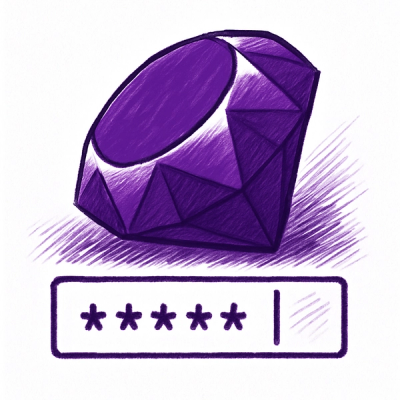
Research
/Security News
60 Malicious Ruby Gems Used in Targeted Credential Theft Campaign
A RubyGems malware campaign used 60 malicious packages posing as automation tools to steal credentials from social media and marketing tool users.
Zodios is a typescript api client with auto-completion features backed by axios and zod
It's an axios compatible API client, with the following features:
> npm install zodios
or
> yarn add zodios
For an almost complete example on how to use zodios and how to split your APIs declarations, take a look at dev.to example.
Here is an example of API declaration with Zodios.
import { Zodios } from "zodios";
import { z } from "zod";
const apiClient = new Zodios(
"https://jsonplaceholder.typicode.com",
// API definition
[
{
method: "get",
path: "/users/:id", // auto detect :id and ask for it in apiClient get params
description: "Get a user",
response: z.object({
id: z.number(),
name: z.string(),
}),
},
] as const,
);
Calling this API is now easy and has builtin autocomplete features :
// typed auto-complete path auto-complete params
// ▼ ▼ ▼
const user = await apiClient.get("/users/:id", { params: { id: 7 } });
console.log(user);
It should output
{ id: 7, name: 'Kurtis Weissnat' }
Zodios comes with a plugin to inject and renew your tokens :
import { pluginToken } from 'zodios/plugins/token';
apiClient.use(pluginToken({
getToken: async () => "token"
}));
you can get back the underlying axios instance to customize it.
const axiosInstance = apiClient.axios;
you can instanciate zodios with your own axios intance.
const apiClient = new Zodios(
"https://jsonplaceholder.typicode.com",
[ ... ] as const,
// Optional Axios instance
{
axiosIntance: customAxiosInstance
}
);
const apiClient = new Zodios(
"https://jsonplaceholder.typicode.com",
[ ... ] as const,
// Disable validation
{
validateResponse: false
}
);
Zodios comes with a Query and Mutation hook helper.
It's a thin wrapper around React-Query but with zodios auto completion.
Zodios query hook also returns an invalidation helper to allow you to reset react query cache easily
import { QueryClient, QueryClientProvider } from 'react-query';
import { Zodios } from "zodios";
import { ZodiosHooks } from "zodios/react";
import { z } from "zod";
const userSchema = z
.object({
id: z.number(),
name: z.string(),
});
const usersSchema = z.array(userSchema);
type User = z.infer<typeof userSchema>;
type Users = z.infer<typeof usersSchema>;
const api = [
{
method: "get",
path: "/users",
description: "Get all users",
response: usersSchema,
},
{
method: "post",
path: "/users",
description: "Create a user",
parameters: [
{
name: "body",
type: "Body",
schema: userSchema,
},
],
response: userSchema,
},
] as const;
const baseUrl = "https://jsonplaceholder.typicode.com";
const zodios = new Zodios(baseUrl, api);
const zodiosHooks = new ZodiosHooks("jsonplaceholder", zodios);
const Users = () => {
const {
data: users,
isLoading,
error,
invalidate: invalidateUsers,
} = zodiosHooks.useQuery("/users");
const { mutate } = zodiosHooks.useMutation("post", "/users", {
onSuccess: () => invalidateUsers(), // zodios also provides invalidation helpers
});
return (
<div>
<h1>Users</h1>
<button onClick={() => mutate({ data: { id: 10, name: "john doe" } })}>
add user
</button>
{isLoading && <div>Loading...</div>}
{error && <div>Error: {(error as Error).message}</div>}
{users && (
<ul>
{users.map((user) => (
<li key={user.id}>{user.name}</li>
))}
</ul>
)}
</div>
);
};
// on another file
const queryClient = new QueryClient();
export const App = () => {
return (
<QueryClientProvider client={queryClient}>
<Users />
</QueryClientProvider>
);
};
FAQs
Typescript API client with autocompletion and zod validations
The npm package zodios receives a total of 733 weekly downloads. As such, zodios popularity was classified as not popular.
We found that zodios demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
/Security News
A RubyGems malware campaign used 60 malicious packages posing as automation tools to steal credentials from social media and marketing tool users.
Security News
The CNA Scorecard ranks CVE issuers by data completeness, revealing major gaps in patch info and software identifiers across thousands of vulnerabilities.
Research
/Security News
Two npm packages masquerading as WhatsApp developer libraries include a kill switch that deletes all files if the phone number isn’t whitelisted.