8. This is particularly useful when dealing with systems that have byte-length constraints for strings, such as databases or network protocols.
Truncate a string to a specified number of bytes
This feature allows you to truncate a string to a specified number of bytes. In this example, the string 'Hello, world!' is truncated to 5 bytes, resulting in 'Hello'.
const truncate = require('truncate-utf8-bytes');
const str = 'Hello, world!';
const truncatedStr = truncate(str, 5);
console.log(truncatedStr); // Output: 'Hello'
Handle multi-byte characters correctly
This feature ensures that multi-byte characters are handled correctly. In this example, the Japanese string 'こんにちは' is truncated to 6 bytes, resulting in 'こん', which is a valid UTF-8 string.
const truncate = require('truncate-utf8-bytes');
const str = 'こんにちは'; // 'Hello' in Japanese
const truncatedStr = truncate(str, 6);
console.log(truncatedStr); // Output: 'こん'
The 'utf8-byte-length' package calculates the byte length of a UTF-8 string but does not provide truncation functionality. It is useful for determining the byte length of a string before performing operations that require byte-length constraints.
The 'utf8' package provides utilities for encoding and decoding UTF-8 strings but does not include truncation functionality. It is more focused on converting between UTF-8 and other encodings.
The 'string-byte-length' package calculates the byte length of a string and can also truncate it to a specified byte length. However, it may not handle multi-byte characters as gracefully as 'truncate-utf8-bytes'.
Truncate a string to the given length in bytes. Correctly handles multi-byte characters and surrogate pairs.
A browser implementation that doesn't use Buffer.byteLength
is
provided to minimize build size.
var truncate = require("truncate-utf8-bytes")
var str = "a☃" // a = 1 byte, ☃ = 3 bytes
console.log(truncate(str, 2))
// -> "a"
var truncate = require("truncate-utf8-bytes")
When using browserify or webpack, this automatically resolves to an
implementation that does not use Buffer.byteLength
.
truncate(string, length)
Returns string
truncated to at most length
bytes in length.
FAQs
Truncate string to given length in bytes
The npm package truncate-utf8-bytes receives a total of 1,000,934 weekly downloads. As such, truncate-utf8-bytes popularity was classified as popular.
We found that truncate-utf8-bytes demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
CISOs are racing to adopt AI for cybersecurity, but hurdles in budgets and governance may leave some falling behind in the fight against cyber threats.
Research
Security News
Socket researchers uncovered a backdoored typosquat of BoltDB in the Go ecosystem, exploiting Go Module Proxy caching to persist undetected for years.
Security News
Company News
Socket is joining TC54 to help develop standards for software supply chain security, contributing to the evolution of SBOMs, CycloneDX, and Package URL specifications.