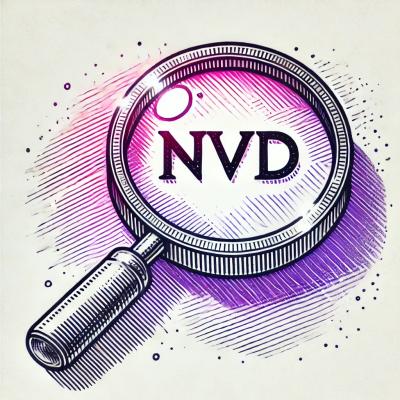
Security News
NIST Under Federal Audit for NVD Processing Backlog and Delays
As vulnerability data bottlenecks grow, the federal government is formally investigating NIST’s handling of the National Vulnerability Database.
apple-calendar-integration
Advanced tools
The ICloud API for events management
Run this command:
pip install apple-calendar-integration
from datetime import datetime, timedelta
from apple_calendar_integration import ICloudCalendarAPI
api = ICloudCalendarAPI(username, password)
start_date = int(datetime.now().timestamp())
end_date = start_date + timedelta(hours=2)
etag, ctag, guid = api.create_event('Your title', start_date, end_date)
This python script will create one two hour event from now. etag
, ctag
and guid
will be used to edit or delete event.
from apple_calendar_integration import ICloudCalendarAPI
api = ICloudCalendarAPI(username, password)
api.delete_event(new_etag, ctag, guid)
Should return True
if everything is OK.
{
"count": 5,
"freq": "daily"
}
This will mean repeat every day for 5 times(days).
freq
has such options:
Also, you can specify not num of times, but with the end date parameter.
{
"until": 1536910206,
"freq": "monthly"
}
All code together
from apple_calendar_integration import ICloudCalendarAPI
api = ICloudCalendarAPI(username, password)
repeat = {
"until": 1536910206,
"freq": "monthly"
}
new_etag = api.edit_event(etag, ctag, guid, repeat=repeat)
Should return new etag
if everything is OK
If you want to create alarm in the moment of event
{
"before": false
}
If before the event
{
"before": false,
"hours": 3,
"minutes": 2,
"seconds": 1
}
This will send notification about event 3 hours 2 minute and 1 second before event start date
There are such options:
All code together
from apple_calendar_integration import ICloudCalendarAPI
api = ICloudCalendarAPI(username, password)
alarm = {
"before": False,
"hours": 3,
"minutes": 2,
"seconds": 1
}
new_etag = api.edit_event(etag, ctag, guid, alarm=alarm)
Should return new etag
if everything is OK
How to add new people to event
from apple_calendar_integration import ICloudCalendarAPI
api = ICloudCalendarAPI(username, password)
invites = ["email_1@gmail.com", "email_2@gmail.com"]
new_etag = api.edit_event(etag, ctag, guid, add_invites=invites)
How to remove someone from invites
from apple_calendar_integration import ICloudCalendarAPI
api = ICloudCalendarAPI(username, password)
invites = ["email_1@gmail.com"]
new_etag = api.edit_event(new_etag, ctag, guid, remove_invites=invites)
Should return new etag
if everything is OK
note
, url
, title
or event date
from apple_calendar_integration import ICloudCalendarAPI
import time
api = ICloudCalendarAPI(username, password)
new_etag = api.edit_event(etag, ctag, guid,
note='New notw', url='https://my_url.com', title='New title',
start_date_timestamp=int(time.time()), end_date_timestamp=int(time.time()) + 1000)
Should return new etag
if everything is OK
FAQs
The ICloud API for events management
We found that apple-calendar-integration demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
As vulnerability data bottlenecks grow, the federal government is formally investigating NIST’s handling of the National Vulnerability Database.
Research
Security News
Socket’s Threat Research Team has uncovered 60 npm packages using post-install scripts to silently exfiltrate hostnames, IP addresses, DNS servers, and user directories to a Discord-controlled endpoint.
Security News
TypeScript Native Previews offers a 10x faster Go-based compiler, now available on npm for public testing with early editor and language support.