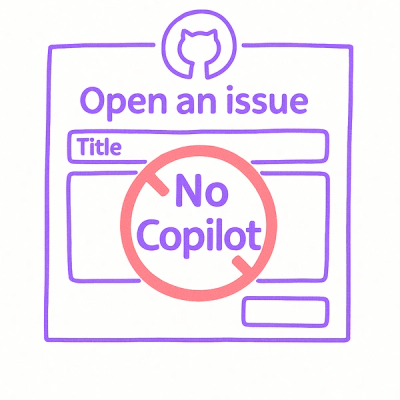
Security News
Open Source Maintainers Demand Ability to Block Copilot-Generated Issues and PRs
Open source maintainers are urging GitHub to let them block Copilot from submitting AI-generated issues and pull requests to their repositories.
The aiOla Streaming SDK provides Python bindings for aiOla's real-time streaming services, enabling real-time audio streaming, transcription, and keyword spotting capabilities.
pip install aiola-stt
from aiola_stt import AiolaSttClient, AiolaSocketConfig, AiolaSocketNamespace
# Create configuration
config = AiolaSocketConfig(
base_url="your-api-key", # i.e https://api.aiola.ai
namespace=AiolaSocketNamespace.EVENTS,
api_key="your-api-key",
query_params={}
)
# Initialize client
client = AiolaSttClient(config)
# Connect and start recording with default built-in microphone
await client.connect(auto_record=True)
# Stop recording
await client.stop_recording()
# Clean up
await client.cleanup_socket()
from aiola_streaming_sdk import AiolaSocketEvents
# Define event handlers
events = AiolaSocketEvents(
on_transcript=lambda data: print(f"Transcript: {data}"),
on_connect=lambda transport: print(f"Connected via {transport}"),
on_disconnect=lambda: print("Disconnected"),
on_error=lambda error: print(f"Error: {error}"),
on_start_record=lambda: print("Recording started"),
on_stop_record=lambda: print("Recording stopped")
)
# Add events to config
config = AiolaSocketConfig(
base_url="your-api-key", # i.e https://api.aiola.ai
namespace=AiolaSocketNamespace.EVENTS,
api_key="your-api-key",
query_params={},
events=events
)
# Set keywords to spot in the audio stream
await client.set_keywords(["hello", "world"])
# Get currently active keywords
active_keywords = client.get_active_keywords()
async def custom_audio_generator():
# Your custom audio streaming logic here
while True:
audio_data = await get_audio_data() # Your audio data source
yield audio_data
# Use custom audio generator
await client.connect(auto_record=True, custom_stream_generator=custom_audio_generator())
from aiola_streaming_sdk import MicConfig
mic_config = MicConfig(
sample_rate=16000, # Hz
chunk_size=4096, # Samples per chunk
channels=1 # Mono audio
)
from aiola_streaming_sdk import VadConfig
vad_config = VadConfig(
vad_threshold=0.5, # VAD threshold (0.0 to 1.0)
min_silence_duration_ms=250 # Minimum silence duration
)
To install development dependencies:
pip install -e ".[dev]"
The SDK provides comprehensive error handling through the AiolaSocketError
class:
from aiola_streaming_sdk import AiolaSocketError, AiolaSocketErrorCode
try:
await client.connect()
except AiolaSocketError as e:
print(f"Error: {e.message}")
print(f"Error code: {e.code}")
print(f"Details: {e.details}")
MIT License
FAQs
Aiola Speech-To-Text Python SDK
We found that aiola-stt demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Open source maintainers are urging GitHub to let them block Copilot from submitting AI-generated issues and pull requests to their repositories.
Research
Security News
Malicious Koishi plugin silently exfiltrates messages with hex strings to a hardcoded QQ account, exposing secrets in chatbots across platforms.
Research
Security News
Malicious PyPI checkers validate stolen emails against TikTok and Instagram APIs, enabling targeted account attacks and dark web credential sales.