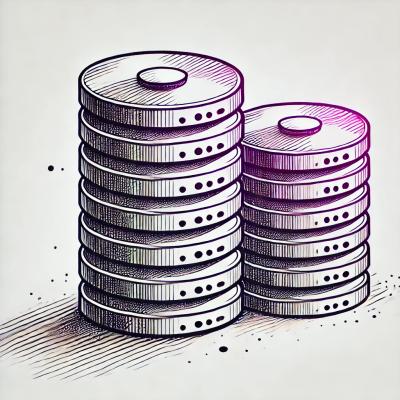
Security News
MCP Community Begins Work on Official MCP Metaregistry
The MCP community is launching an official registry to standardize AI tool discovery and let agents dynamically find and install MCP servers.
Please read UPGRADE-v2.0.md
to learn how to upgrade to Graphene 2.0
.
A SQLAlchemy integration for Graphene.
For instaling graphene, just run this command in your shell
pip install "alchql>=3.0"
Here is a simple SQLAlchemy model:
from sqlalchemy import Column, Integer, String
from sqlalchemy.ext.declarative import declarative_base
Base = declarative_base()
class UserModel(Base):
__tablename__ = 'user'
id = Column(Integer, primary_key=True)
name = Column(String)
last_name = Column(String)
To create a GraphQL schema for it you simply have to write the following:
import graphene
from alchql import SQLAlchemyObjectType
class User(SQLAlchemyObjectType):
class Meta:
model = UserModel
# use `only_fields` to only expose specific fields ie "name"
# only_fields = ("name",)
# use `exclude_fields` to exclude specific fields ie "last_name"
# exclude_fields = ("last_name",)
class Query(graphene.ObjectType):
users = graphene.List(User)
def resolve_users(self, info):
query = await User.get_query(info) # SQLAlchemy query
return query.all()
schema = graphene.Schema(query=Query)
Then you can simply query the schema:
query = '''
query {
users {
name,
lastName
}
}
'''
result = schema.execute(query, context_value={'session': db_session})
You may also subclass SQLAlchemyObjectType by providing abstract = True
in
your subclasses Meta:
from alchql import SQLAlchemyObjectType
import sqlalchemy as sa
import graphene
class ActiveSQLAlchemyObjectType(SQLAlchemyObjectType):
class Meta:
abstract = True
@classmethod
async def get_node(cls, info, id):
return (await cls.get_query(info)).filter(
sa.and_(
cls._meta.model.deleted_at == None,
cls._meta.model.id == id
)
).first()
class User(ActiveSQLAlchemyObjectType):
class Meta:
model = UserModel
class Query(graphene.ObjectType):
users = graphene.List(User)
def resolve_users(self, info):
query = await User.get_query(info) # SQLAlchemy query
return query.all()
schema = graphene.Schema(query=Query)
To learn more check out the following examples:
FAQs
Graphene SQLAlchemy core integration
We found that alchql demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
The MCP community is launching an official registry to standardize AI tool discovery and let agents dynamically find and install MCP servers.
Research
Security News
Socket uncovers an npm Trojan stealing crypto wallets and BullX credentials via obfuscated code and Telegram exfiltration.
Research
Security News
Malicious npm packages posing as developer tools target macOS Cursor IDE users, stealing credentials and modifying files to gain persistent backdoor access.