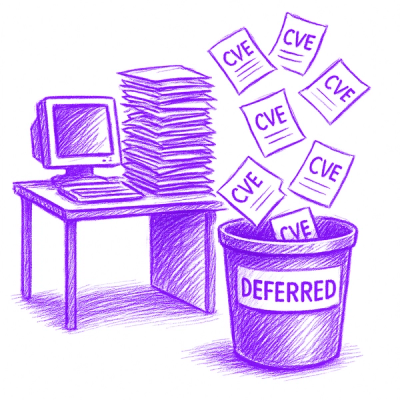
Security News
NVD Quietly Sweeps 100K+ CVEs Into a “Deferred” Black Hole
NVD now marks all pre-2018 CVEs as "Deferred," signaling it will no longer enrich older vulnerabilities, further eroding trust in its data.
The `FileMa` (File Management) library provides utilities for file and directory management in Python. It includes functions for creating, removing, renaming, copying files and directories, as well as reading file contents, printing directory structures, and more. The library uses colored terminal output for better visual feedback.
The FileMa
library provides utilities for file and directory management in Python. It includes functions for creating, removing, renaming, copying files and directories, as well as reading file contents, printing directory structures, and more. The library uses colored terminal output for better visual feedback.
Ensure that you have the colorama
library installed. You can install it via pip if it's not already installed:
pip install colorama
pip install FileMa
Below are the available functions and how to use them.
File Creation & Deletion
touch(file_name)
: Create a new file or update the access time if it already exists.rm(file_name)
: Remove a file if it exists.File Reading & Writing
nano(file_name, mode, content)
: Write or append content to a file.cat(file_name)
: Read and display the contents of a file.File Operations
ren(name, new_name)
: Rename a file or directory.mv(source_path, destination_path)
: Move a file or directory to a new location.copy(file_name, new_file, mode)
: Copy contents from one file to another with the specified mode.copyf(source_path, destination_path)
: Copy a file from the source to the destination path.Directory Management
mkdir(folder_name)
: Create a new directory if it doesn't already exist.rmdir(folder_name)
: Remove an empty directory.mkdirs(folder_name)
: Create directories, including intermediate ones.rmdirs(directory_path)
: Remove directories recursively.copyd(source_path, destination_path)
: Copy an entire directory with its contents.Directory Navigation & Listing
cd(path)
: Change the current working directory.tree(path, prefix="")
: Recursively print the directory tree structure.pwd()
: Print the current working directory.ls(path)
: List the contents of a directory, identifying files and directories.ls_l(path)
: List the contents of a directory with detailed information (permissions, last modified time, size).File Metadata
istime(path_name)
: Print the last access time of a file.size(path_name)
: Print the size of a file.System Information
get_info()
: Print environment variables and their values.get_path()
: Print executable paths.These functions offer a robust set of tools for file management operations, and they include error handling for common issues like file not found or permission errors.
import FileMa
Here is the usage guide for the FileMa
library functions :
FileMa.touch('example.txt')
Description: Creates a new file named example.txt
if it does not already exist. If it does exist, it updates the access time.
FileMa.rm('example.txt')
Description: Removes the file named example.txt
if it exists.
FileMa.nano('example.txt', 'w', 'Hello, World!')
Description: Writes 'Hello, World!' to example.txt
. Use 'a'
instead of 'w'
to append the content instead of overwriting it.
FileMa.cat('example.txt')
Description: Displays the contents of example.txt
.
FileMa.ren('old_name.txt', 'new_name.txt')
Description: Renames old_name.txt
to new_name.txt
.
FileMa.mv('source_path.txt', 'destination_path.txt')
Description: Moves source_path.txt
to destination_path.txt
.
FileMa.copy('source.txt', 'destination.txt', 'w')
Description: Copies the content of source.txt
to destination.txt
. You can use 'a'
instead of 'w'
to append the content.
FileMa.copyf('source.txt', 'destination.txt')
Description: Copies the file source.txt
to destination.txt
.
FileMa.mkdir('new_folder')
Description: Creates a new directory named new_folder
.
FileMa.rmdir('new_folder')
Description: Removes the empty directory named new_folder
.
FileMa.copyd('source_folder', 'destination_folder')
Description: Copies source_folder
and all its contents to destination_folder
.
FileMa.mkdirs('parent_folder/child_folder')
Description: Creates parent_folder
and child_folder
, including any necessary intermediate directories.
FileMa.rmdirs('folder_to_remove')
Description: Removes folder_to_remove
and all its contents.
FileMa.cd('path_to_directory')
Description: Changes the current working directory to path_to_directory
.
FileMa.tree('path_to_directory')
Description: Prints the directory structure starting from path_to_directory
in a hierarchical format.
FileMa.pwd()
Description: Prints the current working directory.
FileMa.ls('path_to_directory')
Description: Lists the contents of path_to_directory
, indicating whether each item is a file or a directory.
FileMa.ls_l('path_to_directory')
Description: Lists the contents of path_to_directory
with detailed information, such as permissions, last modification time, and size.
FileMa.istime('file_name')
Description: Prints the last access time of the file file_name
.
FileMa.size('file_name')
Description: Prints the size of the file file_name
in bytes.
FileMa.get_info()
Description: Prints all environment variables and their values.
FileMa.get_path()
Description: Prints the paths where executables are searched for.
You can use these functions in your scripts to perform various file and directory operations effectively.
FAQs
The `FileMa` (File Management) library provides utilities for file and directory management in Python. It includes functions for creating, removing, renaming, copying files and directories, as well as reading file contents, printing directory structures, and more. The library uses colored terminal output for better visual feedback.
We found that FileMa demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
NVD now marks all pre-2018 CVEs as "Deferred," signaling it will no longer enrich older vulnerabilities, further eroding trust in its data.
Research
Security News
Lazarus-linked threat actors expand their npm malware campaign with new RAT loaders, hex obfuscation, and over 5,600 downloads across 11 packages.
Security News
Safari 18.4 adds support for Iterator Helpers and two other TC39 JavaScript features, bringing full cross-browser coverage to key parts of the ECMAScript spec.