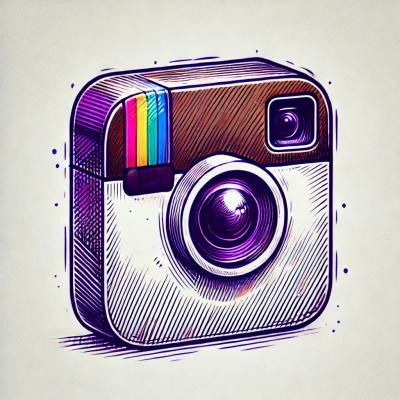
Research
PyPI Package Disguised as Instagram Growth Tool Harvests User Credentials
A deceptive PyPI package posing as an Instagram growth tool collects user credentials and sends them to third-party bot services.
A custom Qtableview that adds many features for tables, including using SQL, filters, sub-tables, footers, checkboxes and more..
This is based on a table UI element from an expensive piece of software from my work that is extremely handy for a manufacturing environment for tracking work/showing data. I thought I'd recreate it.
My implementation of this will remain performant even with tens of thousands of rows of data.
Features:
setup_table arguments (NOTE: these arguments do NOT need to be passed in at time of table initialization. The parameters/argument can be changed with separate functions later.
ExtentedQtableview.setup_table(maintable_data, maintable_headers, columns_with_checkboxes, checked_indexes_rows, sub_table_data, editable_columns, datetime_columns, footer, footer_values, subtable_col_checkboxes, sub_table_headers_labels, expandable_rows, add_mainrow_option, del_mainrow_option, add_subrow_option, del_subrow_option, subtable_datetime_columns, dbleclick_edit_only, use_sql, sql_maintable_path, sql_maintable_name, sql_maintable_query, sql_subtable_path, sql_subtable_name, sql_subtable_query)
Accepts list of strings representing each row of table, IE: [[row1, row1, row1], [row2, row2, row2]]
Accepts list of strings for header labels, IE: [label1, label2, label3]
Accepts list of integers for columns you want to have checkboxes, IE: [1, 2, 5]
Accepts a dictionary representing which rows you want to have checked for the columns with checkboxes, key = column number, values = row numbers IE: {1: [4, 5, 6], 2: [1, 2, 6], 5: [1, 3, 7]}
Accepts a list of strings representing each row of the subtable for EACH row of the maintable, IE: [ [[1sub1, 1sub1, 1sub1], [1sub2, 1sub2 1sub2]], [[2sub1, 2sub1, 2sub1], [2sub2, 2sub2, 2sub2]] ] If using subtables, this argument MUST be equal to the number of rows on the main table, even if the list is blank such as [].
For Example if your maintable has 3 rows and you are using expandable rows, then at minimum you need to pass [[], [], []] into this parameter
Accepts list of integers representing which columns you want to be editable, IE: [1, 2, 5]
Accepts list of integers representing which columns you want to use datetime and have a calendar popup, IE: [1, 2, 5]
Accepts True or False bool to enable/disable footer
Accepts a dictionary for which columns you want to have a footer, keys are columns indexes and values can be "total" or "sum", IE: {1: "sum", 2: "total"} "sum" = will sum integers/float values in the column together "total" = will add up total columns or total boxes checked in column if the column is a checkbox column Note: These values on the table will change dynamically based on any rows filtered
Accepts list of integers representing which columns in sub-tables to have checkboxes, IE: [0, 1, 3]
Accepts list of strings representing header labels for sub-tables, IE: [header1, header2, header3]
Accepts True or False bool. This MUST be enabled to use sub-tables!
Accepts True or False bool to enable option to add rows to main table
Accepts True or False bool to enable option for deletion of rows to main table
Accepts True or False bool to enable option to add rows to sub-tables
Accepts True or False bool to enable option for deletion of rows to sub-tables
Accepts list of integers representing which columns in the sub-tables you want to be datetime with calendar popups, IE: [1, 5, 7]
Accepts True or False bool to enable editing for the main table on a separate pop-up rather than on the table itself.
(useful to prevent accidental changes to the main table.
Accepts True or False to enable the use of SQL tables. This MUST be set to True to use SQL tables
Accepts string representing full path to the SQL database file for the main table data
Accepts string representing name of SQL table to grab from the SQL database file
Accepts query to do a specific SQL query to grab specific data from the SQL table. NOTE: THIS HAS NOT BEEN TESTED
Accepts string representing full path to SQL database file for the sub-table data
Accepts string representing SQL table name from the SQL database file listed in the sql_subtable_path argument
Accepts query to do a specific SQL query to grab specific data from the sub-table SQL table. NOTE: THIS HAS NOT BEEN TESTED
If you've already setup your table instance, with say the following:
import ExtendedQtableview table = ExtendedQtableview.setup_table()
table.loadnew_maintable_sql(maintable_name, maintable_sql_path, maintable_query, subtable_sql_name, subtable_sql_path, subtable_headers, subtable_query, keep_existing_filter)
Name of SQL table to get from database
Directory path to SQL database file
SQL query to pass to get specific data from table NOTE: This has NOT been tested
Name of SQL sub table to get from database
Directory path to SQL database file
List of column header names for the sub tables
SQL query to pass to get specific data from table NOTE: This has NOT been tested
Accepts list of strings representing each row of table, IE: [[row1, row1, row1], [row2, row2, row2]]
Whether you want to keep any existing filters applied to the current table or not. This is useful if you want the new table to be filtered from the get go.
Accepts a list of strings representing each row of the subtable for EACH row of the maintable, IE: [ [[1sub1, 1sub1, 1sub1], [1sub2, 1sub2 1sub2]], [[2sub1, 2sub1, 2sub1], [2sub2, 2sub2, 2sub2]] ] If using subtables, this argument MUST be equal to the number of rows on the main table, even if the list is blank such as [].
For Example if your maintable has 3 rows and you are using expandable rows, then at minimum you need to pass [[], [], []] into this parameter
Table name listed in the SQL database
Directory path to SQL database file
Accepts list of strings representing each name of the header columns
Query to run on SQL database to get specific data from the table. NOTE: This has not been tested
Accepts a dictionary representing which rows you want to have checked for the columns with checkboxes, key = column number, values = row numbers IE: {1: [4, 5, 6], 2: [1, 2, 6], 5: [1, 3, 7]}
Change columns you want designated on the main table to be checkbox columns
Change which columns you want to be user editable
Change which columns you want to be date time. If columns are editable, will provide a calendar popup to let user change dates.
Accepts a dictionary for which columns you want to have a footer, keys are columns indexes and values can be "total" or "sum", IE: {1: "sum", 2: "total"} "sum" = will sum integers/float values in the column together "total" = will add up total columns or total boxes checked in column if the column is a checkbox column Note: These values on the table will change dynamically based on any rows filtered
Change column headers for the sub-tables
Change which column(s) in the sub tables you want to be checkbox columns
Change if you want to allow user to add rows to main table
Change if you want to allow user to delete rows from main table
Change if you want to allow user to add rows to the sub-tables
Change if you want to allow user to delete rows from the sub-tables
Change which column(s) you want the sub-table to be datetime, which will allow user to update date via calendar popup.
Enable to allow editing of the main table through a separate screen rather than on the table itself. Useful to prevent accidental changes.
Enable/Disable using a footer
Change the column headers on the main table
Change on whether to use SQL tables. Obviously must be enabled to pull data from SQL database and use tables
Enable/Disable using expandable rows with sub-tables
Function clears table and pass in whether you want to retain the existing filter or not when loading in new table
If you've already setup your table instance, with say the following:
import ExtendedQtableview table = ExtendedQtableview.setup_table()
To use existing QTableView functions, just do table.table_view.(existing qtableview function)()
For example:
table.table_view.resizeColumnsToContents()
maintable_index column1 column2 1 data1 data2 1 data3 something1 2 test1 test2 2 data52 data0
createEditor() (for creating the button) setEditorData() (for setting the data to be in the editor) updateEditorGeometry() (for setting size of editor within the cell)And you may or may not want to send a signal to the handleDateeditKeyPress() function if you make a change in the editor and want to forcibly close it and commit the data say on the index change of a QCombobox
FAQs
A custom Qtableview that adds many features for tables, including using SQL, filters, sub-tables, footers, checkboxes and more..
We found that ExtendedQtableview demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
A deceptive PyPI package posing as an Instagram growth tool collects user credentials and sends them to third-party bot services.
Product
Socket now supports pylock.toml, enabling secure, reproducible Python builds with advanced scanning and full alignment with PEP 751's new standard.
Security News
Research
Socket uncovered two npm packages that register hidden HTTP endpoints to delete all files on command.