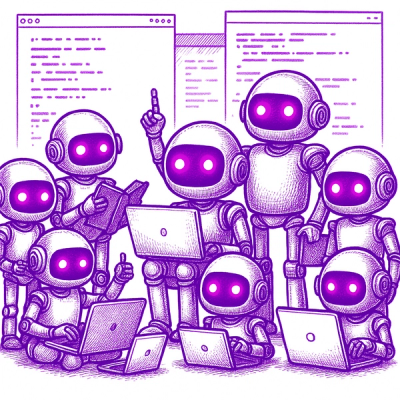
Security News
Open Source CAI Framework Handles Pen Testing Tasks up to 3,600× Faster Than Humans
CAI is a new open source AI framework that automates penetration testing tasks like scanning and exploitation up to 3,600× faster than humans.
ts-messenger-api
Advanced tools
Unofficial API for Facebook Messenger. This package provides programmatic access to Facebook Messenger activity like sending and receiving messages on behalf of actual user.
⚠ Note: This package works only on NodeJS v15 and higher!
Since Facebook makes frequent modification to their API, please bear in mind, that this library is prone to developing bugs, that may not be immediately fixed.
You can install the package with NPM:
npm i ts-messenger-api
import facebookLogin from 'ts-messenger-api';
// for plain JavaScript use this:
// const facebookLogin = require('ts-messenger-api').default;
const api = await facebookLogin({
email: 'your.email@example.com',
password: 'your_messenger_password'
});
const friends = await api.getFriendsList();
await api.listen();
await api.sendMessage({ body: 'Hi' }, friends[0].id);
There are some examples of sending messages below. First of all, start with await api.listen();
to establish a websocket connection.
api.sendMessage({ body: 'This' }, friends[3].id);
Facebook automatically recognises file type (picture, video, audio or general file).
import fs from 'fs';
api.sendMessage({ attachment: fs.createReadStream('path-to-file') }, friends[3].id);
// or send multiple attachments in one message
api.sendMessage(
{
attachment: [
fs.createReadStream('path-to-file1'),
fs.createReadStream('path-to-file2'),
fs.createReadStream('path-to-file3')
]
},
friends[3].id
);
api.sendMessage(
{
body: 'This is my reply to your question',
replyToMessage: originalMessageId
},
friends[3].id
);
Similarly, you can send a message with mentions. The types for that are in the docs.
First of all, start with await api.listen()
to establish a websocket connection.
You can access the EventEmitter
by using the returned value of this function
or get it directly from api.listener
property.
Posible event types are:
"message"
for all incoming messages and events (for listening to events,
specify the options
argument as {listenEvents: true}
while login)"presence"
for information about friends' active state"typ"
for incoming typing indicators"error"
for possible errors caused by websocket communicationapi.listener.addEventListener('message', (msg: AnyIncomingMessage) => console.log(msg));
api.listener.addEventListener('error', err => console.error(err));
Type of an incoming message is defined in
msg.type
.
AppState
You can login to Facebook account for the second time without the need to provide login
credentials (email and password). This feature is provided with AppState
(array of
cookies provided by Facebook). We advise you to save the AppState right after first
successful login using:
// after first login
fs.writeFileSync('./appState.json', JSON.stringify(api.getAppState()));
Now you can use the saved cookies to log in later.
// using the saved AppState
let api: Api | null = null;
try {
api = (await login(
{
appState: JSON.parse(fs.readFileSync('./appState.json').toString())
},
{ listenEvents: true }
)) as Api;
} catch (error) {
// something like `console.error(error);`
}
You can find the documentation here.
The following table lists all features that are implemented or are destined to be implemented in the future. If you would like to have some feature implemented do not hesitate to submit PR.
Feature | Implemented |
---|---|
Login | ✔ |
Logout | ✔ |
Get app state | ✔ |
Messages | |
Send message | ✔ |
Unsend message | ✔ |
Delete message | ❌ |
Forward attachment | ✔ |
Set message reaction | ✔ |
Send typing indicator | ✔ |
Users | |
Get friends list | ✔ |
Get user ID | ❌ |
Get user info | ✔ |
Threads | |
Get thread list | ❌ |
Get thread info | ✔ |
Get thread history | ✔ |
Get thread pictures | ❌ |
Delete thread | ❌ |
Mute thread | ❌ |
Search for thread | ❌ |
Customisation | |
Change thread colour | ✔ |
Change thread emoji | ✔ |
Group management | |
Add user to group | ✔ |
Remove user from group | ✔ |
Leave group | ✔ |
Change admin status | ✔ |
Change group image | ✔ |
Change group title | ✔ |
Change nickname | ❌ |
Create poll | ❌ |
Mark as read | ✔ |
Mark as read all | ❌ |
Change archived status | ❌ |
Change blocked status | ❌ |
FAQs
The unofficial API for FB Messenger communication
The npm package ts-messenger-api receives a total of 14 weekly downloads. As such, ts-messenger-api popularity was classified as not popular.
We found that ts-messenger-api demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
CAI is a new open source AI framework that automates penetration testing tasks like scanning and exploitation up to 3,600× faster than humans.
Security News
Deno 2.4 brings back bundling, improves dependency updates and telemetry, and makes the runtime more practical for real-world JavaScript projects.
Security News
CVEForecast.org uses machine learning to project a record-breaking surge in vulnerability disclosures in 2025.