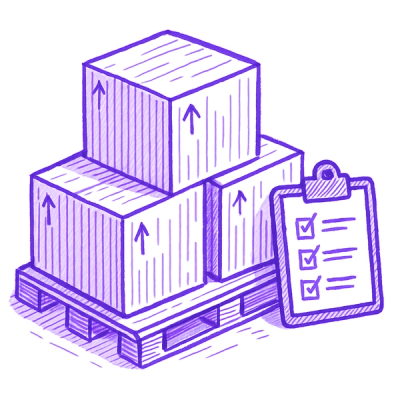
Security News
Open Source Maintainers Feeling the Weight of the EU’s Cyber Resilience Act
The EU Cyber Resilience Act is prompting compliance requests that open source maintainers may not be obligated or equipped to handle.
A JavaScript wrapper for the National Weather Service API - built with TypeScript.
A couple examples are below. There is also extensive typedoc generated documentation.
npm install weathered
import { Client } from 'weathered';
const client = new Client();
const active = true;
const latitude = 35.6175667;
const longitude = -80.7709911;
const alerts = await client.getAlerts(active, { latitude, longitude });
alerts.features.forEach(feature => {
console.log(feature.properties.description);
console.log(feature.geometry);
});
// At 744 PM EDT, Doppler radar indicated strong thunderstorms along a
// line extending from 11 miles southeast of Yadkinville to 6 miles
// south of Mocksville to 7 miles northwest of Huntersville, and moving
// east at 20 mph.
// {
// type: 'Polygon',
// coordinates: [
// [ [Array], [Array] ]
// ]
// }
const alerts = await client.getAlerts(active, { region: 'AL' });
alerts.features.forEach(feature => {
console.log(feature.properties.description);
console.log(feature.geometry);
});
// The Flood Warning continues for
// the Pearl River Above Philadelphia ...
// {
// type: 'Polygon',
// coordinates: [
// [ [Array], [Array] ]
// ]
// }
const forecast = await client.getForecast(latitude, longitude, 'baseline');
forecast.properties.periods.forEach(period => {
console.log(`${period.name}: ${period.detailedForecast}`);
});
// Today Partly sunny, with a high near 86. Northeast wind 2 to 6 mph.
// Tonight Partly cloudy, with a low around 68. South southeast wind around 3 mph.
const stations = await client.getStations(latitude, longitude);
stations.features.forEach(station => console.log(station.properties.name));
// San Francisco, San Francisco International Airport
// SAN FRANCISCO DOWNTOWN
// Half Moon Bay Airport
const nearestStation = await client.getNearestStation(latitude, longitude);
if (nearestStation) {
console.log(nearestStation.properties.stationIdentifier);
}
// KSFO
const nearestStation = await client.getNearestStation(latitude, longitude);
if (nearestStation) {
const { stationIdentifier } = nearestStation.properties;
const observations = await client.getStationObservations(stationIdentifier);
observations.features.forEach(obs => console.log(obs.properties.temperature));
// { value: 16.1, unitCode: 'unit:degC', qualityControl: 'qc:V' }
// { value: 16.7, unitCode: 'unit:degC', qualityControl: 'qc:V' }
// { value: 17.2, unitCode: 'unit:degC', qualityControl: 'qc:V' }
}
const latestObservation = await client.getLatestStationObservations('KSFO');
console.log(latestObservation.properties.relativeHumidity);
// { value: 64.486025639597, unitCode: 'unit:percent', qualityControl: 'qc:V' }
FAQs
A JavaScript wrapper for the National Weather Service API
The npm package weathered receives a total of 62 weekly downloads. As such, weathered popularity was classified as not popular.
We found that weathered demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
The EU Cyber Resilience Act is prompting compliance requests that open source maintainers may not be obligated or equipped to handle.
Security News
Crates.io adds Trusted Publishing support, enabling secure GitHub Actions-based crate releases without long-lived API tokens.
Research
/Security News
Undocumented protestware found in 28 npm packages disrupts UI for Russian-language users visiting Russian and Belarusian domains.