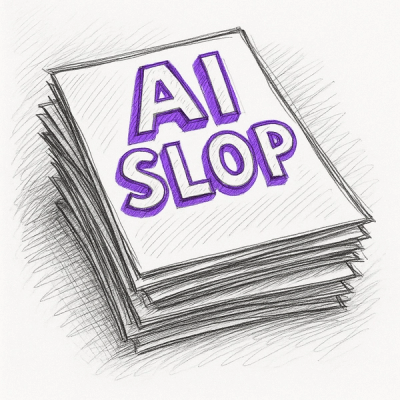
Security News
Django Joins curl in Pushing Back on AI Slop Security Reports
Django has updated its security policies to reject AI-generated vulnerability reports that include fabricated or unverifiable content.
util-methods
Advanced tools
Util methods is a versatile utility library designed to simplify your programming tasks by providing a comprehensive collection of methods for manipulating strings, arrays, objects, and more
Welcome to the String Utilities package! This library provides a set of useful JavaScript methods for string manipulation, making it easier to work with text data in your projects.
You can install the package using npm:
npm install util-methods
Import the method into your file and execute it once the DOM is fully loaded.
const { methodName } = require('util-methods');
document.addEventListener('DOMContentLoaded', function() {
utilMethods();
});
componentDidMount(){
utilMethods();
}
useEffect(()=>{
utilMethods();
},[])
mounted() {
this.utilMethods();
}
Reverses the input string.
Example:
import utilMethods from 'util-methods';
console.log(reverse('hello')); // 'olleh'
Converts a string into camelCase format
Example
import utilMethods from 'util-methods';
console.log(camelCase('hello world')); // 'helloWorld'
Checks if the input string is a palindrome (reads the same forwards and backwards).
Example
import utilMethods from 'util-methods';
console.log(isPalindrome('racecar')); // true
console.log(isPalindrome('hello')); // false
Removes all instances of a specified symbol from the input string.
import utilMethods from 'util-methods';
console.log(stripSymbol('hello@world!', '@')); // 'helloworld!'
Generates a random string of the specified length.
Example
import utilMethods from 'util-methods';
console.log(generateRandomString(10)); // 'a1B2c3D4e5' (example output)
FAQs
Util methods is a versatile utility library designed to simplify your programming tasks by providing a comprehensive collection of methods for manipulating strings, arrays, objects, and more
The npm package util-methods receives a total of 3 weekly downloads. As such, util-methods popularity was classified as not popular.
We found that util-methods demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Django has updated its security policies to reject AI-generated vulnerability reports that include fabricated or unverifiable content.
Security News
ECMAScript 2025 introduces Iterator Helpers, Set methods, JSON modules, and more in its latest spec update approved by Ecma in June 2025.
Security News
A new Node.js homepage button linking to paid support for EOL versions has sparked a heated discussion among contributors and the wider community.