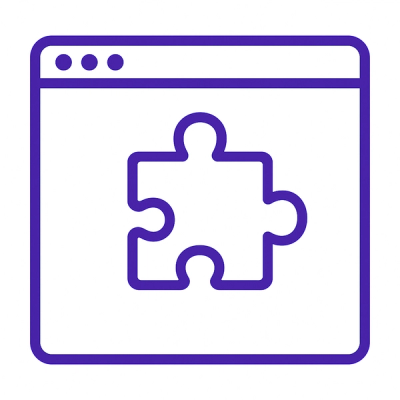
Research
Security News
The Growing Risk of Malicious Browser Extensions
Socket researchers uncover how browser extensions in trusted stores are used to hijack sessions, redirect traffic, and manipulate user behavior.
This is a simple way to raise a server in Node.JS.
npm install x-easy --save
// or
yarn add x-easy
//<project_path>/index.js
require('x-easy')
// the default port is 3000
// global object call. possibilities:
// XEasy, xEasy, xeasy, XEASY
XEasy.createApplication('myApp', { // Options for initialize app. It's opcional
port: 3000, // default
enableWebsocket: false, // default
enableSession: false // default
secureKeys: {
key: './keyFile.key', // or './keyFile.pem'
cert: './certFile.crt', // or './certFile.pem'
ca: ['./ca1.crt', './ca2.crt'] // chain certificate or (ca: './ca.crt') single certificate
requestCert: false, // default
rejectUnauthorized: false // default
}
})
// addModule can load <project_path>/<module_name>/index.js, <project_path>/<module_name>/index.json,
// <project_path>/<module_name>.js and <project_path>/<module_name>.json
// file to load data
.addModule('data') // json file
// file to default settings
.addModule('config') // js file
// file for route settings
.addModule('routes') // js file
// file for websocket settings
.addModule('websocket') // js file
// method for creating channel between processes
.join('chat')
// start children process
/* 'auto' sets automatic scanning for the number of processor
** cores and creates processes for each of them
** .start('auto') with 6 cores start 6 process
**
** negative numbers subtract from the number of cores to
** generate the number of processes that at least 1
** .start(-2) with 6 cores start 4 process*/
.start(/* number of process, default is 1*/)
The loadModule function is used to instantiate objects in the application by passing it in the constructor.
//data.json
{
'data': 'something else',
'viewEngine': 'pug',
'viewEnginePath': 'pages'
}
//config.js
module.exports = class Config {
// order of the constructor parameters does not matter
constructor(app, modules) {
// event called when the process is exiting
app.callStop = () => {
//... stopping worker
}
// method that receives messages from channels between processes
app.addProcessMessageListener((channel, data) => {
// ...message processing
// channel attribute refers to the channel name or application name
})
// for password protection, this method transforms the hashed data
app.setParameterHash('pwd')
app.setParameterHash(
'password',
/* optional */ {
seed: 'secret', // default is ''
loop: 5 // default is 1
}
)
// children properties can also be digested
app.setParameterHash('user.password')
// method to take a public folder
app.setPublic('public')
// if there are subfolders
// app.setPublic('public/images')
// page rendering system
app.setViewEngine(modules.data.viewEngine)
app.setViewPath(modules.data.viewEnginePath)
// permission for accept upload of files
app.addUploadURLPermission('/upload')
}
}
//routes.js
module.exports = class Routes {
// again! order of the constructor parameters does not matter
constructor(modules, app) {
app.get('/', function(req, res) {
// Sending messages to all registered processes on this channel
app.sendProcessMessage(
'chat',
{ foo: 'foo' } /* worker id 'app.worker.id' */
) // the message has to be an object
// Sending messages to all processes on this application
app.sendAppProcessMessage({ foo: 'foo' } /* worker id 'app.worker.id' */) // the message has to be an object too
res.end('test ok - ' + req.query.test)
})
/** CAUTION **/
// the file will be loaded into RAM memory
app.post('/upload', (req, res) => {
// ... file upload process
// req.files.<form name>.name - file name
// req.files.<form name>.encoding - file encoding
// req.files.<form name>.mimetype - file mimetype
// req.files.<form name>.data - Buffer bytes
res.end('file upload success')
})
}
}
//websocket.js
const URL = require('url')
module.exports = class WebSocket {
// again! order of the constructor parameters does not matter
// the definition of possibilities is in the part of
// Constructor Parameters
constructor(wss) {
wss.on('request', req => {
// ... process req
if(false/**...process req...**/) req.reject()
const client = req.accept()
conn.on('message', msg => {
// ... process message
}
conn.on('close', () => {
// ... process close connection
})
})
}
}
The websocket api documentation is located on this link
server // http server object
app // XEasy app control
name // application name entered in the creation
worker // child process control object
wss || websocketServer || wsServer // Websocket server
modules // list of added modules in application
digest // convert data into hash sha512
FAQs
Make simple and modular, building a web application with express.
The npm package x-easy receives a total of 9 weekly downloads. As such, x-easy popularity was classified as not popular.
We found that x-easy demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers uncover how browser extensions in trusted stores are used to hijack sessions, redirect traffic, and manipulate user behavior.
Research
Security News
An in-depth analysis of credential stealers, crypto drainers, cryptojackers, and clipboard hijackers abusing open source package registries to compromise Web3 development environments.
Security News
pnpm 10.12.1 introduces a global virtual store for faster installs and new options for managing dependencies with version catalogs.