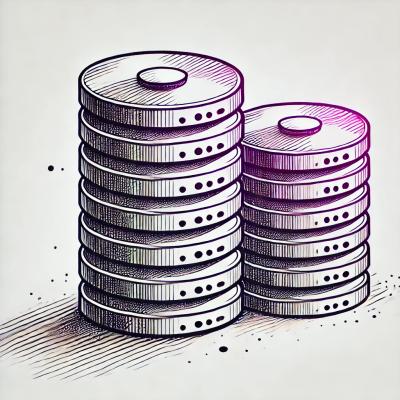
Security News
MCP Community Begins Work on Official MCP Metaregistry
The MCP community is launching an official registry to standardize AI tool discovery and let agents dynamically find and install MCP servers.
Python library for Redis object storage with sync/async interfaces.
pip install ashredis
from ashredis import AsyncRedisObject, RedisParams
REDIS_PARAMS = RedisParams(
host="localhost",
port=6379,
password="your_password",
db=0
)
class AsyncRedisObject(AsyncRedisObject):
"""Base class for all async models"""
def __init__(self, key: str | int = None, path: list[str] = None):
super().__init__(redis_params=REDIS_PARAMS, key=key, path=path)
class Product(AsyncRedisObject):
"""Product model example"""
name: str
price: float
stock: int
tags: list
metadata: dict
is_priority: bool
last_update_ts: int
__category__ = "product" # Redis key prefix
from datetime import timedelta, datetime
async with Product(key="prod_123") as p:
p.name = "Wireless Headphones"
p.price = 129.99
p.stock = 25
p.tags = ["audio", "wireless"]
p.metadata = {"brand": "Sony", "color": "black"}
p.is_priority = True
p.last_update_ts = int(datetime.now().timestamp() * 1000)
await p.save(ttl=timedelta(hours=2)) # Optional TTL
async with Product(key="prod_123") as p:
if await p.load():
print(f"Product: {p.name}")
print(f"Price: ${p.price}")
print(f"In Stock: {p.stock}")
async with Product(key="prod_123") as p:
p.stock = 20
await p.save()
async with Product(key="prod_123") as p:
if await p.delete():
print("Product deleted successfully")
# Creates key "product:electronics:audio:prod_123"
async with Product(key="prod_123", path=["electronics", "audio"]) as p:
p.name = "Studio Headphones"
await p.save()
# Get all products
all_products = await Product().load_all()
# Get sorted by price (descending)
expensive_first = await Product().load_sorted("price", reverse_sorted=True)
# Get recently updated
recent = await Product().load_for_time(
ts_field="last_update_ts",
time_range=timedelta(days=1)
)
async with Product(key="prod_123") as p:
await p.copy(p2) # Copies all data from original
p.price = 90.00
await p.save()
# Export to dict
product_data = {
"name": "Gaming Mouse",
"price": 59.99,
"stock": 30
}
async with Product(key="mouse_01") as p:
p.load_dict(product_data)
await p.save()
# Verify
exported = p.get_dict()
print(exported)
from datetime import timedelta
async with Product(key="temp_product") as p:
p.name = "Temporary Offer"
await p.save(ttl=timedelta(minutes=30))
# Check remaining time
ttl = await p.get_ttl()
print(f"Product expires in {ttl} seconds")
from datetime import datetime, timedelta
# Get events from specific time range
start_ts = int((datetime.now() - timedelta(hours=1)).timestamp() * 1000)
end_ts = int(datetime.now().timestamp() * 1000)
events = await Product().get_stream_in_interval(start_ts, end_ts)
for event in events:
print(f"Event: {event.name} at {event.last_update_ts}")
async def handle_event(event):
print(f"New product update: {event.name}")
# Start listening for events
async def monitor_updates():
product = Product(path=["electronics"])
await product.listen_for_stream(
callback=handle_event,
delay=1 # check every second
)
FAQs
Async Redis ORM for Python
We found that ashredis demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
The MCP community is launching an official registry to standardize AI tool discovery and let agents dynamically find and install MCP servers.
Research
Security News
Socket uncovers an npm Trojan stealing crypto wallets and BullX credentials via obfuscated code and Telegram exfiltration.
Research
Security News
Malicious npm packages posing as developer tools target macOS Cursor IDE users, stealing credentials and modifying files to gain persistent backdoor access.