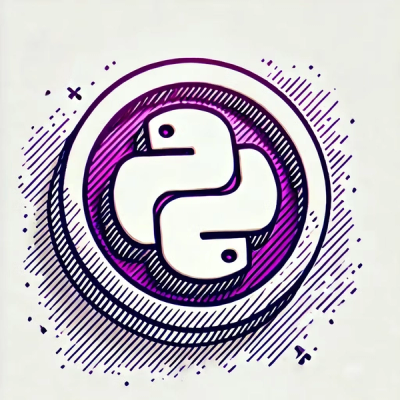
Product
Socket Now Supports pylock.toml Files
Socket now supports pylock.toml, enabling secure, reproducible Python builds with advanced scanning and full alignment with PEP 751's new standard.
vue-select
Advanced tools
Everything you wish the HTML <select> element could do, wrapped up into a lightweight, extensible Vue component.
Supply Chain Security
Vulnerability
Quality
Maintenance
License
vue-select is a Vue.js component that provides a customizable, single or multi-select dropdown with search functionality. It is designed to be flexible and easy to integrate into Vue applications, offering a range of features to enhance user experience.
Basic Usage
This code demonstrates the basic usage of vue-select, where a simple dropdown with predefined options is created.
<template>
<v-select :options="['Option 1', 'Option 2', 'Option 3']"></v-select>
</template>
<script>
import vSelect from 'vue-select'
export default {
components: { vSelect }
}
</script>
Custom Options
This example shows how to use custom objects as options in the dropdown, specifying a label to display.
<template>
<v-select :options="options" label="name"></v-select>
</template>
<script>
import vSelect from 'vue-select'
export default {
components: { vSelect },
data() {
return {
options: [
{ id: 1, name: 'Option 1' },
{ id: 2, name: 'Option 2' },
{ id: 3, name: 'Option 3' }
]
}
}
}
</script>
Multi-Select
This code demonstrates how to enable multi-select functionality, allowing users to select multiple options from the dropdown.
<template>
<v-select :options="['Option 1', 'Option 2', 'Option 3']" multiple></v-select>
</template>
<script>
import vSelect from 'vue-select'
export default {
components: { vSelect }
}
</script>
Searchable Dropdown
This example shows how to enable the search functionality within the dropdown, allowing users to filter options by typing.
<template>
<v-select :options="['Option 1', 'Option 2', 'Option 3']" searchable></v-select>
</template>
<script>
import vSelect from 'vue-select'
export default {
components: { vSelect }
}
</script>
Async Options
This code demonstrates how to load options asynchronously, which is useful for fetching data from an API based on user input.
<template>
<v-select :options="fetchOptions" :reduce="option => option.id"></v-select>
</template>
<script>
import vSelect from 'vue-select'
export default {
components: { vSelect },
methods: {
fetchOptions(search, loading) {
loading(true);
setTimeout(() => {
loading(false);
return [
{ id: 1, name: 'Option 1' },
{ id: 2, name: 'Option 2' },
{ id: 3, name: 'Option 3' }
];
}, 1000);
}
}
}
</script>
vue-multiselect is another Vue.js component for creating multi-select dropdowns with search functionality. It offers a similar feature set to vue-select but includes additional customization options and better support for complex data structures.
vue-selectize is a Vue.js wrapper for the Selectize.js library, providing a highly customizable and feature-rich select component. It offers advanced features like tagging, remote data loading, and custom rendering, making it a strong alternative to vue-select.
vue-treeselect is a multi-select component with nested options support, allowing users to select items from a tree structure. It is particularly useful for hierarchical data and offers features like async loading and custom rendering, which are not available in vue-select.
Everything you wish the HTML
<select>
element could do, wrapped up into a lightweight, zero dependency, extensible Vue component.
Vue Select is a feature rich select/dropdown/typeahead component. It provides a default template that fits most use cases for a filterable select dropdown. The component is designed to be as lightweight as possible, while maintaining high standards for accessibility, developer experience, and customization.
Complete documentation and examples available at https://vue-select.org.
It takes a lot of effort to maintain this project. If it has saved you development time, please consider sponsoring the project with GitHub sponsors!
Huge thanks to the sponsors and contributors that make Vue Select possible!
Vue 3 / Vue Select 4.x-beta
Vue 3 support is on the
beta
channel:vue-select@beta
, and will become the new default whenv4
is released. See #1579 for more details!
Install:
yarn add vue-select@beta
# or use npm
npm install vue-select@beta
Then, import and register the component:
# main.ts or main.js
import { createApp } from "vue";
import App from "./App.vue";
import { VueSelect } from "vue-select";
createApp(App)
.component("v-select", VueSelect)
.mount("#app");
The component itself does not include any CSS. You'll need to include it separately in your Component.vue:
<style>
@import "vue-select/dist/vue-select.css";
</style>
Vue 2 / Vue Select 3.x
Install:
yarn add vue-select
# or use npm
npm install vue-select
Then, import and register the component:
import Vue from "vue";
import vSelect from "vue-select";
Vue.component("v-select", vSelect);
The component itself does not include any CSS. You'll need to include it separately:
import "vue-select/dist/vue-select.css";
You can also include vue-select directly in the browser. Check out the documentation for loading from CDN..
FAQs
Everything you wish the HTML <select> element could do, wrapped up into a lightweight, extensible Vue component.
The npm package vue-select receives a total of 147,741 weekly downloads. As such, vue-select popularity was classified as popular.
We found that vue-select demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 9 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Socket now supports pylock.toml, enabling secure, reproducible Python builds with advanced scanning and full alignment with PEP 751's new standard.
Security News
Research
Socket uncovered two npm packages that register hidden HTTP endpoints to delete all files on command.
Research
Security News
Malicious Ruby gems typosquat Fastlane plugins to steal Telegram bot tokens, messages, and files, exploiting demand after Vietnam’s Telegram ban.