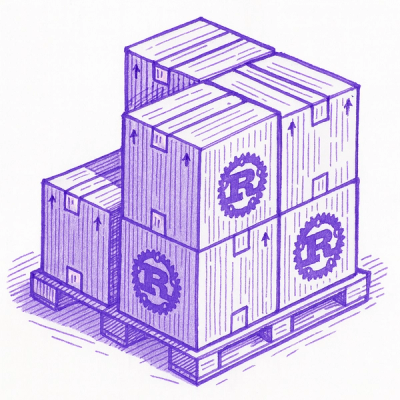
Security News
Crates.io Implements Trusted Publishing Support
Crates.io adds Trusted Publishing support, enabling secure GitHub Actions-based crate releases without long-lived API tokens.
vue-infi-scroller
Advanced tools
Vue-based infinite scroll component that just works. It supports window
and scrollable nodes, without inline style for height.
To install the component, run:
npm install vue-infi-scroller
To import the component in your project:
import InfiScroller from 'vue-infi-scroller';
Use InfiScroller
on the window object:
<template>
<div>
<InfiScroller
:has-more="hasMore"
:on-load-more="onLoadMore"
>
<ul>
<li
v-for="item in items"
:key="item.id"
style="height: 100px"
>
{{ `Item ${item.id}` }}
</li>
</ul>
</InfiScroller>
</div>
</template>
<script>
import InfiScroller from 'vue-infi-scroller';
export default {
components: { InfiScroller },
data() {
return {
items: this.generateItems(),
hasMore: true
};
},
methods: {
generateItems(items = [], length = 30) {
const nextItems = [...items, ...Array.from({ length })];
return nextItems.map((item, index) => ({ id: index }));
},
onLoadMore() {
const nextItems = this.generateItems(this.items);
this.items = nextItems;
this.hasMore = nextItems.length < 120;
}
}
};
</script>
Use InfiScroller
on a custom scroll target (like a modal):
<template>
<div>
<div
ref="scroller"
class="items-scroller"
>
<InfiScroller
:scroll-target="refScroller"
:has-more="hasMore"
:on-load-more="onLoadMore"
>
<ul>
<li
v-for="item in items"
:key="item.id"
style="height: 100px"
>
{{ `Item ${item.id}` }}
</li>
</ul>
</InfiScroller>
</div>
</div>
</template>
<script>
import InfiScroller from 'vue-infi-scroller';
export default {
components: { InfiScroller },
data() {
return {
refScroller: null,
items: this.generateItems(),
hasMore: true
};
},
mounted() {
this.refScroller = this.$refs.scroller;
},
methods: {
generateItems(items = [], length = 30) {
const nextItems = [...items, ...Array.from({ length })];
return nextItems.map((item, index) => ({ id: index }));
},
onLoadMore() {
const nextItems = this.generateItems(this.items);
this.items = nextItems;
this.hasMore = nextItems.length < 120;
}
}
};
</script>
<style>
.items-scroller {
height: 500px;
overflow: auto;
background-color: white;
}
</style>
Use multiple InfiScroller
components with custom scroll targets:
<template>
<div>
<div
ref="itemsScroller"
class="items-scroller"
>
<InfiScroller
:scroll-target="refItemsScroller"
:has-more="hasMore"
:on-load-more="onLoadMoreItems"
>
<ul>
<li
v-for="item in items"
:key="item.id"
style="height: 100px"
>
{{ `Item ${item.id}` }}
</li>
</ul>
</InfiScroller>
</div>
<div
ref="otherItemsScroller"
class="other-items-scroller"
>
<InfiScroller
:scroll-target="refOtherItemsScroller"
:has-more="hasMoreOther"
:on-load-more="onLoadMoreOtherItems"
>
<ul>
<li
v-for="item in otherItems"
:key="item.id"
style="height: 100px"
>
{{ `Other Item ${item.id}` }}
</li>
</ul>
</InfiScroller>
</div>
</div>
</template>
<script>
import InfiScroller from 'vue-infi-scroller';
export default {
components: { InfiScroller },
data() {
return {
refItemsScroller: null,
refOtherItemsScroller: null,
items: this.generateItems(),
otherItems: this.generateItems(),
hasMore: true,
hasMoreOther: true
};
},
mounted() {
this.refItemsScroller = this.$refs.itemsScroller;
this.refOtherItemsScroller = this.$refs.otherItemsScroller;
},
methods: {
generateItems(items = [], length = 30) {
const nextItems = [...items, ...Array.from({ length })];
return nextItems.map((item, index) => ({ id: index }));
},
onLoadMoreItems() {
const nextItems = this.generateItems(this.items);
this.items = nextItems;
this.hasMore = nextItems.length < 300;
},
onLoadMoreOtherItems() {
const nextOtherItems = this.generateItems(this.otherItems);
this.otherItems = nextOtherItems;
this.hasMoreOther = nextOtherItems.length < 120;
}
}
};
</script>
<style>
.items-scroller {
height: 300px;
overflow: auto;
background-color: white;
}
.other-items-scroller {
height: 500px;
margin-top: 40px;
overflow: auto;
background-color: white;
}
</style>
Use InfiScroller
with a spinner/loader:
<template>
<div>
<InfiScroller
:has-more="hasMore"
:on-load-more="onLoadMore"
>
<ul>
<li
v-for="item in items"
:key="item.id"
style="height: 100px"
>
{{ `Item ${item.id}` }}
</li>
</ul>
<span v-if="hasMore">Loading...</span>
</InfiScroller>
</div>
</template>
<script>
import InfiScroller from 'vue-infi-scroller';
export default {
components: { InfiScroller },
data() {
return {
items: this.generateItems(),
hasMore: true
};
},
methods: {
generateItems(items = [], length = 30) {
const nextItems = [...items, ...Array.from({ length })];
return nextItems.map((item, index) => ({ id: index }));
},
onLoadMore() {
const nextItems = this.generateItems(this.items);
this.items = nextItems;
this.hasMore = nextItems.length < 120;
}
}
};
</script>
Name | Type | Default | Description |
---|---|---|---|
children | Node | NodeList | The content in the infinite scroller. Contains the list of items you want to trigger infinite scrolling for. | |
scrollTarget | Node | null | The scroll target. Can be set to a custom scrollable node or omitted/null. When omitted/null the window object is used as scroll target. |
debounceDelay | Number | 300 | Debounce delay (in milliseconds) to optimize high-frequency scroll events. A recommended delay of 300 milliseconds is set by default. |
gutter | Number | 10 | Additional space (in pixels) used in the default shouldLoadMore calculation. Increasing it will cause the onLoadMore callback to be called before the scrollbar has reached the bottom of the scrollTarget . The larger the number, the earlier the onLoadMore callback will be called. A recommended minimum gutter of 10 pixels is set by default. |
immediate | Boolean | false | Whether to trigger an initial check, before any scroll event, if onLoadMore callback should be called. Set it to true when you want onLoadMore to be called immediately after the scrollTarget is mounted. This can be useful in case the scrollbar has been preset to the bottom of the scrollTarget or the content of the scrollTarget is less than its height and no scroll exist for it yet. |
active | Boolean | true | Turn on/off the infinite scroller. Keeps the component's children visible. Attaches event listeners when set to true . Detaches event listeners when set to false . Useful when the infinite scroller is placed inside a modal and you want it disabled until the modal is activated. |
hasMore | Boolean | false | Whether there are more items to load. This flag is used to determine if onLoadMore should be called. The entire check looks like this hasMore && shouldLoadMore(...) . |
shouldLoadMore | Function( scrollTargetHeight: number, scrollYOffset: number, gutter: number, scrollHeight: number ) | ( scrollTargetHeight, scrollYOffset, gutter, scrollHeight ) => ( scrollTargetHeight + scrollYOffset + gutter >= scrollHeight ) | Determine if more items should be loaded. By default a scrollTargetHeight + scrollYOffset + gutter >= scrollHeight formula is used. Provide a different function to customize this behavior. Note that shouldLoadMore will be called only if hasMore is true . |
onLoadMore | Function | Called when hasMore && shouldLoadMore(...) is true . You should load and render more items in the infinite scroller when onLoadMore is called. |
FAQs
Vue-based infinite scroll component
We found that vue-infi-scroller demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Crates.io adds Trusted Publishing support, enabling secure GitHub Actions-based crate releases without long-lived API tokens.
Research
/Security News
Undocumented protestware found in 28 npm packages disrupts UI for Russian-language users visiting Russian and Belarusian domains.
Research
/Security News
North Korean threat actors deploy 67 malicious npm packages using the newly discovered XORIndex malware loader.