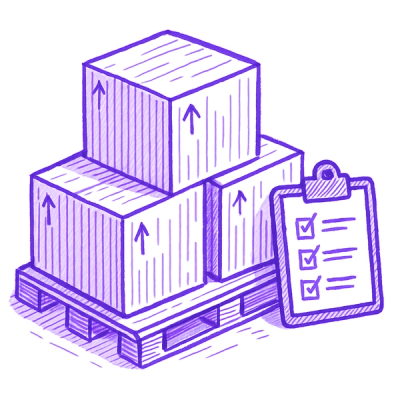
Security News
Open Source Maintainers Feeling the Weight of the EU’s Cyber Resilience Act
The EU Cyber Resilience Act is prompting compliance requests that open source maintainers may not be obligated or equipped to handle.
The Job Scheduler is a Python library designed for scheduling and managing recurring tasks (jobs). This library allows you to easily set up jobs that can run at specific intervals (e.g., every minute, every hour, etc.), on specific days, or until a certain time, with support for repetition. Threading is also supported and optional for non-blocking execution.
To install the Job Scheduler, run the following command:
pip install Timeline-Manager
The Job Scheduler provides two main methods for scheduling tasks: the Standard Method and the Decorator Method. Below are examples of how to use both methods.
from Timeline_Manager import JobScheduler, Job
# Initialize the scheduler
scheduler = JobScheduler()
# Define a standard task function
def my_task():
print("Task executed!")
# Create a job using the standard method
job = Job().every(1).minute.repeat(3).do(my_task)
# Add the job to the scheduler
scheduler.add_job(job)
# Start the scheduler
scheduler.run_pending()
from Timeline_Manager import JobScheduler, jobs
# Initialize the scheduler
scheduler = JobScheduler()
# Define a sample job function using the decorator method
@jobs(interval=1, unit='minute', repeat=3, scheduler=scheduler)
def my_task():
print("Task executed!")
# Start the scheduler
scheduler.run_pending()
In both examples:
The Job
class provides a variety of options for scheduling jobs. These options can be chained together to create complex scheduling scenarios.
The interval
and unit
methods define how often the job should run. Supported units include:
second
minute
hour
day
week
month
year
job.every(5).minute.do(my_task)
This will schedule the job to run every 5 minutes.
every()
for Intervals Greater Than 1Standard Method:
from Timeline_Manager import JobScheduler, Job
# Initialize the scheduler
scheduler = JobScheduler()
# Define the task function
def task_every_2_hours():
print("Task executed every 2 hours!")
# Create a job to run every 2 hours
job = Job().every(2).hour.do(task_every_2_hours)
# Add the job to the scheduler
scheduler.add_job(job)
# Start the scheduler
scheduler.run_pending()
Decorator Method:
from Timeline_Manager import JobScheduler, jobs
# Initialize the scheduler
scheduler = JobScheduler()
@jobs(interval=2, unit='hour', scheduler=scheduler)
def task_every_2_hours():
print("Task executed every 2 hours!")
# Start the scheduler
scheduler.run_pending()
In this example:
Standard Method:
from Timeline_Manager import JobScheduler, Job
# Initialize the scheduler
scheduler = JobScheduler()
# Define the task function
def task_every_3_days():
print("Task executed every 3 days!")
# Create a job to run every 3 days
job = Job().every(3).day.do(task_every_3_days)
# Add the job to the scheduler
scheduler.add_job(job)
# Start the scheduler
scheduler.run_pending()
Decorator Method:
from Timeline_Manager import JobScheduler, jobs
# Initialize the scheduler
scheduler = JobScheduler()
@jobs(interval=3, unit='day', scheduler=scheduler)
def task_every_3_days():
print("Task executed every 3 days!")
# Start the scheduler
scheduler.run_pending()
In this example:
Standard Method:
from Timeline_Manager import JobScheduler, Job
# Initialize the scheduler
scheduler = JobScheduler()
# Define the task function
def task_every_2_weeks():
print("Task executed every 2 weeks!")
# Create a job to run every 2 weeks on Monday
job = Job().every(2).week(weekday=0).do(task_every_2_weeks)
# Add the job to the scheduler
scheduler.add_job(job)
# Start the scheduler
scheduler.run_pending()
Decorator Method:
from Timeline_Manager import JobScheduler, jobs
# Initialize the scheduler
scheduler = JobScheduler()
@jobs(interval=2, unit='week', scheduler=scheduler)
def task_every_2_weeks():
print("Task executed every 2 weeks!")
# Start the scheduler
scheduler.run_pending()
In this example:
Standard Method:
from Timeline_Manager import JobScheduler, Job
# Initialize the scheduler
scheduler = JobScheduler()
# Define the task function
def task_every_3_months():
print("Task executed every 3 months!")
# Create a job to run every 3 months on the 15th at 9:00 AM
job = Job().every(3).month(day=15, time_at="09:00").do(task_every_3_months)
# Add the job to the scheduler
scheduler.add_job(job)
# Start the scheduler
scheduler.run_pending()
Decorator Method:
from Timeline_Manager import JobScheduler, jobs
# Initialize the scheduler
scheduler = JobScheduler()
@jobs(interval=3, unit='month', time_at="09:00", scheduler=scheduler)
def task_every_3_months():
print("Task executed every 3 months!")
# Start the scheduler
scheduler.run_pending()
In this example:
Standard Method:
from Timeline_Manager import JobScheduler, Job
# Initialize the scheduler
scheduler = JobScheduler()
# Define the task function
def task_every_2_years():
print("Task executed every 2 years!")
# Create a job to run every 2 years on January 1st at midnight
job = Job().every(2).year(month=1, day=1, time_at="00:00").do(task_every_2_years)
# Add the job to the scheduler
scheduler.add_job(job)
# Start the scheduler
scheduler.run_pending()
Decorator Method:
from Timeline_Manager import JobScheduler, jobs
# Initialize the scheduler
scheduler = JobScheduler()
@jobs(interval=2, unit='year', time_at="00:00", scheduler=scheduler)
def task_every_2_years():
print("Task executed every 2 years!")
# Start the scheduler
scheduler.run_pending()
In this example:
The Job Scheduler allows you to schedule multiple jobs that can run at the same time. For example, you might want to run two different tasks every day at the same time.
from Timeline_Manager import JobScheduler, Job
# Initialize the scheduler
scheduler = JobScheduler()
# Define task functions
def task1():
print("Task 1 executed!")
def task2():
print("Task 2 executed!")
# Create jobs that run at the same time
job1 = Job().every(1).day.at("08:00").do(task1)
job2 = Job().every(1).day.at("08:00").do(task2)
# Add jobs to the scheduler
scheduler.add_job(job1)
scheduler.add_job(job2)
# Start the scheduler
scheduler.run_pending()
from Timeline_Manager import JobScheduler, jobs
# Initialize the scheduler
scheduler = JobScheduler()
@jobs(interval=1, unit='day', time_at="08:00", scheduler=scheduler)
def task1():
print("Task 1 executed!")
@jobs(interval=1, unit='day', time_at="08:00", scheduler=scheduler)
def task2():
print("Task 2 executed!")
# Start the scheduler
scheduler.run_pending()
In this example:
task1
and task2
are scheduled to run at 8:00 AM every day.When scheduling jobs, you can specify specific weekdays, days of the month, and months. Below are the values for each:
Weekday Values:
Day Values:
Month Values:
To run jobs in separate threads (useful for non-blocking execution), set use_threading=True
when initializing the JobScheduler
.
scheduler = JobScheduler(use_threading=True)
In this example:
The JobScheduler
class manages the execution of all scheduled jobs. You can add jobs using the add_job
method and start the scheduler using the run_pending
method. The scheduler will automatically remove completed jobs.
To start running the scheduled jobs, call scheduler.run_pending()
in your main program. This will loop through all jobs and run them if they are scheduled to run.
scheduler.run_pending()
If you pass unsupported units or incorrect time formats, the scheduler will raise appropriate exceptions to help you debug.
This project is licensed under the MIT License. Feel free to use, modify, and distribute it.
If you find any issues or have suggestions for improvements, feel free to submit a pull request or open an issue on the project's repository.
For further questions or support, please contact the project maintainer.
FAQs
A job scheduling library that supports various time intervals
We found that Timeline-Manager demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
The EU Cyber Resilience Act is prompting compliance requests that open source maintainers may not be obligated or equipped to handle.
Security News
Crates.io adds Trusted Publishing support, enabling secure GitHub Actions-based crate releases without long-lived API tokens.
Research
/Security News
Undocumented protestware found in 28 npm packages disrupts UI for Russian-language users visiting Russian and Belarusian domains.