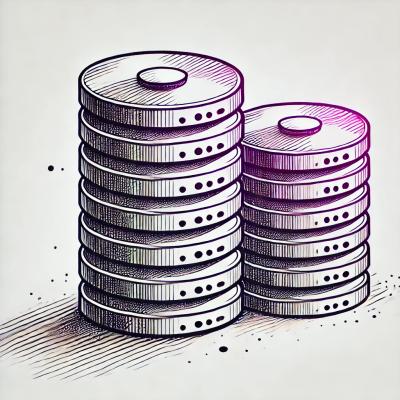
Security News
MCP Community Begins Work on Official MCP Metaregistry
The MCP community is launching an official registry to standardize AI tool discovery and let agents dynamically find and install MCP servers.
typed-structures
Advanced tools
Typed Structures is an MIT-licensed on-going open source project meant to bring a fullset of data structures to the awesome TypeScript 🎉
With the rise in popularity of Typescript, we think that having the same kind of data structures as the standard libraries of widely used strongly typed languages would be helpful to anyone planning to implement complex features. We believe in the power of open-source and we want this project to be driven by the ones who need it.
npm install typed-structures
import { Map, Buffer, Set /* ... */ } from 'typed-structures';
Complete API available here.
Generic doubly linked list.
import { DoublyLinkedList, Node, Queue } from 'typed-structures';
import { Track } from './track';
import { MixService } from './mix-service';
class Player {
private playlist: DoublyLinkedList<Track> = new DoublyLinkedList<Track>();
private mix: Queue<Track> = MixService.getMix();
private currentTrack: Node<Track>;
constructor() {
this.playlist.push(this.mix.dequeue());
this.currentTrack = new Node<Track>(this.playlist.peek());
}
onNextClick() {
this.next();
}
onPreviousClick() {
this.previous();
}
onTrackEnd() {
this.next();
}
private next() {
this.playlist.push(this.mix.dequeue());
this.currentTrack = this.currentTrack.next();
}
private previous() {
this.currentTrack = this.currentTrack.previous();
}
}
Generic plain data map.
import { Map, Stack } from 'typed-structures';
import { Task } from "./task";
let map: Map<string, Stack<Task>> = new Map<string, Stack<Task>>();
map.put('todo', new Stack<Task>());
map.put('in-progress', new Stack<Task>());
map.put('done', new Stack<Task>());
let firstTask: Task = new Task('Read the docs');
let todo: Stack<Task> = map.get('todo');
todo.stack(firstTask);
map.replace('todo', todo);
let inProgress: Stack<Task> = map.get('in-progress');
inProgress.stack(map.get('todo').unstack());
map.replace('in-progress', inProgress);
let done: Stack<Task> = map.get('done');
done.stack(map.get('in-progress').unstack());
map.replace('done', done);
Generic set.
import { Set } from 'typed-structures';
let set: Set<string> = new Set<string>();
set.add('first');
set.add('second');
console.log(set.contains('first')); // true
for (let key of set.keys())
console.log(key); // first, second
Simple and generic stack.
import { Stack } from "typed-structures";
import { Task } from "./task";
let taskStack: Stack<Task> = new Stack<Task>();
taskStack.stack(new Task('taskName'));
console.log(taskStack.peek().name); // taskName
console.log(taskStack.empty()); // false
console.log(taskStack.length()); // 1
console.log(taskStack.unstack().name); //taskName
console.log(taskStack.empty()); // true
import { Queue } from 'typed-structures';
import { Emiter } from './emiter';
import { Logger } from './logger';
let emiter1: Emiter = new Emiter();
let emiter2: Emiter = new Emiter();
let logger: Logger = new Logger();
let channel: Queue<string> = new Queue<string>();
channel.enqueue(emiter1.emit('Hi !'));
channel.enqueue((emiter2.emit('Hey, How are you doing ?')));
/* ... */
while (!channel.empty()) {
logger.log(channel.dequeue());
}
import { BinaryTreeSearch } from 'typed-structures';
let tree: BinaryTreeSearch<string> = new BinaryTreeSearch<string>();
tree.add('Find me ^^');
console.log(tree.find(tree.root(), 'Find me ^^')); // Find me ^^
console.log(tree.find(tree.root(), '404')); // undefined
Generic buffer.
import { GenericBuffer } from 'typed-structures';
let b: GenericBuffer<number> = new GenericBuffer<number>(4, 3, 0, -1);
b.put(1);
b.put(2);
b.rewind();
console.log(b.get()); // 1
console.log(b.get()); // 2
Generic ring buffer.
import { GenericRingBuffer } from 'typed-structures';
let b: GenericRingBuffer<number> = new GenericRingBuffer<number>(4, 3, 0, 0, 0);
b.put(1);
b.put(2);
console.log(b.get()); // 1
Minimalist implementation of .NET's LinQ for TypedStructures.
import { TsQ, Set } from 'typed-structures';
import { Person } from './person';
let firstUnknown: Person = new Person(1, 'John', 'Doe', new Date('1970-01-01'));
let secondUnknown: Person = new Person(2, 'Jane', 'Doe', new Date('1970-01-02'));
let thirdUnknown: Person = new Person(3, 'Complete', 'Stranger', new Date('1970-01-03'));
let fourthUnknown: Person = new Person(4, 'Unidentified', 'Person', new Date('1970-01-04'));
let fifthUnknown: Person = new Person(5, 'Unnamed', 'Person', new Date('1970-01-04'));
let unknowns: Set<Person> = new Set<Person>();
unknowns.add(firstUnknown);
unknowns.add(secondUnknown);
unknowns.add(thirdUnknown);
unknowns.add(fourthUnknown);
unknowns.add(fifthUnknown);
TsQ.from(unknowns)
.select("name")
.where(e => e.name.length > 4)
.order_by("id", "desc")
.fetch();
TsQ.from(unknowns)
.group_by("group")
.fetch();
For questions and support feel free to open an issue
See CONTRIBUTING.md
Feel free to contribute and enhance typed structures 🎉💛
2018-present Anthony & Thibaud
FAQs
Common typed structures implementations in TypeScript
The npm package typed-structures receives a total of 33 weekly downloads. As such, typed-structures popularity was classified as not popular.
We found that typed-structures demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
The MCP community is launching an official registry to standardize AI tool discovery and let agents dynamically find and install MCP servers.
Research
Security News
Socket uncovers an npm Trojan stealing crypto wallets and BullX credentials via obfuscated code and Telegram exfiltration.
Research
Security News
Malicious npm packages posing as developer tools target macOS Cursor IDE users, stealing credentials and modifying files to gain persistent backdoor access.