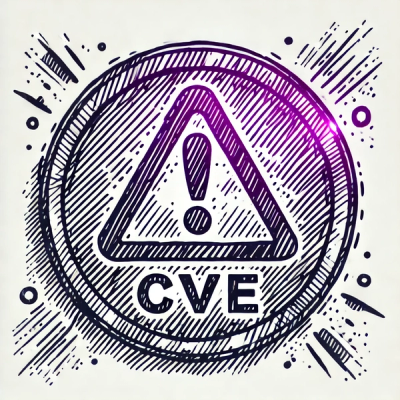
Security News
CISA Extends MITRE Contract as Crisis Accelerates Alternative CVE Coordination Efforts
CISA extended MITRE’s CVE contract by 11 months, avoiding a shutdown but leaving long-term governance and coordination issues unresolved.
FBGraphHandler is a Python library that simplifies the process of interacting with Facebook's Graph API. It provides methods for authenticating users, retrieving user details, posting text and video feeds, and more.
You can install FBGraphHandler using pip:
pip install FBGraphHandler
from FBGraphHandler import FBGraphHandler
# Create an instance of FBGraphHandler
graph_handler = FBGraphHandler()
# Configure FBGraphHandler with your Facebook App credentials
graph_handler.Config(client_id="YOUR_CLIENT_ID", client_secret="YOUR_CLIENT_SECRET", version="14.0")
# Generate an authorization URL for users to complete the authentication process
auth_url = graph_handler.GenerateAuthURL(redirect_uri="YOUR_REDIRECT_URI", scopes=["public_profile", "email"])
# Exchange the authorization code for an access token
access_token = graph_handler.ExchangeCodewithToken(code="AUTHORIZATION_CODE")
# Get user details using the access token
user_details = graph_handler.GetUserDetails(access_token="ACCESS_TOKEN")
# Post a text feed to a user or page
post_id = graph_handler.PostTextFeed(text="Hello, Facebook!", id="PAGE_OR_USER_ID", access_token="ACCESS_TOKEN")
# Post a video feed to a user or page
post_id = graph_handler.PostVideoFeed(text="Check out this video!", id="PAGE_OR_USER_ID", access_token="ACCESS_TOKEN", video_url="VIDEO_URL")
# Post text, photos, or videos to a user or page
media_urls = ["PHOTO_URL_1", "PHOTO_URL_2"]
post_id = graph_handler.PostToFacebook(text="My Facebook post", media_urls=media_urls, access_token="ACCESS_TOKEN", id="PAGE_OR_USER_ID", isVideo=False)
For detailed documentation and more information about available methods and parameters, please refer to the official documentation.
This project is licensed under the MIT License - see the LICENSE file for details.
FAQs
Unofficial Facebook Graph API Handler
We found that GraphHandler demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
CISA extended MITRE’s CVE contract by 11 months, avoiding a shutdown but leaving long-term governance and coordination issues unresolved.
Product
Socket's Rubygems ecosystem support is moving from beta to GA, featuring enhanced security scanning to detect supply chain threats beyond traditional CVEs in your Ruby dependencies.
Research
The Socket Research Team investigates a malicious npm package that appears to be an Advcash integration but triggers a reverse shell during payment success, targeting servers handling transactions.