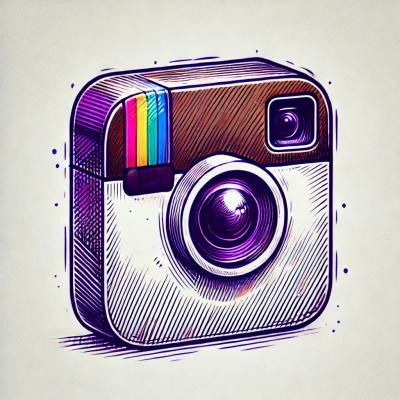
Research
PyPI Package Disguised as Instagram Growth Tool Harvests User Credentials
A deceptive PyPI package posing as an Instagram growth tool collects user credentials and sends them to third-party bot services.
*TestBase* gives you a flying start with - fluent assertions that are easy to extend - sharp error messages - tools to help you test with “heavyweight” dependencies on - AspNetCore.Mvc, AspNet.Mvc or WebApi Contexts - HttpClient - Ado.Net - Streams & Logging - Mix & match with your favourite test runners & assertions. ``` UnitUnderTest.Action() .ShouldNotBeNull() .ShouldEqualByValueExceptFor(new {Id=1, Descr=expected}, ignoreList ) .Payload .ShouldMatchIgnoringCase("I expected this") .Should(someOtherPredicate); .Items .ShouldAll(predicate) .ShouldContain(item) .ShouldNotContain(predicate) .Where(predicate) .SingleOrAssertFail() .ShouldEqualByValue().ShouldEqualByValueExceptFor(...).ShouldEqualByValueOnMembers() work with all kinds of object and collections, and report what differed. string.ShouldMatch(pattern).ShouldNotMatch().ShouldBeEmpty().ShouldNotBeEmpty() .ShouldNotBeNullOrEmptyOrWhiteSpace().ShouldEqualIgnoringCase() .ShouldContain().ShouldStartWith().ShouldEndWith().ShouldBeContainedIn(), ... numeric.ShouldBeBetween().ShouldEqualWithTolerance()....GreaterThan....LessThan...GreaterOrEqualTo ... ienumerable.ShouldAll().ShouldContain().ShouldNotContain().ShouldBeEmpty().ShouldNotBeEmpty() ... stream.ShouldHaveSameStreamContentAs().ShouldContain() value.ShouldBe().ShouldNotBe().ShouldBeOfType().ShouldBeAssignableTo()... ``` Testable Logging is in packages Extensions.Logging.ListOfString and Serilog.Sinks.ListOfString ``` // Extensions.Logging.ListOfString var log = new List<String>(); ILogger mslogger= new LoggerFactory().AddStringListLogger(log).CreateLogger("Test2"); // Serilog.Sinks.ListOfString Serilog.Logger slogger= new LoggerConfiguration().WriteTo.StringList(log).CreateLogger(); ```
TestBase gives you a flying start with
UnitUnderTest.Action()
.ShouldNotBeNull()
.ShouldEqualByValueExceptFor(new {Id=1, Descr=expected}, ignoreList )
.Payload
.ShouldMatchIgnoringCase("I expected this")
.Should(someOtherPredicate);
.Items
.ShouldAll(predicate)
.ShouldContain(item)
.ShouldNotContain(predicate)
.Where(predicate)
.SingleOrAssertFail()
# works with all kinds of object and collections, and report what differed.
objectOrCollection
.ShouldEqualByValue().ShouldEqualByValueExceptFor(...).ShouldEqualByValueOnMembers()
string
.ShouldMatch(pattern).ShouldNotMatch().ShouldBeEmpty().ShouldNotBeEmpty()
.ShouldNotBeNullOrEmptyOrWhiteSpace().ShouldEqualIgnoringCase()
.ShouldContain().ShouldStartWith().ShouldEndWith().ShouldBeContainedIn(), ...
numeric
.ShouldBeBetween().ShouldEqualWithTolerance()....GreaterThan....LessThan...GreaterOrEqualTo ...
ienumerable
.ShouldAll().ShouldContain().ShouldNotContain().ShouldBeEmpty().ShouldNotBeEmpty() ...
stream
.ShouldHaveSameStreamContentAs().ShouldContain()
value
.ShouldBe().ShouldNotBe().ShouldBeOfType().ShouldBeAssignableTo()...
TestBase.HttpClient.Fake
new FakeHttpClient()
.Setup(x=>x.RequestUri.PathAndQuery.StartsWith("/this"))
.Returns(response)
.Setup(x=>x.Method==HttpMethod.Put)
.Returns(new HttpResponseMessage(HttpStatusCode.Accepted));
FakeDbConnection
- db.SetupForQuery(…)
- db.SetupForExecuteNonQuery(…)
- db.ShouldHaveUpdated("tableName", …)
- db.ShouldHaveSelected("tableName", …)
- db.ShouldHaveDeleted("tableName", …)
- db.Verify( x=>x.CommandText.Matches("Insert [case] .*")
&& x.Parameters["id"].Value==1 )
- db
.ShouldHaveInvoked(cmd => predicate(cmd))
.ShouldHaveParameter("name", value)
RecordingDbConnection
ControllerUnderTest.WithControllerContext()
.Action()
.ShouldbeViewResult()
.ShouldHaveModel<TModel>()
.ShouldEqualByValue(expected)
ControllerUnderTest.Action()
.ShouldBeRedirectToRouteResult()
.ShouldHaveRouteValue("expectedKey", [Optional] "expectedValue");
ShouldHaveViewDataContaining(), ShouldBeJsonResult() etc.
controllerUnderTest.WithControllerContext(actionUnderTest)
HostedMvcTestFixtureBase
and specify your MVCApplications Startup
class.[TestCase("/dummy")]
public async Task Put_Should_ReturnA(string url)
{
var httpClient=GivenClientForRunningServer<Startup>();
GivenRequestHeaders(httpClient, "CustomHeader", "HeaderValue1");
var result = await httpClient.PutAsync(url, json);
result.ShouldBe_202Accepted();
}
For Mvc4 and Mvc 5, fake your http request & context, and use the RegisterRoutes
method
of your actual application to set up Controller.Url
ControllerUnderTest
.WithHttpContextAndRoutes(
RouteConfig.RegisterRoutes,
"/incomingurl"
);
ApiControllerUnderTest.WithWebApiHttpContext<T>(
httpMethod,
requestUri,
routeTemplate)
Testable Logging
// Extensions.Logging.ListOfString
var log = new List<String>();
ILogger mslogger= new LoggerFactory().AddStringListLogger(log).CreateLogger("Test2");
// Serilog.Sinks.ListOfString
Serilog.Logger slogger= new LoggerConfiguration().WriteTo.StringList(log).CreateLogger();
### Release Notes
6.0.0 Better Assertion output for core asserts includes ActualValue,ActualExpression,Asserted,AssertedDetail
FAQs
*TestBase* gives you a flying start with - fluent assertions that are easy to extend - sharp error messages - tools to help you test with “heavyweight” dependencies on - AspNetCore.Mvc, AspNet.Mvc or WebApi Contexts - HttpClient - Ado.Net - Streams & Logging - Mix & match with your favourite test runners & assertions. ``` UnitUnderTest.Action() .ShouldNotBeNull() .ShouldEqualByValueExceptFor(new {Id=1, Descr=expected}, ignoreList ) .Payload .ShouldMatchIgnoringCase("I expected this") .Should(someOtherPredicate); .Items .ShouldAll(predicate) .ShouldContain(item) .ShouldNotContain(predicate) .Where(predicate) .SingleOrAssertFail() .ShouldEqualByValue().ShouldEqualByValueExceptFor(...).ShouldEqualByValueOnMembers() work with all kinds of object and collections, and report what differed. string.ShouldMatch(pattern).ShouldNotMatch().ShouldBeEmpty().ShouldNotBeEmpty() .ShouldNotBeNullOrEmptyOrWhiteSpace().ShouldEqualIgnoringCase() .ShouldContain().ShouldStartWith().ShouldEndWith().ShouldBeContainedIn(), ... numeric.ShouldBeBetween().ShouldEqualWithTolerance()....GreaterThan....LessThan...GreaterOrEqualTo ... ienumerable.ShouldAll().ShouldContain().ShouldNotContain().ShouldBeEmpty().ShouldNotBeEmpty() ... stream.ShouldHaveSameStreamContentAs().ShouldContain() value.ShouldBe().ShouldNotBe().ShouldBeOfType().ShouldBeAssignableTo()... ``` Testable Logging is in packages Extensions.Logging.ListOfString and Serilog.Sinks.ListOfString ``` // Extensions.Logging.ListOfString var log = new List<String>(); ILogger mslogger= new LoggerFactory().AddStringListLogger(log).CreateLogger("Test2"); // Serilog.Sinks.ListOfString Serilog.Logger slogger= new LoggerConfiguration().WriteTo.StringList(log).CreateLogger(); ```
We found that testbase demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
A deceptive PyPI package posing as an Instagram growth tool collects user credentials and sends them to third-party bot services.
Product
Socket now supports pylock.toml, enabling secure, reproducible Python builds with advanced scanning and full alignment with PEP 751's new standard.
Security News
Research
Socket uncovered two npm packages that register hidden HTTP endpoints to delete all files on command.