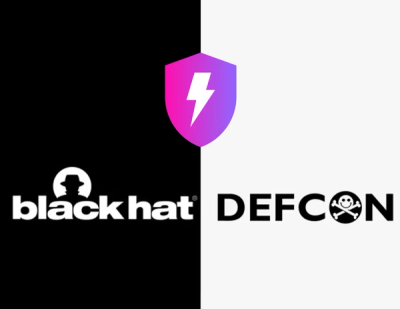
Security News
Meet Socket at Black Hat and DEF CON 2025 in Las Vegas
Meet Socket at Black Hat & DEF CON 2025 for 1:1s, insider security talks at Allegiant Stadium, and a private dinner with top minds in software supply chain security.
ComplexPy is a Python library for storing and doing arithmetic with complex numbers.
This library allows you to perform elementary, trigonometric, logarithmic, and comparative operations with complex numbers.
- toString
- add
/ sum
- sub
/ subtract
/ difference
- mul
/ multiply
/ product
/ scale
- div
/ divide
/ quotient
- pow
/ exponentiate
- sqrt
/ square_root
- nroot
/ nth_root
- abs
/ absolute_value
/ mod
/ modulus
- conj
/ conjugate
- arg
/ argument
- ln
/ natural_log
/ natural_logarithm
- log
/ logarithm
/ general_log
- eq
/ equals
- le
/ less
/ less_than
- gr
/ greater
/ greater_than
- leeq
/ less_than_or_equals
- greq
/ greater_than_or_equals
- sin
/ sine
- cos
/ cosine
- tan
/ tangent
from ComplexPy import *
number = complexNumber(3, 7)
number2 = complexNumber(8, 4)
print(number.toString()) # Will print "3 + 7 i"
print(number.add(number2).toString()) # Will print "11 + 11 i"
print(number.pow(complexNumber(3, 0)).toString()) # Will print "-414.00000000000006 - 154.0 i"
print(number.sine().toString()) # Will print "77.37850442046603 - 542.8288478055589 i"
print(number.sqrt().toString()) # Will print "2.3038850997677716 + 1.5191729832155236 i"
print(number.sqrt(1).toString()) # Will print "-2.3038850997677716 - 1.5191729832155234 i"
You can install this library by running the command below in a command prompt or powershell terminal.
pip install ComplexPy
- Initial release.
FAQs
A Python library for handling calculations of complex number.
We found that ComplexPy demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Meet Socket at Black Hat & DEF CON 2025 for 1:1s, insider security talks at Allegiant Stadium, and a private dinner with top minds in software supply chain security.
Security News
CAI is a new open source AI framework that automates penetration testing tasks like scanning and exploitation up to 3,600× faster than humans.
Security News
Deno 2.4 brings back bundling, improves dependency updates and telemetry, and makes the runtime more practical for real-world JavaScript projects.