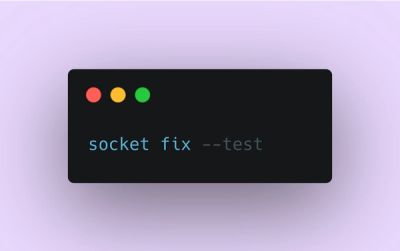
Product
Introducing Socket Fix for Safe, Automated Dependency Upgrades
Automatically fix and test dependency updates with socket fixβa new CLI tool that turns CVE alerts into safe, automated upgrades.
Help in opening URLs (mostly HTTP) in a complex world β basic and digest authentication, redirections, timeout and more. Base undici API.
The urllib npm package is a utility library for making HTTP requests and handling URLs. It provides a simple and flexible API for performing various types of HTTP requests, handling query parameters, and working with URLs.
HTTP GET Request
This feature allows you to make a simple HTTP GET request to a specified URL. The callback function handles the response data or any errors that occur.
const urllib = require('urllib');
urllib.request('https://api.example.com/data', function (err, data, res) {
if (err) {
console.error(err);
return;
}
console.log(data.toString());
});
HTTP POST Request
This feature allows you to make an HTTP POST request with a payload. The data object contains the key-value pairs to be sent in the request body.
const urllib = require('urllib');
urllib.request('https://api.example.com/data', {
method: 'POST',
data: {
key1: 'value1',
key2: 'value2'
}
}, function (err, data, res) {
if (err) {
console.error(err);
return;
}
console.log(data.toString());
});
Handling Query Parameters
This feature allows you to include query parameters in your HTTP request. The data object is automatically serialized into a query string and appended to the URL.
const urllib = require('urllib');
const params = {
key1: 'value1',
key2: 'value2'
};
urllib.request('https://api.example.com/data', {
data: params
}, function (err, data, res) {
if (err) {
console.error(err);
return;
}
console.log(data.toString());
});
Custom Headers
This feature allows you to set custom headers for your HTTP request. The headers object contains key-value pairs representing the header names and values.
const urllib = require('urllib');
urllib.request('https://api.example.com/data', {
headers: {
'Authorization': 'Bearer token',
'Content-Type': 'application/json'
}
}, function (err, data, res) {
if (err) {
console.error(err);
return;
}
console.log(data.toString());
});
Axios is a promise-based HTTP client for the browser and Node.js. It provides a more modern and flexible API compared to urllib, with support for interceptors, request cancellation, and automatic JSON data transformation.
Node-fetch is a lightweight module that brings the Fetch API to Node.js. It is a minimalistic alternative to urllib, focusing on simplicity and compliance with the Fetch standard.
Request is a simplified HTTP client for Node.js with a rich set of features. It is more feature-rich than urllib, offering support for OAuth, cookies, and multipart form data. However, it has been deprecated in favor of more modern alternatives like axios.
Request HTTP URLs in a complex world β basic and digest authentication, redirections, timeout and more.
npm install urllib
import { request } from 'urllib';
const { data, res } = await request('http://cnodejs.org/');
// result: { data: Buffer, res: Response }
console.log('status: %s, body size: %d, headers: %j', res.status, data.length, res.headers);
const { request } = require('urllib');
const { data, res } = await request('http://cnodejs.org/');
// result: { data: Buffer, res: Response }
console.log('status: %s, body size: %d, headers: %j', res.status, data.length, res.headers);
async request(url[, options])
GET
. Could be GET
, POST
, DELETE
or PUT
. Alias 'type'.data
will be ignored.data
and content
will be ignored.callback
will be called with data
set null
after finished writing.multipart/form-data
format, base on formstream
. If method
not set, will use POST
method by default.json
(Notes: not use application/json
here). If it's json
, will auto set Content-Type: application/json
header.text
or json
. If it's text
, the callback
ed data
would be a String. If it's json
, the data
of callback would be a parsed JSON Object and will auto set Accept: application/json
header. Default callback
ed data
would be a Buffer
.false
.5000
. You can use timeout: 5000
to tell urllib use same timeout on two phase or set them seperately such as timeout: [3000, 5000]
, which will set connecting timeout to 3s and response 5s.number | null
- Default is 4000
, 4 seconds - The timeout after which a socket without active requests will time out. Monitors time between activity on a connected socket. This value may be overridden by keep-alive hints from the server. See MDN: HTTP - Headers - Keep-Alive directives for more details.username:password
used in HTTP Basic Authorization.username:password
used in HTTP Digest Authorization.url.resolve(from, to)
.res
object when request connected, default false
. alias customResponse
gzip, br
response content and auto decode it, default is false
.true
.null
.67108864
, 64 KiB.options.data
When making a request:
await request('https://example.com', {
method: 'GET',
data: {
'a': 'hello',
'b': 'world',
},
});
For GET
request, data
will be stringify to query string, e.g. http://example.com/?a=hello&b=world
.
For others like POST
, PATCH
or PUT
request,
in defaults, the data
will be stringify into application/x-www-form-urlencoded
format
if content-type
header is not set.
If content-type
is application/json
, the data
will be JSON.stringify
to JSON data format.
options.content
options.content
is useful when you wish to construct the request body by yourself,
for example making a content-type: application/json
request.
Notes that if you want to send a JSON body, you should stringify it yourself:
await request('https://example.com', {
method: 'POST',
headers: {
'Content-Type': 'application/json',
},
content: JSON.stringify({
a: 'hello',
b: 'world',
}),
});
It would make a HTTP request like:
POST / HTTP/1.1
host: example.com
content-type: application/json
{
"a": "hello",
"b": "world"
}
This exmaple can use options.data
with application/json
content type:
await request('https://example.com', {
method: 'POST',
headers: {
'content-type': 'application/json'
},
data: {
a: 'hello',
b: 'world',
}
});
options.files
Upload a file with a hello
field.
await request('https://example.com/upload', {
method: 'POST',
files: __filename,
data: {
hello: 'hello urllib',
},
});
Upload multi files with a hello
field.
await request('https://example.com/upload', {
method: 'POST',
files: [
__filename,
fs.createReadStream(__filename),
Buffer.from('mock file content'),
],
data: {
hello: 'hello urllib with multi files',
},
});
Custom file field name with uploadfile
.
await request('https://example.com/upload', {
method: 'POST',
files: {
uploadfile: __filename,
},
});
Response is normal object, it contains:
status
or statusCode
: response status code.
-1
meaning some network error like ENOTFOUND
-2
meaning ConnectionTimeoutErrorheaders
: response http headers, default is {}
size
: response sizeaborted
: response was aborted or notrt
: total request and response time in ms.timing
: timing object if timing enable.socket
: socket infoNODE_DEBUG=urllib:* npm test
Create a HttpClient with options.allowH2 = true
import { HttpClient } from 'urllib';
const httpClient = new HttpClient({
allowH2: true,
});
const response = await httpClient.request('https://node.js.org');
console.log(response.status);
console.log(response.headers);
export from undici
import { strict as assert } from 'assert';
import { MockAgent, setGlobalDispatcher, request } from 'urllib';
const mockAgent = new MockAgent();
setGlobalDispatcher(mockAgent);
const mockPool = mockAgent.get('http://localhost:7001');
mockPool.intercept({
path: '/foo',
method: 'POST',
}).reply(400, {
message: 'mock 400 bad request',
});
const response = await request('http://localhost:7001/foo', {
method: 'POST',
dataType: 'json',
});
assert.equal(response.status, 400);
assert.deepEqual(response.data, { message: 'mock 400 bad request' });
export from undici
import { ProxyAgent, request } from 'urllib';
const proxyAgent = new ProxyAgent('http://my.proxy.com:8080');
const response = await request('https://www.npmjs.com/package/urllib', {
dispatcher: proxyAgent,
});
console.log(response.status, response.headers);
undici@6.19.2
Node.js v18.20.3
βββββββββββ¬ββββββββββββββββββββββββ¬ββββββββββ¬βββββββββββββββββββββ¬ββββββββββββββ¬ββββββββββββββββββββββββββ
β (index) β Tests β Samples β Result β Tolerance β Difference with slowest β
βββββββββββΌββββββββββββββββββββββββΌββββββββββΌβββββββββββββββββββββΌββββββββββββββΌββββββββββββββββββββββββββ€
β 0 β 'urllib2 - request' β 10 β '321.53 req/sec' β 'Β± 0.38 %' β '-' β
β 1 β 'http - no keepalive' β 10 β '607.77 req/sec' β 'Β± 0.80 %' β '+ 89.02 %' β
β 2 β 'got' β 101 β '7929.51 req/sec' β 'Β± 4.46 %' β '+ 2366.15 %' β
β 3 β 'node-fetch' β 40 β '8651.95 req/sec' β 'Β± 2.99 %' β '+ 2590.84 %' β
β 4 β 'request' β 101 β '8864.09 req/sec' β 'Β± 7.81 %' β '+ 2656.82 %' β
β 5 β 'undici - fetch' β 101 β '9607.01 req/sec' β 'Β± 4.23 %' β '+ 2887.87 %' β
β 6 β 'axios' β 55 β '10378.80 req/sec' β 'Β± 2.94 %' β '+ 3127.91 %' β
β 7 β 'superagent' β 75 β '11286.74 req/sec' β 'Β± 2.90 %' β '+ 3410.29 %' β
β 8 β 'http - keepalive' β 60 β '11288.96 req/sec' β 'Β± 2.95 %' β '+ 3410.98 %' β
β 9 β 'urllib4 - request' β 101 β '11352.65 req/sec' β 'Β± 10.20 %' β '+ 3430.79 %' β
β 10 β 'urllib3 - request' β 40 β '13831.19 req/sec' β 'Β± 2.89 %' β '+ 4201.64 %' β
β 11 β 'undici - pipeline' β 60 β '14562.44 req/sec' β 'Β± 2.91 %' β '+ 4429.06 %' β
β 12 β 'undici - request' β 70 β '19630.64 req/sec' β 'Β± 2.87 %' β '+ 6005.32 %' β
β 13 β 'undici - stream' β 55 β '20843.50 req/sec' β 'Β± 2.90 %' β '+ 6382.54 %' β
β 14 β 'undici - dispatch' β 55 β '21233.10 req/sec' β 'Β± 2.82 %' β '+ 6503.70 %' β
βββββββββββ΄ββββββββββββββββββββββββ΄ββββββββββ΄βββββββββββββββββββββ΄ββββββββββββββ΄ββββββββββββββββββββββββββ
Node.js v20.15.0
βββββββββββ¬ββββββββββββββββββββββββ¬ββββββββββ¬βββββββββββββββββββββ¬βββββββββββββ¬ββββββββββββββββββββββββββ
β (index) β Tests β Samples β Result β Tolerance β Difference with slowest β
βββββββββββΌββββββββββββββββββββββββΌββββββββββΌβββββββββββββββββββββΌβββββββββββββΌββββββββββββββββββββββββββ€
β 0 β 'urllib2 - request' β 10 β '332.91 req/sec' β 'Β± 1.13 %' β '-' β
β 1 β 'http - no keepalive' β 10 β '615.50 req/sec' β 'Β± 2.25 %' β '+ 84.88 %' β
β 2 β 'got' β 55 β '7658.39 req/sec' β 'Β± 2.98 %' β '+ 2200.42 %' β
β 3 β 'node-fetch' β 30 β '7832.96 req/sec' β 'Β± 2.96 %' β '+ 2252.86 %' β
β 4 β 'axios' β 40 β '8607.27 req/sec' β 'Β± 2.79 %' β '+ 2485.44 %' β
β 5 β 'request' β 35 β '8703.49 req/sec' β 'Β± 2.84 %' β '+ 2514.35 %' β
β 6 β 'undici - fetch' β 65 β '9971.24 req/sec' β 'Β± 2.96 %' β '+ 2895.15 %' β
β 7 β 'superagent' β 30 β '11006.46 req/sec' β 'Β± 2.90 %' β '+ 3206.11 %' β
β 8 β 'http - keepalive' β 55 β '11610.14 req/sec' β 'Β± 2.87 %' β '+ 3387.44 %' β
β 9 β 'urllib3 - request' β 25 β '13873.38 req/sec' β 'Β± 2.96 %' β '+ 4067.27 %' β
β 10 β 'urllib4 - request' β 25 β '14291.36 req/sec' β 'Β± 2.92 %' β '+ 4192.82 %' β
β 11 β 'undici - pipeline' β 45 β '14617.69 req/sec' β 'Β± 2.84 %' β '+ 4290.85 %' β
β 12 β 'undici - dispatch' β 101 β '18716.29 req/sec' β 'Β± 3.97 %' β '+ 5521.98 %' β
β 13 β 'undici - request' β 101 β '19165.16 req/sec' β 'Β± 3.25 %' β '+ 5656.81 %' β
β 14 β 'undici - stream' β 30 β '21816.28 req/sec' β 'Β± 2.99 %' β '+ 6453.15 %' β
βββββββββββ΄ββββββββββββββββββββββββ΄ββββββββββ΄βββββββββββββββββββββ΄βββββββββββββ΄ββββββββββββββββββββββββββ
Node.js v22.3.0
βββββββββββ¬ββββββββββββββββββββββββ¬ββββββββββ¬βββββββββββββββββββββ¬βββββββββββββ¬ββββββββββββββββββββββββββ
β (index) β Tests β Samples β Result β Tolerance β Difference with slowest β
βββββββββββΌββββββββββββββββββββββββΌββββββββββΌβββββββββββββββββββββΌβββββββββββββΌββββββββββββββββββββββββββ€
β 0 β 'urllib2 - request' β 15 β '297.46 req/sec' β 'Β± 2.65 %' β '-' β
β 1 β 'http - no keepalive' β 10 β '598.25 req/sec' β 'Β± 1.94 %' β '+ 101.12 %' β
β 2 β 'axios' β 30 β '8487.94 req/sec' β 'Β± 2.91 %' β '+ 2753.52 %' β
β 3 β 'got' β 50 β '10054.46 req/sec' β 'Β± 2.89 %' β '+ 3280.16 %' β
β 4 β 'request' β 45 β '10306.02 req/sec' β 'Β± 2.87 %' β '+ 3364.73 %' β
β 5 β 'node-fetch' β 55 β '11160.02 req/sec' β 'Β± 2.87 %' β '+ 3651.83 %' β
β 6 β 'superagent' β 80 β '11302.28 req/sec' β 'Β± 2.85 %' β '+ 3699.66 %' β
β 7 β 'undici - fetch' β 60 β '11357.87 req/sec' β 'Β± 2.89 %' β '+ 3718.35 %' β
β 8 β 'http - keepalive' β 60 β '13782.10 req/sec' β 'Β± 2.97 %' β '+ 4533.34 %' β
β 9 β 'urllib4 - request' β 70 β '15965.62 req/sec' β 'Β± 2.88 %' β '+ 5267.40 %' β
β 10 β 'urllib3 - request' β 55 β '16010.37 req/sec' β 'Β± 2.90 %' β '+ 5282.45 %' β
β 11 β 'undici - pipeline' β 35 β '17969.37 req/sec' β 'Β± 2.95 %' β '+ 5941.03 %' β
β 12 β 'undici - dispatch' β 101 β '18765.50 req/sec' β 'Β± 3.01 %' β '+ 6208.68 %' β
β 13 β 'undici - request' β 85 β '20091.12 req/sec' β 'Β± 2.95 %' β '+ 6654.33 %' β
β 14 β 'undici - stream' β 45 β '21599.12 req/sec' β 'Β± 2.81 %' β '+ 7161.30 %' β
βββββββββββ΄ββββββββββββββββββββββββ΄ββββββββββ΄βββββββββββββββββββββ΄βββββββββββββ΄ββββββββββββββββββββββββββ
Made with contributors-img.
FAQs
Help in opening URLs (mostly HTTP) in a complex world β basic and digest authentication, redirections, timeout and more. Base undici API.
The npm package urllib receives a total of 188,549 weekly downloads. As such, urllib popularity was classified as popular.
We found that urllib demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago.Β It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Automatically fix and test dependency updates with socket fixβa new CLI tool that turns CVE alerts into safe, automated upgrades.
Security News
CISA denies CVE funding issues amid backlash over a new CVE foundation formed by board members, raising concerns about transparency and program governance.
Product
Weβre excited to announce a powerful new capability in Socket: historical data and enhanced analytics.