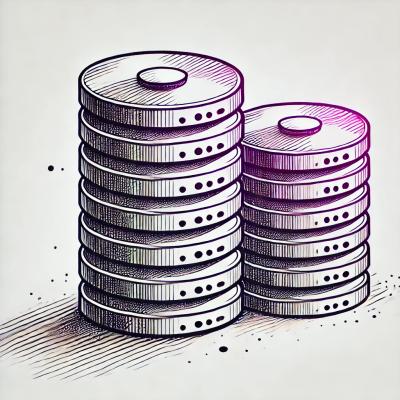
Security News
MCP Community Begins Work on Official MCP Metaregistry
The MCP community is launching an official registry to standardize AI tool discovery and let agents dynamically find and install MCP servers.
A wide-character aware text alignment function for use on the console or with fixed width fonts.
The wide-align npm package is designed to align text strings in console output, taking into consideration the width of characters in environments where character width can vary (e.g., Unicode characters). It is particularly useful for creating neatly formatted console tables or aligning text in a way that accommodates wide characters.
Align text to the center
This feature allows you to center-align a string within a given space. It is useful for creating headers or titles in console output that are visually centered, regardless of the terminal width.
"const wideAlign = require('wide-align');\nconsole.log(wideAlign.center('Some text'));"
Align text to the left
This feature enables left alignment of a string with a specified total width. It's particularly useful for aligning text in columns where the first column's content should start at the same horizontal position.
"const wideAlign = require('wide-align');\nconsole.log(wideAlign.left('Some text', 20));"
Align text to the right
Right-aligns a string within a specified total width. This is useful for aligning numbers or any content that should be right-aligned for better readability or aesthetic reasons.
"const wideAlign = require('wide-align');\nconsole.log(wideAlign.right('Some text', 20));"
While not directly offering alignment functionalities, string-width is a package that can be used in conjunction with others to achieve similar results. It calculates and returns the visual width of a string, which is crucial for accurately aligning text that includes wide characters. It's a complementary tool rather than a direct alternative.
A wide-character aware text alignment function for use in terminals / on the console.
var align = require('wide-align')
// Note that if you view this on a unicode console, all of the slashes are
// aligned. This is because on a console, all narrow characters are
// an en wide and all wide characters are an em. In browsers, this isn't
// held to and wide characters like "古" can be less than two narrow
// characters even with a fixed width font.
console.log(align.center('abc', 10)) // ' abc '
console.log(align.center('古古古', 10)) // ' 古古古 '
console.log(align.left('abc', 10)) // 'abc '
console.log(align.left('古古古', 10)) // '古古古 '
console.log(align.right('abc', 10)) // ' abc'
console.log(align.right('古古古', 10)) // ' 古古古'
align.center(str, length)
→ str
Returns str with spaces added to both sides such that that it is length chars long and centered in the spaces.
align.left(str, length)
→ str
Returns str with spaces to the right such that it is length chars long.
align.right(str, length)
→ str
Returns str with spaces to the left such that it is length chars long.
These functions were originally taken from cliui. Changes include switching to the MUCH faster pad generation function from lodash, making center alignment pad both sides and adding left alignment.
FAQs
A wide-character aware text alignment function for use on the console or with fixed width fonts.
The npm package wide-align receives a total of 11,977,411 weekly downloads. As such, wide-align popularity was classified as popular.
We found that wide-align demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
The MCP community is launching an official registry to standardize AI tool discovery and let agents dynamically find and install MCP servers.
Research
Security News
Socket uncovers an npm Trojan stealing crypto wallets and BullX credentials via obfuscated code and Telegram exfiltration.
Research
Security News
Malicious npm packages posing as developer tools target macOS Cursor IDE users, stealing credentials and modifying files to gain persistent backdoor access.