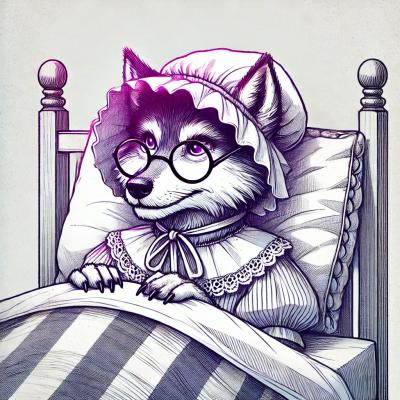
Security News
GitHub Removes Malicious Pull Requests Targeting Open Source Repositories
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
use-form-input
Advanced tools
Simple hook to provide form validation in react
Install:
npm i use-form-input
Form handling can be done with useFormInput
.
Example:
// Basic form handling
import { useFormInput } from 'use-form-input';
export default function BasicFormHandling() {
const [data, { onChange, onSubmit }] = useFormInput(
{
firstName: '',
lastName: '',
},
(data) => {
console.log('FINAL DATA', data);
}
);
return (
<>
<form onSubmit={onSubmit} style={{ marginLeft: 10 }}>
<input
type="text"
name="firstName"
value={data.firstName}
onChange={onChange}
/>
<br />
<input
type="text"
name="lastName"
value={data.lastName}
onChange={onChange}
/>
<br />
<input value="Submit" type="submit" />
</form>
</>
);
}
Note: The form element must have name
prop available to use onChange
.
Form validation can be done by passing third parameter ( function ) which should return an error object. The function is called with the data.
Example:
import { useFormInput } from 'use-form-input';
export default function FormValidation() {
const [data, { onChange, onSubmit, errors, modified }] = useFormInput(
{
firstName: '',
lastName: '',
},
(data) => {
console.log('FINAL DATA', data);
},
(data) => {
const errors = {};
if (data.firstName.length === 0) {
errors.firstName = 'Empty first name';
}
if (data.lastName.length === 0) {
errors.lastName = 'Empty last name';
}
return errors;
}
);
return (
<>
<form onSubmit={onSubmit} style={{ marginLeft: 10 }}>
<input
type="text"
name="firstName"
value={data.firstName}
onChange={onChange}
/>
{modified.firstName && errors.firstName && (
<span style={{ color: 'red' }}>{errors.firstName}</span>
)}
<br />
<input
type="text"
name="lastName"
value={data.lastName}
onChange={onChange}
/>
{modified.lastName && errors.lastName && (
<span style={{ color: 'red' }}>{errors.lastName}</span>
)}
<br />
<input value="Submit" type="submit" />
</form>
</>
);
}
Note: modified
object is used so that, the error is not shown by default. The form should either be submitted or certain fields must change before it should show error. modified
object has the property same as fields passed to useFormInput
hook.
Form can be manually validated by using validator
, setErrors
and isValid
methods.
Example:
import { useFormInput } from 'use-form-input';
export default function ManualValidation() {
const [data, { onChange, errors, setErrors, isValid, validator }] =
useFormInput({
firstName: '',
lastName: '',
});
const onSubmit = (e) => {
e.preventDefault();
const catchedErrors = {};
const validate = validator(catchedErrors);
validate('firstName', {
condition: data.firstName.length === 0,
message: 'Empty first name',
});
validate('lastName', {
condition: data.lastName.length === 0,
message: 'Emptry last name',
});
setErrors(catchedErrors);
if (!isValid(catchedErrors)) {
return;
}
console.log('FINAL DATA', data);
};
return (
<>
<form onSubmit={onSubmit} style={{ marginLeft: 10 }}>
<input
type="text"
name="firstName"
value={data.firstName}
onChange={onChange}
/>
{errors.firstName && (
<span style={{ color: 'red' }}>{errors.firstName}</span>
)}
<br />
<input
type="text"
name="lastName"
value={data.lastName}
onChange={onChange}
/>
{errors.lastName && (
<span style={{ color: 'red' }}>{errors.lastName}</span>
)}
<br />
<input value="Submit" type="submit" />
</form>
</>
);
}
We need a method to manually set the values of certain fields. We can do it by using setValue
method.
Example:
const [data, { setValue }] = useFormInput({ firstName: '' });
...
<input
type="text"
name="firstName"
value={data.firstName}
onChange={e => setValue('firstName', e.target.value)}
/>
setValue
accepts two parameters:
We can modify the previous values using setValue
method. For example, we can use it in checkbox.
Example:
const [data, { setValue }] = useFormInput({ married: false });
...
<input
type="text"
name="married"
checked={data.married}
onChange={e => setValue('married', (previousValue) => !previousValue)}
/>
It works like a setState
function, getting previous value and returning modified one.
We can clear the from by using clear
method which sets all the modified fields to its initial one.
Example:
const [data, { clear }] = useFormInput({ firstName: '', lastName: '' });
...
<button onClick={() => clear()}>Clear</button>
MIT
FAQs
Simple hook to provide form validation in react
The npm package use-form-input receives a total of 0 weekly downloads. As such, use-form-input popularity was classified as not popular.
We found that use-form-input demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.