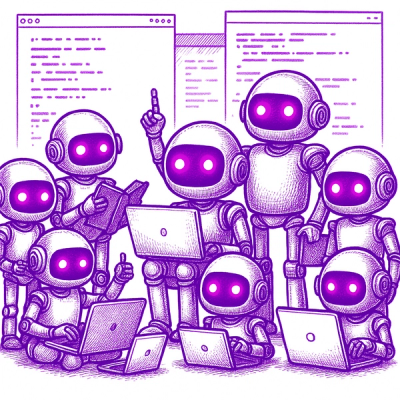
Security News
Open Source CAI Framework Handles Pen Testing Tasks up to 3,600× Faster Than Humans
CAI is a new open source AI framework that automates penetration testing tasks like scanning and exploitation up to 3,600× faster than humans.
Sentiscope is a powerful Python package for analyzing sentiment and extracting entities from financial news articles. It leverages multiple state-of-the-art sentiment analysis models and named entity recognition techniques to provide comprehensive insights into financial news.
pip install sentiscope
from sentiscope import SentimentAnalyzer, NewsFetcher, EntityExtractor
# Initialize components
sentiment_analyzer = SentimentAnalyzer()
news_fetcher = NewsFetcher(api_key="your_newsapi_key_here")
entity_extractor = EntityExtractor()
# Fetch news
news = news_fetcher.fetch_financial_news("Banking")
# Analyze sentiment and extract entities
for article in news:
finbert_sentiment = sentiment_analyzer.analyze_sentiment_finbert(article['description'])
vader_sentiment = sentiment_analyzer.analyze_sentiment_vader(article['description'])
entities = entity_extractor.extract_entities_flair(article['description'])
print(f"Title: {article['title']}")
print(f"FinBERT Sentiment: {finbert_sentiment}")
print(f"VADER Sentiment: {vader_sentiment}")
print(f"Entities: {entities}")
print("---")
Sentiscope offers multiple sentiment analysis models:
text = "The company reported strong earnings, beating market expectations."
finbert_sentiment = sentiment_analyzer.analyze_sentiment_finbert(text)
vader_sentiment = sentiment_analyzer.analyze_sentiment_vader(text)
esgbert_sentiment = sentiment_analyzer.analyze_sentiment_esgbert(text)
finbert_tone_sentiment = sentiment_analyzer.analyze_sentiment_finbert_tone(text)
flair_sentiment = sentiment_analyzer.analyze_sentiment_flair(text)
Fetch financial news for specific sectors:
news = news_fetcher.fetch_financial_news("Technology")
Sentiscope uses the following pre-trained models:
This package requires an API key from NewsAPI to fetch financial news articles. You can obtain a key by registering at https://newsapi.org.
This project is licensed under the MIT License.
Contributions are welcome! Please feel free to submit a Pull Request.
If you encounter any issues or have questions, please file an issue on the GitHub repository.
Sentiscope is built on top of several open-source projects and pre-trained models. We are grateful to the developers and researchers who have made their work available to the community.
FAQs
A package for financial news sentiment analysis and entity extraction
We found that SENTISCOPE demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
CAI is a new open source AI framework that automates penetration testing tasks like scanning and exploitation up to 3,600× faster than humans.
Security News
Deno 2.4 brings back bundling, improves dependency updates and telemetry, and makes the runtime more practical for real-world JavaScript projects.
Security News
CVEForecast.org uses machine learning to project a record-breaking surge in vulnerability disclosures in 2025.