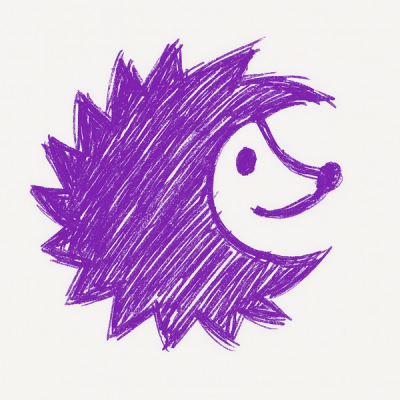
Security News
Browserslist-rs Gets Major Refactor, Cutting Binary Size by Over 1MB
Browserslist-rs now uses static data to reduce binary size by over 1MB, improving memory use and performance for Rust-based frontend tools.
vue-insert-compo
Advanced tools
A lightweight helper to insert Vue component and create Vue popups easily.
A lightweight helper to insert Vue component and create Vue popups easily. Check out Demo
vue-insert-compo can help you insert Vue component dynamically into the DOM tree. Some use cases are:
Supports all major browsers, IE >= 9.
NOTE: For IE 9, you need a Promise polyfill to use vue-insert-compo.
If you are using npm:
npm install vue-insert-compo --save
If you are using yarn:
yarn add vue-insert-compo
First, you need to create a Vue component that you want to insert dynamically:
// Alert.vue
<template>
<div v-if="enable"
styled="position: fixed; top: 50vh; left: 50vh;">
<div>You are using vue-insert-compo</div>
</div>
</template>
<script>
export default {
data () {
return {
// required
enable: true
}
}
}
</script>
Second, you can use vue-insert-compo to create a global instance (or a module):
import VueInsertCompo from 'vue-insert-compo'
import Alert from './Alert.vue'
const messenger = new VueInsertCompo(Alert)
messenger.enable() // Insert the Alert component into DOM tree
messenger.disable() // Hide the Alert component, it is still in memory, no pain to enable again
messenger.toggle() // Toggle the Alert component
messenger.destroy() // Destroy the Alert component
const insertCompo = new VueInsertCompo(Compo)
// or,
const insertCompo = new VueInsertCompo(Compo, {
hideEl: '#el-to-hide',
wrapperEl: '#wrapper-el'
})
Will show the component.
Will hide the component.
Will toggle the visibility of the component
Will clean up the VueInsertCompo instance and the DOM elements created.
FAQs
A lightweight helper to insert Vue component and create Vue popups easily.
We found that vue-insert-compo demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Browserslist-rs now uses static data to reduce binary size by over 1MB, improving memory use and performance for Rust-based frontend tools.
Research
Security News
Eight new malicious Firefox extensions impersonate games, steal OAuth tokens, hijack sessions, and exploit browser permissions to spy on users.
Security News
The official Go SDK for the Model Context Protocol is in development, with a stable, production-ready release expected by August 2025.