use-local-storage
Advanced tools
Comparing version
/// <reference types="react" /> | ||
declare type Serializer<T> = (object: T | undefined) => string; | ||
declare type Parser<T> = (val: string) => T | undefined; | ||
declare type Setter<T> = React.Dispatch<React.SetStateAction<T>>; | ||
declare type Setter<T> = React.Dispatch<React.SetStateAction<T | undefined>>; | ||
declare type Options<T> = Partial<{ | ||
@@ -12,3 +12,2 @@ serializer: Serializer<T>; | ||
declare function useLocalStorage<T>(key: string, defaultValue: T, options?: Options<T>): [T, Setter<T>]; | ||
declare function useLocalStorage<T>(key: string, defaultValue?: undefined, options?: Options<T>): [T | undefined, Setter<T | undefined>]; | ||
export default useLocalStorage; |
@@ -36,4 +36,12 @@ "use strict"; | ||
return; | ||
var updateLocalStorage = function () { | ||
if (storedValue !== undefined) { | ||
window.localStorage.setItem(key, serializer(storedValue)); | ||
} | ||
else { | ||
window.localStorage.removeItem(key); | ||
} | ||
}; | ||
try { | ||
window.localStorage.setItem(key, serializer(storedValue)); | ||
updateLocalStorage(); | ||
} | ||
@@ -40,0 +48,0 @@ catch (e) { |
17
index.ts
@@ -5,3 +5,3 @@ import { useEffect, useMemo, useState } from "react"; | ||
type Parser<T> = (val: string) => T | undefined; | ||
type Setter<T> = React.Dispatch<React.SetStateAction<T>>; | ||
type Setter<T> = React.Dispatch<React.SetStateAction<T | undefined>>; | ||
@@ -22,7 +22,2 @@ type Options<T> = Partial<{ | ||
key: string, | ||
defaultValue?: undefined, | ||
options?: Options<T> | ||
): [T | undefined, Setter<T | undefined>]; | ||
function useLocalStorage<T>( | ||
key: string, | ||
defaultValue?: T, | ||
@@ -59,4 +54,12 @@ options?: Options<T> | ||
const updateLocalStorage = () => { | ||
if (storedValue !== undefined) { | ||
window.localStorage.setItem(key, serializer(storedValue)); | ||
} else { | ||
window.localStorage.removeItem(key); | ||
} | ||
} | ||
try { | ||
window.localStorage.setItem(key, serializer(storedValue)); | ||
updateLocalStorage(); | ||
} catch (e) { | ||
@@ -63,0 +66,0 @@ logger(e); |
{ | ||
"name": "use-local-storage", | ||
"version": "2.2.6", | ||
"version": "2.3.6", | ||
"description": "A flexible React Hook for using Local Storage.", | ||
@@ -5,0 +5,0 @@ "main": "dist/index.js", |
@@ -31,3 +31,3 @@ 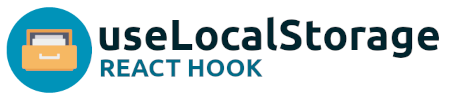 | ||
The following usage will persist the `username` variable in a `"name"` key in Local Storage. It will have a default/initial value of an empty string `""`. This default value witll _only_ be used if there is no value already in Local Storage. | ||
The following usage will persist the `username` variable in a `"name"` key in Local Storage. It will have a default/initial value of an empty string `""`. This default value will _only_ be used if there is no value already in Local Storage, moreover setting the variable `username` to `undefined` will remove it from Local Storage. | ||
@@ -48,2 +48,9 @@ ```jsx | ||
/> | ||
<button | ||
onClick={() => { | ||
setUsername(undefined); | ||
}} | ||
> | ||
Remove Username | ||
</button> | ||
</> | ||
@@ -76,2 +83,9 @@ ); | ||
/> | ||
<button | ||
onClick={() => { | ||
setUsername(undefined); | ||
}} | ||
> | ||
Remove Username | ||
</button> | ||
</> | ||
@@ -78,0 +92,0 @@ ); |
AI-detected possible typosquat
Supply chain riskAI has identified this package as a potential typosquat of a more popular package. This suggests that the package may be intentionally mimicking another package's name, description, or other metadata.
Found 1 instance in 1 package
13232
4.14%177
5.36%119
13.33%1
Infinity%