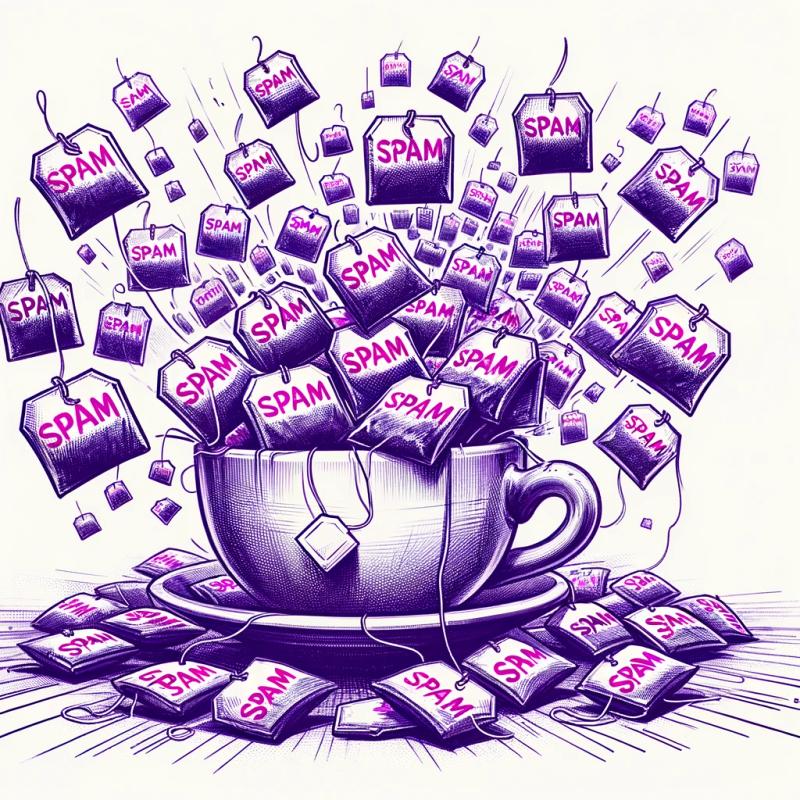
Security News
tea.xyz Spam Plagues npm and RubyGems Package Registries
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
vue-plugin-timers
Advanced tools
Readme
A Vue.JS plugin/mixin that helps set timeouts and heartbeats in your Vue components
Say we create a vue.js component
export default {
data: {
minutes: new Date().getMinutes()
}
}
What if we want minutes to update along with the user's clock when this component is open.
export default {
get minutes() {
return new Date().getMinutes()
}
}
But, unfortunately this does not work.
Reason ?
new Date()
or Date.now()
type of objects are not reactive
We get their value once, but it doesn't update every minutes/second or so
With vue-plugin-timers
we can create a timers
option in Vue
components. Timers run a method in your component every x
seconds
(where you can choose x).
We can activate timers in two ways
As a Component Mixin in any component
// @/components/AwesomeComponent.vue <script>
import { VueTimersMixin } from 'vue-plugin-timers'
export default {
mixins: [VueTimersMixin],
...,
timers: { ... }
}
As a global Vue Plugin if you want to use in multiple components
// @/main.js
import Vue from 'vue'
import VueTimers from 'vue-plugin-timers'
Vue.use(VueTimers)
You can use timers
property in all components now
// @/components/AnyComponent.vue <script>
export default {
...,
timers: { ... }
}
You can define timers in 2 ways -
timers
property in component options (in single-file-component export or inside Vue.extend())vue-class-component
, then there are @Timer
decorators 🎉timers
component option// @/components/AwesomeComponent.vue - <script>
export default {
data: {
time: new Date().toTimeString()
},
methods: {
updateTime() {this.time = new Date().toTimeString()}
},
timers: {
/* key = name of method */
updateMinutes: {
/* interval of delay (default: 1000) */
interval: 30000,
/* repeat (default: false)
true => setInterval,
false => setTimeout once */
repeat: true
}
}
}
@Timer
decorator⚠️ IMPORTANT: This needs
vue-class-component
to be installed, (orvue-property-decorator
)
import Vue from 'vue'
import Component from 'vue-class-component'
import { Timer } from 'vue-plugin-timer'
@Component
class TimerComponent extends Vue {
count = 0
@Timer({ interval: 200 })
incr() {
this.count++
}
}
The @Timer
decorator takes all the same options as the
timers
component options hashes do. Except the method name, because
you are decorating the method itself and so it is not needed
NOTE: The umd builds do NOT have the decorator, as decorators are something we usually use when building with Vue CLI using Babel and/or Typescript
FAQs
Interval and Timeout timers for Vue as a plugin or mixin
The npm package vue-plugin-timers receives a total of 155 weekly downloads. As such, vue-plugin-timers popularity was classified as not popular.
We found that vue-plugin-timers demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.
Security News
UnitedHealth Group disclosed that the ransomware attack on Change Healthcare compromised protected health information for millions in the U.S., with estimated costs to the company expected to reach $1 billion.