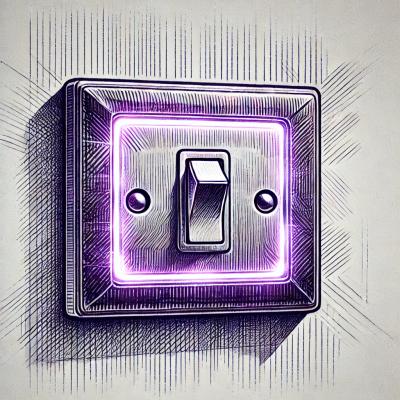
Research
Security News
Kill Switch Hidden in npm Packages Typosquatting Chalk and Chokidar
Socket researchers found several malicious npm packages typosquatting Chalk and Chokidar, targeting Node.js developers with kill switches and data theft.
The xxhashjs npm package is a pure JavaScript implementation of the XXHash algorithm, which is a fast non-cryptographic hash algorithm. It is used primarily for generating consistent hash values of data, such as strings or binary content, with high performance.
Hashing a string
This feature allows you to hash a string using the XXHash algorithm with a specified seed. The result is a hash value that can be used for checksums, fingerprinting, or other purposes where a unique identifier for data is needed.
"use strict";
const XXH = require('xxhashjs');
const H = XXH.h32(0xABCD) // seed = 0xABCD
const buf = new Buffer('hello world');
const hash = H.update(buf).digest().toString(16); // hash is a hexadecimal string
console.log(hash);
Hashing a file stream
This feature demonstrates how to hash the contents of a file stream. As the stream is read, chunks are continuously updated to the hash function, and the final hash value is computed at the end of the stream.
"use strict";
const fs = require('fs');
const XXH = require('xxhashjs');
const H = XXH.h32(0xABCD);
const stream = fs.createReadStream('example.txt');
stream.on('data', function (chunk) {
H.update(chunk);
});
stream.on('end', function () {
const hash = H.digest().toString(16);
console.log(hash);
});
This package is a binding to the native C++ implementation of XXHash. It offers better performance than xxhashjs but requires a compilation step during installation, which may not be suitable for all environments.
hash-wasm provides a variety of hash functions compiled to WebAssembly for high performance. It includes an XXHash implementation and can be faster than pure JavaScript implementations, but it relies on WebAssembly support.
crypto-js is a collection of cryptographic algorithms implemented in JavaScript. While it doesn't include XXHash, it offers a wide range of hash functions like SHA-1, SHA-256, and MD5. It is suitable for cryptographic purposes rather than just fast hashing.
xxHash is a very fast hashing algorithm (see the details here). xxhashjs is a Javascript implementation of it, written in 100% Javascript. Although not as fast as the C version, it does perform pretty well given the current Javascript limitations in handling unsigned 32 bits integers.
In nodejs:
npm install xxhashjs
In the browser, include the following, and access the constructor with XXH:
<script src="/your/path/to/xxhash.js"></script>
var h = XXH.h32( 'abcd', 0xABCD ).toString(16) // seed = 0xABCD
0xCDA8FAE4
var H = XXH.h32( 0xABCD ) // seed = 0xABCD
var h = H.update( 'abcd' ).digest().toString(16)
0xCDA8FAE4
XXH makes 2 functions available for 32 bits XXH and 64 bits XXH respectively, with the same signature:
In one step:
XXH.h32(<data>, <seed>)
The data can either be a string, an ArrayBuffer or a NodeJS Buffer object.
The seed can either be a number or a UINT32 object.
In several steps:
instantiate a new XXH object H:
XXH.h32(<seed>)
or XXH.h32()
The seed can be set later on with the init
method
add data to the hash calculation:
H.update(<data>)
finish the calculations:
H.digest()
The object returned can be converted to a string with toString(<radix>)
or a number toNumber()
.
Once digest()
has been called, the object can be reused. The same seed will be used or it can be changed with init(<seed>)
.
XXH.h32()
.init(<seed>)
Initialize the XXH object with the given seed. The seed can either be a number or a UINT32 object..update(<data>)
Add data for hashing. The data can either be a string, an ArrayBuffer or a NodeJS Buffer object.digest()
(UINT32)
Finalize the hash calculations and returns an UINT32 object. The hash value can be retrieved with toString().
XXH.h64()
.init(<seed>)
Initialize the XXH object with the given seed. The seed can either be a number or a UINT64 object..update(<data>)
Add data for hashing. The data can either be a string, an ArrayBuffer or a NodeJS Buffer object..digest()
(UINT64)
Finalize the hash calculations and returns an UINT64 object. The hash value can be retrieved with toString().MIT
FAQs
xxHash in Javascript
The npm package xxhashjs receives a total of 1,053,261 weekly downloads. As such, xxhashjs popularity was classified as popular.
We found that xxhashjs demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers found several malicious npm packages typosquatting Chalk and Chokidar, targeting Node.js developers with kill switches and data theft.
Security News
pnpm 10 blocks lifecycle scripts by default to improve security, addressing supply chain attack risks but sparking debate over compatibility and workflow changes.
Product
Socket now supports uv.lock files to ensure consistent, secure dependency resolution for Python projects and enhance supply chain security.