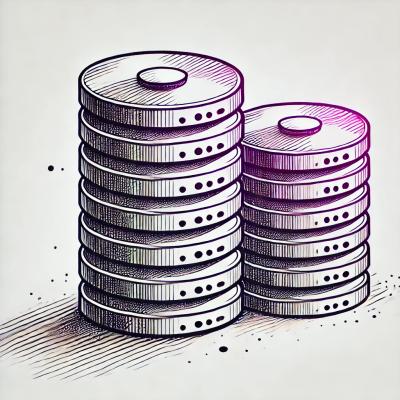
Security News
MCP Community Begins Work on Official MCP Metaregistry
The MCP community is launching an official registry to standardize AI tool discovery and let agents dynamically find and install MCP servers.
windows-release
Advanced tools
Get the name of a Windows version from the release number: `5.1.2600` → `XP`
The windows-release npm package is used to get the name of a Windows version from the release number. This can be particularly useful for applications that need to display or log the Windows version in a human-readable format.
Get Windows Version Name
This feature allows you to get the name of the Windows version based on the release number. By default, it uses the current operating system's release number.
const windowsRelease = require('windows-release');
console.log(windowsRelease()); // e.g., '10' for Windows 10
Get Windows Version Name from Specific Release Number
This feature allows you to get the name of the Windows version from a specific release number. This can be useful for parsing and displaying version information from logs or other data sources.
const windowsRelease = require('windows-release');
console.log(windowsRelease('10.0.19041')); // '10' for Windows 10
The os-name package provides a more general solution for getting the name of the operating system, including Windows, macOS, and Linux. It offers broader functionality compared to windows-release, which is specific to Windows.
The os-release package is another alternative that provides information about the operating system release. It is more focused on Linux distributions but can be used in conjunction with other tools to get similar information for Windows.
Get the name of a Windows version from the release number:
5.1.2600
→XP
npm install windows-release
import os from 'node:os';
import windowsRelease from 'windows-release';
// On a Windows XP system
windowsRelease();
//=> 'XP'
os.release();
//=> '5.1.2600'
windowsRelease(os.release());
//=> 'XP'
windowsRelease('4.9.3000');
//=> 'ME'
Type: string
By default, the current OS is used, but you can supply a custom release number, which is the output of os.release()
.
Note: Most Windows Server versions cannot be detected based on the release number alone. There is runtime detection in place to work around this, but it will only be used if no argument is supplied, or the supplied argument matches os.release()
.
FAQs
Get the name of a Windows version from the release number: `5.1.2600` → `XP`
The npm package windows-release receives a total of 2,801,274 weekly downloads. As such, windows-release popularity was classified as popular.
We found that windows-release demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
The MCP community is launching an official registry to standardize AI tool discovery and let agents dynamically find and install MCP servers.
Research
Security News
Socket uncovers an npm Trojan stealing crypto wallets and BullX credentials via obfuscated code and Telegram exfiltration.
Research
Security News
Malicious npm packages posing as developer tools target macOS Cursor IDE users, stealing credentials and modifying files to gain persistent backdoor access.