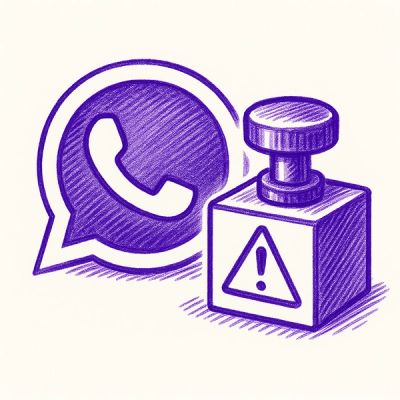
Research
/Security News
Malicious npm Packages Target WhatsApp Developers with Remote Kill Switch
Two npm packages masquerading as WhatsApp developer libraries include a kill switch that deletes all files if the phone number isn’t whitelisted.
ASK-Orex is a package designed to simplify regular expressions in Python. It provides a high-level interface for constructing and working with regular expressions, making it easier and more intuitive to use.
The package is heavily inspired by Richy Cotton's rebus
package for the R
language.
You can install Orex using pip:
pip install ask-orex
To use Orex, import the ask-orex
package into your Python script:
import ask_orex as ox
The easiest regular expression is just a literal string to be found.
s = 'Hello World'
pattern = ox.literal('Hello')
pattern.is_match(s)
True
Orex regular expressions are extended by simply using a +
.
A slightly more useful example is to find a hex colour
pattern = ox.literal('#') + ox.HEXDIGIT + ox.HEXDIGIT +\
ox.HEXDIGIT + ox.HEXDIGIT + ox.HEXDIGIT + ox.HEXDIGIT
s_hex = 'This package is #a83232 hot'
pattern.is_match(s_hex)
True
pattern.findall(s_hex)
['#a83232']
On the other hand
s_nonhex = 'Just a twitter handle: #Red123'
pattern.is_match(s_nonhex)
False
pattern.findall(s_nonhex)
[]
Clearly, the pattern is somewhat burdensome. We can alternatively write it like this
pattern = ox.literal('#') + ox.repeat(ox.HEXDIGIT, 6)
or
pattern = ox.literal('#') + ox.n_or_more(ox.HEXDIGIT, min=6, max=6)
Most of orex
functions accept other regular expressions as characters.
Say we want to find a regex to parse emails. We can define first all email allowed characters
allowed_characters = ox.character_class(ox.ALNUM + ox.DASH + '_' + ox.DOT)
where ox.ALNUM
are just all letters and numbers. Note that the _
is just used as a string.
In Orex it is okay to use strings as part of the pattern, unless they are at the start, where we use ox.literal
to show python that we mean pattern business. Whenever we want to use the .
or _
explicitly, it is best to use ox.DOT
or ox.DASH
, since .
and -
can have a special meaning in regular expression.
Next we define
user_name = ox.one_or_more(allowed_characters)
domain_name = ox.one_or_more(allowed_characters)
extension = ox.n_or_more(ox.ALPHA,2,4)
email_pattern = user_name + '@' + domain_name + ox.DOT + extension
email = 'captainspamalot@funnyspammail.com'
And indeed
email_pattern.is_match(email)
True
If we want to extract the individual components of a match, we have to capture them first. Using the email example above, we can just change the code to
email_pattern = ox.capture(user_name) + '@' + ox.capture(domain_name) \
+ ox.DOT + ox.capture(extension)
email_pattern.findall(email)
returns
[('captainspamalot', 'funnyspammail', 'com')]
Handy indeed! We can also name the individual components for even easier extraction
email_pattern = ox.capture(user_name,'user') \
+ '@' + ox.capture(domain_name,'domain') \
+ ox.DOT + ox.capture(extension,'ext')
We can now use the group_dict
method for even easier access
email_pattern.group_dict(email)
{'user': 'captainspamalot', 'domain': 'funnyspammail', 'ext': 'com'}
Capturing has another benefit, namely that we can use it to find repeated patterns with the ox.backreference
function
tag_name = ox.one_or_more(allowed_characters)
content = ox.one_or_more(ox.ANY_CHAR)
tag_pattern = ox.literal('<') + ox.capture(tag_name, 'tag') + '>' \
+ ox.capture(content,'content') + '</' +ox.backreference(name='tag')+'>'
message = '<name>Sir Snakington</name>'
tag_pattern.group_dict(message)
{'tag': 'name', 'content': 'Sir Snakington'}
Back references can also be used to substitute patterns, though here the named backreference does not work. Instead, the backrefence uses the index of the reference in the pattern.
tag_pattern = ox.literal('<') + ox.capture(tag_name) + '>' + ox.capture(content) + '</' +ox.backreference(1)+'>'
replace_pattern = ox.literal('<') + ox.backreference(2) + '>' + ox.backreference(1) + ox.literal('<') + ox.backreference(2) + '>'
tag_pattern.sub(message, replace_pattern)
'<Sir Snakington>name<Sir Snakington>'
FAQs
ASK-Orex: Ordinary human-friendly Regular Expressions
We found that ask-orex demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
/Security News
Two npm packages masquerading as WhatsApp developer libraries include a kill switch that deletes all files if the phone number isn’t whitelisted.
Research
/Security News
Socket uncovered 11 malicious Go packages using obfuscated loaders to fetch and execute second-stage payloads via C2 domains.
Security News
TC39 advances 11 JavaScript proposals, with two moving to Stage 4, bringing better math, binary APIs, and more features one step closer to the ECMAScript spec.