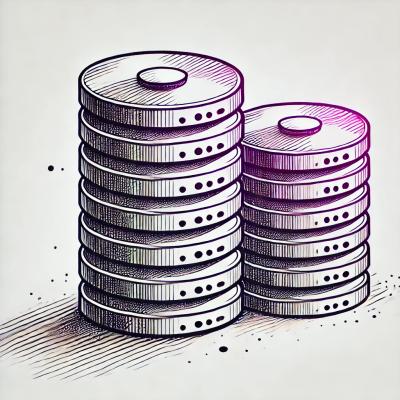
Security News
MCP Community Begins Work on Official MCP Metaregistry
The MCP community is launching an official registry to standardize AI tool discovery and let agents dynamically find and install MCP servers.
webrtc-easy-connect
Advanced tools
A simple, flexible WebRTC library for multiple frontend frameworks.
npm install webrtc-easy-connect
For detailed API documentation, see API Reference.
To build the library from source:
Clone the repository
git clone https://github.com/pious2847/WEB-RTC.git
cd webrtc-easy
Install dependencies
npm install
Build the library
npm run build
This will create the following outputs:
dist/
dist/
dist/webrtc-easy.min.js
The package includes several examples demonstrating different features:
Start the signaling server (required for most examples):
npm run start:signaling
This will start a WebSocket signaling server on port 3000.
Open examples/file-sharing/index.html
in your browser to try the file sharing example.
Open examples/audio-effects/index.html
in your browser to try the audio effects example.
Open examples/screen-recording/index.html
in your browser to try the screen recording example.
import { RTCConnection, WebSocketSignaling } from 'webrtc-easy';
// Create a signaling connection
const signaling = new WebSocketSignaling({
url: 'wss://your-signaling-server.com',
room: 'test-room'
});
// Create a WebRTC connection
const connection = new RTCConnection({
configuration: {
iceServers: [{ urls: 'stun:stun.l.google.com:19302' }]
}
});
// Get user media
const stream = await navigator.mediaDevices.getUserMedia({
video: true,
audio: true
});
// Add stream to connection
connection.addStream(stream);
// Set up signaling
signaling.onMessage(async (message) => {
switch (message.type) {
case 'offer':
const answer = await connection.createAnswer(message.payload);
signaling.send({ type: 'answer', payload: answer });
break;
case 'answer':
await connection.handleAnswer(message.payload);
break;
case 'ice-candidate':
await connection.addIceCandidate(message.payload);
break;
}
});
// Create and send offer
const offer = await connection.createOffer();
signaling.send({ type: 'offer', payload: offer });
import React, { useRef, useEffect } from 'react';
import { useWebRTC } from 'webrtc-easy/react';
import { WebSocketSignaling } from 'webrtc-easy/core';
const VideoChat = () => {
const localVideoRef = useRef<HTMLVideoElement>(null);
const remoteVideoRef = useRef<HTMLVideoElement>(null);
const {
localStream,
remoteStream,
connection,
connectionState,
error,
initConnection,
startScreenShare
} = useWebRTC({
configuration: {
iceServers: [{ urls: 'stun:stun.l.google.com:19302' }]
}
});
useEffect(() => {
if (localVideoRef.current && localStream) {
localVideoRef.current.srcObject = localStream;
}
}, [localStream]);
useEffect(() => {
if (remoteVideoRef.current && remoteStream) {
remoteVideoRef.current.srcObject = remoteStream;
}
}, [remoteStream]);
const handleStart = async () => {
const conn = await initConnection();
const signaling = new WebSocketSignaling({
url: 'wss://your-signaling-server.com',
room: 'test-room'
});
signaling.onMessage(async (message) => {
switch (message.type) {
case 'offer':
const answer = await conn.createAnswer(message.payload);
signaling.send({ type: 'answer', payload: answer });
break;
case 'answer':
await conn.handleAnswer(message.payload);
break;
case 'ice-candidate':
await conn.addIceCandidate(message.payload);
break;
}
});
const offer = await conn.createOffer();
signaling.send({ type: 'offer', payload: offer });
};
return (
<div>
<button onClick={handleStart}>Start Call</button>
<button onClick={startScreenShare}>Share Screen</button>
<div>
<h3>Local Video</h3>
<video ref={localVideoRef} autoPlay muted playsInline />
</div>
<div>
<h3>Remote Video</h3>
<video ref={remoteVideoRef} autoPlay playsInline />
</div>
</div>
);
};
<template>
<div>
<button @click="handleStart">Start Call</button>
<button @click="startScreenShare">Share Screen</button>
<div>
<h3>Local Video</h3>
<video ref="localVideo" autoplay muted playsinline />
</div>
<div>
<h3>Remote Video</h3>
<video ref="remoteVideo" autoplay playsinline />
</div>
</div>
</template>
<script setup>
import { ref, watch } from 'vue';
import { useWebRTC } from 'webrtc-easy/vue';
import { WebSocketSignaling } from 'webrtc-easy/core';
const localVideo = ref(null);
const remoteVideo = ref(null);
const {
localStream,
remoteStream,
connection,
connectionState,
error,
initConnection,
startScreenShare
} = useWebRTC({
configuration: {
iceServers: [{ urls: 'stun:stun.l.google.com:19302' }]
}
});
watch(localStream, (stream) => {
if (localVideo.value && stream) {
localVideo.value.srcObject = stream;
}
});
watch(remoteStream, (stream) => {
if (remoteVideo.value && stream) {
remoteVideo.value.srcObject = stream;
}
});
const handleStart = async () => {
const conn = await initConnection();
const signaling = new WebSocketSignaling({
url: 'wss://your-signaling-server.com',
room: 'test-room'
});
signaling.onMessage(async (message) => {
switch (message.type) {
case 'offer':
const answer = await conn.createAnswer(message.payload);
signaling.send({ type: 'answer', payload: answer });
break;
case 'answer':
await conn.handleAnswer(message.payload);
break;
case 'ice-candidate':
await conn.addIceCandidate(message.payload);
break;
}
});
const offer = await conn.createOffer();
signaling.send({ type: 'offer', payload: offer });
};
</script>
import { RTCConnection } from 'webrtc-easy';
const connection = new RTCConnection({
networkQuality: {
enabled: true,
onQualityChange: (metrics) => {
console.log('Network quality:', metrics.quality);
console.log('Score:', metrics.score);
console.log('Round trip time:', metrics.roundTripTime, 'ms');
console.log('Packet loss:', metrics.packetLoss, '%');
}
}
});
import { RTCConnection } from 'webrtc-easy';
const connection = new RTCConnection({
adaptiveStreaming: {
enabled: true,
config: {
maxBitrate: 2500, // kbps
minBitrate: 100, // kbps
targetQuality: 0.8 // 0-1 scale
}
}
});
// Start adaptive streaming
connection.startAdaptiveStreaming();
import { RTCConnection } from 'webrtc-easy';
const connection = new RTCConnection();
// Create a data channel
const channel = connection.createDataChannel('chat');
// Send data
connection.sendData('chat', 'Hello, world!');
// Receive data
connection.onDataChannelMessage('chat', (data) => {
console.log('Received:', data);
});
For detailed API documentation, see the API Reference.
Check out the examples directory for complete working examples.
MIT
FAQs
Easy to use WebRTC library for multiple frontend frameworks
The npm package webrtc-easy-connect receives a total of 9 weekly downloads. As such, webrtc-easy-connect popularity was classified as not popular.
We found that webrtc-easy-connect demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
The MCP community is launching an official registry to standardize AI tool discovery and let agents dynamically find and install MCP servers.
Research
Security News
Socket uncovers an npm Trojan stealing crypto wallets and BullX credentials via obfuscated code and Telegram exfiltration.
Research
Security News
Malicious npm packages posing as developer tools target macOS Cursor IDE users, stealing credentials and modifying files to gain persistent backdoor access.