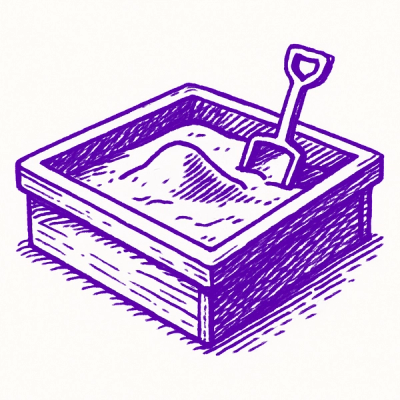
Research
/Security News
Critical Vulnerability in NestJS Devtools: Localhost RCE via Sandbox Escape
A flawed sandbox in @nestjs/devtools-integration lets attackers run code on your machine via CSRF, leading to full Remote Code Execution (RCE).
trusted-accounts-sdk-node
Advanced tools
The Trusted Accounts Node SDK allows you to integrate user verification and validation into your Node.js application with minimal setup. It supports the OIDC flow, dynamic redirects, and session management.
npm install --save trusted-accounts-sdk-node
Get your platform credentials by creating a free developer account here: developer console.
import TrustedAccountsClient from 'trusted-accounts-sdk-node';
const taClient = new TrustedAccountsClient(
"CLIENT_ID", // Your Client ID
"CLIENT_SECRET", // Your Client Secret
"REDIRECT_URL" // Your Redirect URI
);
app.get('/start-verification', async (req, res) => {
// Generate the verification URL
const verificationUrl = await taClient.generateAuthorizationUrl(
'foo@bar.com', // The email of the user you want to validate
);
// Redirect the user to the verification page
// or create a verification button to trigger it manually
res.redirect(verificationUrl);
});
app.get('/callback', async (req, res) => {
const trustedId = await taClient.handleCallback(req.query.code);
res.send('Verification completed! Trusted ID: ' + trustedId);
});
That's it! You have successfully integrated Trusted Accounts on your platform! 🖖 Try validate yourself and then go to the user list in the developer console to find the newly validated user.
FAQs
A simple SDK for Trusted Accounts for NodeJS
The npm package trusted-accounts-sdk-node receives a total of 8 weekly downloads. As such, trusted-accounts-sdk-node popularity was classified as not popular.
We found that trusted-accounts-sdk-node demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
/Security News
A flawed sandbox in @nestjs/devtools-integration lets attackers run code on your machine via CSRF, leading to full Remote Code Execution (RCE).
Product
Customize license detection with Socket’s new license overlays: gain control, reduce noise, and handle edge cases with precision.
Product
Socket now supports Rust and Cargo, offering package search for all users and experimental SBOM generation for enterprise projects.