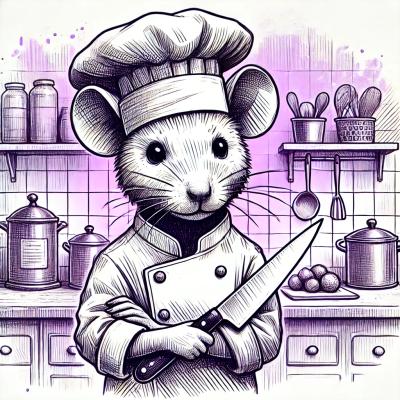
Research
Security News
Quasar RAT Disguised as an npm Package for Detecting Vulnerabilities in Ethereum Smart Contracts
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
vue-hot-reload-api
Advanced tools
The vue-hot-reload-api package is a library that provides hot-reloading capabilities for Vue.js components. It allows developers to update Vue components in real-time without losing the state of the application, which significantly speeds up the development process.
Install and Setup
This code demonstrates how to install and set up the vue-hot-reload-api. It installs the hot-reload API and sets it up to work with Vue. The module.hot.accept() call ensures that the module accepts hot updates.
const hotAPI = require('vue-hot-reload-api');
hotAPI.install(require('vue'));
if (module.hot) {
module.hot.accept();
}
Create a Hot-Reloadable Component
This code shows how to create a hot-reloadable Vue component. When the MyComponent.vue file is updated, the new component is reloaded without losing the state of the application.
if (module.hot) {
module.hot.accept('./MyComponent.vue', function () {
const NewComponent = require('./MyComponent.vue').default;
hotAPI.reload('my-component-id', NewComponent);
});
}
Preserve Component State
This code demonstrates how to preserve the state of a Vue component during hot-reloading. By passing the { preserveState: true } option, the state of the component is maintained even after the component is reloaded.
if (module.hot) {
module.hot.accept('./MyComponent.vue', function () {
const NewComponent = require('./MyComponent.vue').default;
hotAPI.reload('my-component-id', NewComponent, { preserveState: true });
});
}
react-hot-loader is a similar package for React that provides hot-reloading capabilities for React components. It allows developers to update React components in real-time without losing the state of the application. Compared to vue-hot-reload-api, react-hot-loader is specifically designed for React and integrates seamlessly with the React ecosystem.
webpack-hot-middleware is a middleware for Webpack that enables hot module replacement (HMR) for any JavaScript application. It is framework-agnostic and can be used with various front-end frameworks, including Vue.js. While vue-hot-reload-api is specifically designed for Vue components, webpack-hot-middleware provides a more general solution for hot-reloading in any JavaScript project.
Vite is a build tool that provides fast development server and hot module replacement (HMR) out of the box. It supports Vue.js, React, and other frameworks. Vite offers a more modern and efficient approach to hot-reloading compared to vue-hot-reload-api, as it leverages native ES modules and modern browser features for faster performance.
Note:
vue-hot-reload-api@2.x
only works withvue@2.x
Hot reload API for Vue components. This is what vue-loader and vueify use under the hood.
You will only be using this if you are writing some build toolchain based on Vue components. For normal application usage, just use vue-loader
or vueify
.
// define a component as an options object
const myComponentOptions = {
data () { ... },
created () { ... },
render () { ... }
}
// assuming Webpack's HMR API.
// https://webpack.js.org/guides/hot-module-replacement/
if (module.hot) {
const api = require('vue-hot-reload-api')
const Vue = require('vue')
// make the API aware of the Vue that you are using.
// also checks compatibility.
api.install(Vue)
// compatibility can be checked via api.compatible after installation
if (!api.compatible) {
throw new Error('vue-hot-reload-api is not compatible with the version of Vue you are using.')
}
// indicate this module can be hot-reloaded
module.hot.accept()
if (!module.hot.data) {
// for each component option object to be hot-reloaded,
// you need to create a record for it with a unique id.
// do this once on startup.
api.createRecord('very-unique-id', myComponentOptions)
} else {
// if a component has only its template or render function changed,
// you can force a re-render for all its active instances without
// destroying/re-creating them. This keeps all current app state intact.
api.rerender('very-unique-id', myComponentOptions)
// --- OR ---
// if a component has non-template/render options changed,
// it needs to be fully reloaded. This will destroy and re-create all its
// active instances (and their children).
api.reload('very-unique-id', myComponentOptions)
}
}
FAQs
hot reload api for *.vue components
The npm package vue-hot-reload-api receives a total of 497,888 weekly downloads. As such, vue-hot-reload-api popularity was classified as popular.
We found that vue-hot-reload-api demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
Security News
Research
A supply chain attack on Rspack's npm packages injected cryptomining malware, potentially impacting thousands of developers.
Research
Security News
Socket researchers discovered a malware campaign on npm delivering the Skuld infostealer via typosquatted packages, exposing sensitive data.