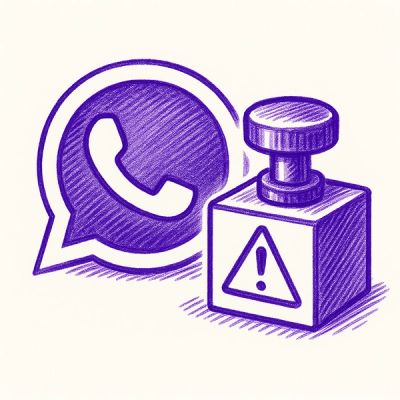
Research
/Security News
Malicious npm Packages Target WhatsApp Developers with Remote Kill Switch
Two npm packages masquerading as WhatsApp developer libraries include a kill switch that deletes all files if the phone number isn’t whitelisted.
xlsx-to-csv
Advanced tools
This library provides an easy way to convert Excel files (.xlsx
, .xls
, .xlsm
, etc.) to CSV format using custom
options such as specifying a sheet, delimiter, datetime format, and destination. It uses a Rust-powered backend for
efficient processing.
To use this library, install the required NPM package by running:
# Using npm
npm install xlsx-to-csv
# Using yarn
yarn add xlsx-to-csv
Make sure to have the binary executable for the conversion process set up as per your platform requirements.
convertFile(filePath: string, options: ConvertFileOptions = {}): string
Converts an Excel file to CSV format with customizable options.
filePath
(string
): Path to the Excel file you want to convert.
options
(ConvertFileOptions
, optional): An object containing various settings to customize the conversion process.
ConvertFileOptions
The options object allows you to configure the conversion with the following fields:
Field | Type | Description | Default |
---|---|---|---|
sheet | string | Sheet name to convert. If not provided, the first sheet will be used. | First sheet |
delimiter | string | Delimiter to use in the CSV file. You can choose between ; , , , or any custom delimiter string. | ; |
dest | string | Destination file path. If set to 'return' , it will return the CSV data as a string. If not specified, it will print to stdout . | stdout |
datetimeFormat | string | Format for parsing datetime values in the sheet. Follows the chrono format documentation. | %Y-%m-%d %H:%M:%S |
import {convertFile} from 'your-library-name';
// Convert an Excel file to CSV and save it to a specific path
convertFile('example.xlsx', {
sheet: 'Sheet1',
delimiter: ',',
dest: 'output.csv',
datetimeFormat: '%d-%m-%Y %H:%M'
});
// Convert an Excel file to CSV and return the result as a string
const csvData = convertFile('example.xlsx', {
sheet: 'Sheet1',
delimiter: ';',
dest: 'return',
datetimeFormat: '%Y-%m-%d'
});
console.log(csvData);
This library internally runs a binary command with the following mappings:
Option | CLI Argument |
---|---|
filePath | --file <filePath> |
sheet | --sheet <sheet> |
delimiter | --delimiter <delimiter> |
dest | --dest <dest> |
datetimeFormat | --datetime-format <format> |
.xlsx
, .xls
, .xlsm
, .xlsb
).datetimeFormat
, it uses the popular chrono crate for flexible and powerful date
formatting options.yarn install
.cargo build
.rustup target add x86_64-unknown-linux-gnu
rustup target add aarch64-apple-darwin
rustup target add x86_64-apple-darwin
[target.x86_64-unknown-linux-gnu]
linker = "x86_64-unknown-linux-gnu-gcc"
[target.x86_64-pc-windows-gnu]
linker = "x86_64-w64-mingw32-gcc"
This project is licensed under the MIT License.
Feel free to open an issue or contribute to the repository if you encounter any bugs or have feature suggestions!
FAQs
Ultra-fast XLSX to CSV converter
The npm package xlsx-to-csv receives a total of 155 weekly downloads. As such, xlsx-to-csv popularity was classified as not popular.
We found that xlsx-to-csv demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
/Security News
Two npm packages masquerading as WhatsApp developer libraries include a kill switch that deletes all files if the phone number isn’t whitelisted.
Research
/Security News
Socket uncovered 11 malicious Go packages using obfuscated loaders to fetch and execute second-stage payloads via C2 domains.
Security News
TC39 advances 11 JavaScript proposals, with two moving to Stage 4, bringing better math, binary APIs, and more features one step closer to the ECMAScript spec.