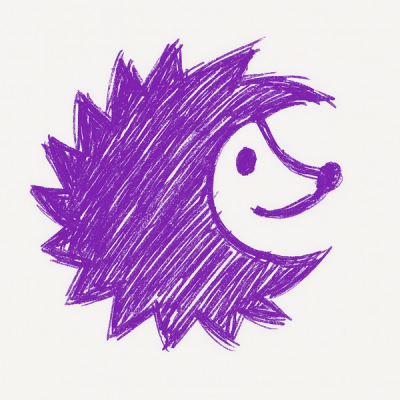
Security News
Browserslist-rs Gets Major Refactor, Cutting Binary Size by Over 1MB
Browserslist-rs now uses static data to reduce binary size by over 1MB, improving memory use and performance for Rust-based frontend tools.
wrn-echarts
Advanced tools
React Native version of Apache Echarts, based on react-native-svg and react-native-skia. Much better performance than webview based solution.
Checkout the full documentation here.
yarn add wrn-echarts echarts
Install react-native-svg or react-native-skia according to your needs.
The latest versions of echarts, react-native-svg and react-native-skia are recommended
Most of the charts in echarts are supported, and the usage remains largely consistent. For more use cases and demo previews, you can download the Taro Playground app.
// import { SkiaChart, SVGRenderer } from 'wrn-echarts';
import SkiaChart, { SVGRenderer } from 'wrn-echarts/skiaChart';
import * as echarts from 'echarts/core';
import { useRef, useEffect } from 'react';
import {
BarChart,
} from 'echarts/charts';
import {
TitleComponent,
TooltipComponent,
GridComponent,
} from 'echarts/components';
// register extensions
echarts.use([
TitleComponent,
TooltipComponent,
GridComponent,
SVGRenderer,
// ...
BarChart,
])
const E_HEIGHT = 250;
const E_WIDTH = 300;
// initial
function SkiaComponent({ option }) {
const skiaRef = useRef<any>(null);
useEffect(() => {
let chart: any;
if (skiaRef.current) {
// @ts-ignore
chart = echarts.init(skiaRef.current, 'light', {
renderer: 'svg',
width: E_WIDTH,
height: E_HEIGHT,
});
chart.setOption(option);
}
return () => chart?.dispose();
}, [option]);
return <SkiaChart ref={skiaRef} />;
}
// Component usage
export default function App() {
const option = {
xAxis: {
type: 'category',
data: ['Mon', 'Tue', 'Wed', 'Thu', 'Fri', 'Sat', 'Sun'],
},
yAxis: {
type: 'value',
},
series: [
{
data: [120, 200, 150, 80, 70, 110, 130],
type: 'bar',
},
],
}
return <SkiaComponent option={option} />
}
// import { SvgChart, SVGRenderer } from 'wrn-echarts';
import SvgChart, { SVGRenderer } from 'wrn-echarts/svgChart';
import * as echarts from 'echarts/core';
import { useRef, useEffect } from 'react';
import {
BarChart,
} from 'echarts/charts';
import {
TitleComponent,
TooltipComponent,
GridComponent,
} from 'echarts/components';
// register extensions
echarts.use([
TitleComponent,
TooltipComponent,
GridComponent,
SVGRenderer,
// ...
BarChart,
])
const E_HEIGHT = 250;
const E_WIDTH = 300;
// initial
function SvgComponent({ option }) {
const svgRef = useRef<any>(null);
useEffect(() => {
let chart: any;
if (svgRef.current) {
// @ts-ignore
chart = echarts.init(svgRef.current, 'light', {
renderer: 'svg',
width: E_WIDTH,
height: E_HEIGHT,
});
chart.setOption(option);
}
return () => chart?.dispose();
}, [option]);
return <SvgChart ref={svgRef} />;
}
// Component usage
export default function App() {
const option = {
xAxis: {
type: 'category',
data: ['Mon', 'Tue', 'Wed', 'Thu', 'Fri', 'Sat', 'Sun'],
},
yAxis: {
type: 'value',
},
series: [
{
data: [120, 200, 150, 80, 70, 110, 130],
type: 'bar',
},
],
}
return <SvgComponent option={option} />
}
import SvgChart, { SVGRenderer } from 'wrn-echarts/svgChart';
or
import SkiaChart, { SVGRenderer } from 'wrn-echarts/skiaChart';
See the contributing guide to learn how to contribute to the repository and the development workflow.
Apache-2.0
Made with create-react-native-library
FAQs
Echarts for react native.
The npm package wrn-echarts receives a total of 125 weekly downloads. As such, wrn-echarts popularity was classified as not popular.
We found that wrn-echarts demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Browserslist-rs now uses static data to reduce binary size by over 1MB, improving memory use and performance for Rust-based frontend tools.
Research
Security News
Eight new malicious Firefox extensions impersonate games, steal OAuth tokens, hijack sessions, and exploit browser permissions to spy on users.
Security News
The official Go SDK for the Model Context Protocol is in development, with a stable, production-ready release expected by August 2025.