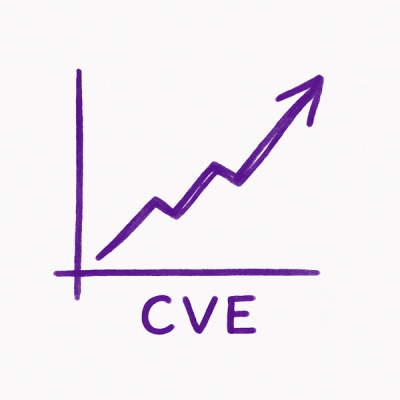
Security News
New CVE Forecasting Tool Predicts 47,000 Disclosures in 2025
CVEForecast.org uses machine learning to project a record-breaking surge in vulnerability disclosures in 2025.
Abnormal expressions (abnex) is an alternative to regular expressions (regex). This is a Python library but the abnex syntax could be ported to other languages.
Regex
([\w\._-]+)@([\w\.]+)
Abnex
{[w"._-"]1++}"@"{[w"."]1++}
Abnex (spaced)
{[w "._-"]1++} "@" {[w "."]1++}
Abnex (expanded)
{
[w "._-"]1++
}
"@"
{
[w "."]1++
}
{{{[a-z '_']1++} {[a-z 0-9 '_-.']0++}} '@' {{[a-z 0-9]1++} '.' {[a-z 0-9]1++} {[a-z 0-9 '-_.']0++}} {[a-z 0-9]1++}}
.
!
for not, {}
for groups, 1++
, 0++
, 0+
for: one or more, zero or more, zero or one.[\w-]+@[\w-_]+
[w "-"]1++ "@" [w "-"]1++
^
-> ->
$
-> <-
\A
-> s>
\Z
-> <s
\b
-> :
\B
-> !:
\<
-> w>
\>
-> <w
\c
-> c
\s
-> _
\S
-> !_
\d
-> d
\D
-> !d
\w
-> w
\W
-> !w
\x
-> x
\o
-> o
*
-> 0++
+
-> 1++
?
-> 0+
.
-> *
a|b
-> "a"|"b"
(...)
-> {...}
(?:...)
-> {#...}
[abc]
-> ['abc']
or ["a" "b" "c"]
[^...]
-> [!...]
[a-q]
-> [a-z]
[A-Q]
-> [A-Q]
[0-7]
-> [0-7]
What is the recommended way to write abnexes
[w "_-"]
[w"_-"]
["abc"]
["a" "b" "c"]
, especially incorrect: ["a""b""c"]
{w} "." {w}
{w}"."{w}
Match for an email address:
[\w-\._]+@[\w-\.]+
{[w "-._"]1++} "@" {[w "-."]1++}
{[w"-._"]1++}"@"{[w"-."]1++}
Abnex has most functions from the re
library, but it also has som extra functionality like: last()
& contains()
.
match()
-> match()
findall()
-> all()
split()
-> split()
sub()
-> replace()
subn()
-> replace_count()
search()
-> first()
holds()
: whether or not a string matches an expression (bool).contains()
: wheter or not a string contains a match (bool).last()
: the last match in a string.FAQs
Abnormal expressions (abnex) is an alternative to regular expressions (regex).
We found that abnex demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
CVEForecast.org uses machine learning to project a record-breaking surge in vulnerability disclosures in 2025.
Security News
Browserslist-rs now uses static data to reduce binary size by over 1MB, improving memory use and performance for Rust-based frontend tools.
Research
Security News
Eight new malicious Firefox extensions impersonate games, steal OAuth tokens, hijack sessions, and exploit browser permissions to spy on users.