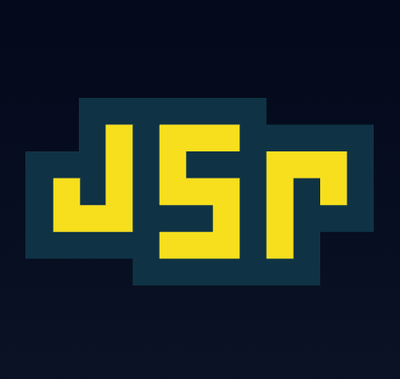
Security News
JSR Working Group Kicks Off with Ambitious Roadmap and Plans for Open Governance
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
Browserify plugin for compiling TypeScript
var browserify = require('browserify');
var tsify = require('tsify');
browserify()
.add('main.ts')
.plugin(tsify, { noImplicitAny: true })
.bundle()
.on('error', function (error) { console.error(error.toString()); })
.pipe(process.stdout);
$ browserify main.ts -p [ tsify --noImplicitAny ] > bundle.js
Note that when using the Browserify CLI, compilation will always halt on the first error encountered, unlike the regular TypeScript CLI. This behavior can be overridden in the API, as shown in the API example.
Also note that the square brackets [ ]
in the example above are required if you want to pass parameters to tsify; they don't denote an optional part of the command.
Just plain ol' npm installation:
npm install browserify
npm install typescript
npm install tsify
For use on the command line, use the flag npm install -g
.
--debug
option is set on Browserify, regardless of the flag status in tsconfig.json
.-d, --declaration
- See tsify#15--out, --outDir
- Use Browserify's file output options instead. These options are overridden because tsify writes to an internal memory store before bundling, instead of to the filesystem.-p, --project
option which allows you to specify the path that will be used when searching for the tsconfig.json
file. You can pass either the path to a directory or to the tsconfig.json
file itself. (When using the API, the project
option can specify either a path to a directory or file, or the JSON content of a tsconfig.json
file.)files
, exclude
and include
options. In particular, if "files": []
is specified, only the Browserify entry points (and their dependencies) are passed to TypeScript for compilation.--global
- This will set up tsify as a global transform. See the Browserify docs for the implications of this flag.--typescript
- By default we just do require('typescript')
to pickup whichever version you installed. However, this option allows you to pass in a different TypeScript compiler, such as NTypeScript. Note that when using the API, you can pass either the name of the alternative compiler or a reference to it:
{ typescript: 'ntypescript' }
{ typescript: require('ntypescript') }
tsify will automatically read options from tsconfig.json
. However, some options from this file will be ignored:
compilerOptions.declaration
- See tsify#15compilerOptions.out
, compilerOptions.outDir
, and compilerOptions.noEmit
- Use Browserify's file output options instead. These options are overridden because tsify writes its intermediate JavaScript output to an internal memory store instead of to the filesystem.files
- Use Browserify's file input options instead. This is necessary because Browserify needs to know which file(s) are the entry points to your program.compilerOptions.sourceMaps
- Source maps are only generated if the --debug
option is set on Browserify.compilerOptions.inlineSourceMaps
- Generated source maps are always inline.Yes! tsify can do incremental compilation using watchify, resulting in much faster incremental build times. Just follow the Watchify documentation, and add tsify as a plugin as indicated in the documentation above.
No problem. See the Gulp recipes on using browserify and watchify, and add tsify as a plugin as indicated in the documentation above.
Use grunt-browserify and you should be good! Just add tsify as a plugin in your Grunt configuration.
The inlined sourcemaps that Browserify generates may not be readable by IE 11 for debugging purposes. This is easy to fix by adding exorcist to your build workflow after Browserify.
TypeScript's ES2015 output mode should work without too much additional setup. Browserify does not support ES2015 modules, so if you want to use ES2015 you still need some transpilation step. Make sure to add babelify to your list of transforms. Note that if you are using the API, you need to set up tsify before babelify:
browserify()
.plugin(tsify, { target: 'es6' })
.transform(babelify, { extensions: [ '.tsx', '.ts' ] })
This error occurs when a TypeScript file is not compiled to JavaScript before being run through the Browserify bundler. There are a couple known reasons you might run into this.
.plugin('tsify')
is done before any transforms such as .transform('babelify')
. tsify needs to run first!expose
set to the name of the included file. More details and a workaround are available in #60.There are several TypeScript compilation transforms available on npm, all with various issues. The TypeScript compiler automatically performs dependency resolution on module imports, much like Browserify itself. Browserify transforms are not flexible enough to deal with multiple file outputs given a single file input, which means that any working TypeScript compilation transform either skips the resolution step (which is necessary for complete type checking) or performs multiple compilations of source files further down the dependency graph.
tsify avoids this problem by using the power of plugins to perform a single compilation of the TypeScript source up-front, using Browserify to glue together the resulting files.
MIT
d.ts
file.@types/browserify
and incorrect/undocumented use of TypeScript types in tsify
signature.index.d.ts
and add @types/browserify
dependency.types
to package.json
.watchify
does not stop listening.forceConsistentCasingInFilenames
compiler option.files
, exclude
and include
options.forceConsistentCasingInFilenames
compiler option.allowJs
no longer causes problems with streams.project
option using the JSON content of a tsconfig.json
file.include
option was broken if tsconfig.json
was not in the current directory.tsconfig
, so filesGlob
is no longer supported. Use TypeScript 2's exclude
and include
options instead.@types
modules.Object.assign
with object-assign
for Node 0.12 compatibility.lib
option) were left out of the compilation when bundling on Windows.tsify
as a transform now results in an explicit error.target
being set to ES3
).-p
).basedir
contains backslashes that need normalization for findConfigFile to work correctly.devDependency
so we don't install one for you. Please run npm install typescript --save-dev
in your project to use whatever version you want.TypeStrong/tsconfig
for parsing tsconfig.json
to add support for exclude
and more.--global
flag to use tsify as a global transform.files
property of tsconfig.json
.--project
flag to use a custom location for tsconfig.json
.debuglog
dependency.debuglog
dependency for compatibility with old versions of Node 0.12.--debug
is set, for potential speed improvements when not using sourcemaps.--jsx=preserve
is set.lodash
and debuglog
dependencies.basedir
option.allowJs
option to enable transpiling ES6+ JS to ES5 or lower.findConfigFile
for TypeScript 1.9 dev.findConfigFile
for use with the TypeScript 1.8 dev version.*.tsx
was not included in Browserify's list of extensions if the jsx
option was set via tsconfig.json
.process.cwd()
(thanks @pnlybubbles!)typescript
dependency to lock it down to version 1.6.xtypescript
dependency to lock it down to version 1.5.x*.tsx
to Browserify's list of extensions if --jsx
is set (with priority *.ts > *.tsx > *.js).--inlineSourceMap
and --inlineSources
(because that's what Browserify expects).lib.d.ts
to support TypeScript 1.6 and to work with the --typescript
option.preserve
.*.tsx
to the regex determining whether to run a file through the TypeScript compiler.file
event to trigger watchify even when there are fatal compilation errors.--typescript
option.--out
and --outDir
compiler options.tsconfig.json
support.stopOnError
option and changed default behavior to continue building when there are typing errors.bundler.add()
.watchify
.FAQs
Browserify plugin for compiling Typescript
The npm package tsify receives a total of 28,536 weekly downloads. As such, tsify popularity was classified as popular.
We found that tsify demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 3 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
Security News
Research
An advanced npm supply chain attack is leveraging Ethereum smart contracts for decentralized, persistent malware control, evading traditional defenses.
Security News
Research
Attackers are impersonating Sindre Sorhus on npm with a fake 'chalk-node' package containing a malicious backdoor to compromise developers' projects.