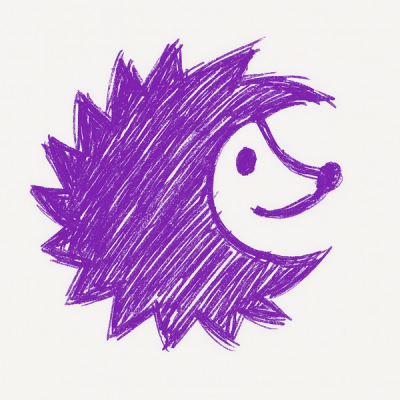
Security News
Browserslist-rs Gets Major Refactor, Cutting Binary Size by Over 1MB
Browserslist-rs now uses static data to reduce binary size by over 1MB, improving memory use and performance for Rust-based frontend tools.
bareunpy
is the python 3 library for bareun.
Bareun is a Korean NLP, which provides tokenizing, POS tagging for Korean.
pip3 install bareunpy
bareun1
server.bareunpy
library to any servers.docker pull bareunai/bareun:latest
import sys
import google.protobuf.text_format as tf
from bareunpy import Tagger
# You can get an API-KEY from https://bareun.ai/
# Please note that you need to sign up and verify your email.
# ์๋์ "https://bareun.ai/"์์ ์ด๋ฉ์ผ ์ธ์ฆ ํ ๋ฐ๊ธ๋ฐ์ API KEY("koba-...")๋ฅผ ์
๋ ฅํด์ฃผ์ธ์. "๋ก๊ทธ์ธ-๋ด์ ๋ณด ํ์ธ"
API_KEY = "koba-ABCDEFG-1234567-LMNOPQR-7654321" # <- ๋ณธ์ธ์ API KEY๋ก ๊ต์ฒด(Replace this with your own API KEY)
# If you have your own localhost bareun.
my_tagger = Tagger(API_KEY, 'localhost')
# or if you have your own bareun which is running on 10.8.3.211:15656.
my_tagger = Tagger(API_KEY, '10.8.3.211', 15656)
# print results.
res = tagger.tags(["์๋
ํ์ธ์.", "๋ฐ๊ฐ์์!"])
# get protobuf message.
m = res.msg()
tf.PrintMessage(m, out=sys.stdout, as_utf8=True)
print(tf.MessageToString(m, as_utf8=True))
print(f'length of sentences is {len(m.sentences)}')
## output : 2
print(f'length of tokens in sentences[0] is {len(m.sentences[0].tokens)}')
print(f'length of morphemes of first token in sentences[0] is {len(m.sentences[0].tokens[0].morphemes)}')
print(f'lemma of first token in sentences[0] is {m.sentences[0].tokens[0].lemma}')
print(f'first morph of first token in sentences[0] is {m.sentences[0].tokens[0].morphemes[0]}')
print(f'tag of first morph of first token in sentences[0] is {m.sentences[0].tokens[0].morphemes[0].tag}')
## Advanced usage.
for sent in m.sentences:
for token in sent.tokens:
for m in token.morphemes:
print(f'{m.text.content}/{m.tag}:{m.probability}:{m.out_of_vocab})
# get json object
jo = res.as_json()
print(jo)
# get tuple of pos tagging.
pa = res.pos()
print(pa)
# another methods
ma = res.morphs()
print(ma)
na = res.nouns()
print(na)
va = res.verbs()
print(va)
# custom dictionary
cust_dic = tagger.custom_dict("my")
cust_dic.copy_np_set({'๋ด๊ณ ์ ๋ช
์ฌ', '์ฐ๋ฆฌ์ง๊ณ ์ ๋ช
์ฌ'})
cust_dic.copy_cp_set({'์ฝ๋ก๋19'})
cust_dic.copy_cp_caret_set({'์ฝ๋ก๋^๋ฐฑ์ ', '"๋
๊ฐ^๋ฐฑ์ '})
cust_dic.update()
# laod prev custom dict
cust_dict2 = tagger.custom_dict("my")
cust_dict2.load()
tagger.set_domain('my')
tagger.pos('์ฝ๋ก๋19๋ ์ธ์ ๋๋ ๊น์?')
import sys
import google.protobuf.text_format as tf
from bareunpy import Tokenizer
# You can get an API-KEY from https://bareun.ai/
# Please note that you need to sign up and verify your email.
# ์๋์ "https://bareun.ai/"์์ ์ด๋ฉ์ผ ์ธ์ฆ ํ ๋ฐ๊ธ๋ฐ์ API KEY("koba-...")๋ฅผ ์
๋ ฅํด์ฃผ์ธ์. "๋ก๊ทธ์ธ-๋ด์ ๋ณด ํ์ธ"
API_KEY = "koba-ABCDEFG-1234567-LMNOPQR-7654321" # <- ๋ณธ์ธ์ API KEY๋ก ๊ต์ฒด(Replace this with your own API KEY)
# If you have your own localhost bareun.
my_tokenizer = Tokenizer(API_KEY, 'localhost')
# or if you have your own bareun which is running on 10.8.3.211:15656.
my_tokenizer = Tagger(API_KEY, '10.8.3.211', 15656)
# print results.
tokenized = tokenizer.tokenize_list(["์๋
ํ์ธ์.", "๋ฐ๊ฐ์์!"])
# get protobuf message.
m = tokenized.msg()
tf.PrintMessage(m, out=sys.stdout, as_utf8=True)
print(tf.MessageToString(m, as_utf8=True))
print(f'length of sentences is {len(m.sentences)}')
## output : 2
print(f'length of tokens in sentences[0] is {len(m.sentences[0].tokens)}')
print(f'length of segments of first token in sentences[0] is {len(m.sentences[0].tokens[0].segments)}')
print(f'tagged of first token in sentences[0] is {m.sentences[0].tokens[0].tagged}')
print(f'first segment of first token in sentences[0] is {m.sentences[0].tokens[0].segments[0]}')
print(f'hint of first morph of first token in sentences[0] is {m.sentences[0].tokens[0].segments[0].hint}')
## Advanced usage.
for sent in m.sentences:
for token in sent.tokens:
for m in token.segments:
print(f'{m.text.content}/{m.hint})
# get json object
jo = tokenized.as_json()
print(jo)
# get tuple of segments
ss = tokenized.segments()
print(ss)
ns = tokenized.nouns()
print(ns)
vs = tokenized.verbs()
print(vs)
# postpositions: ์กฐ์ฌ
ps = tokenized.postpositions()
print(ps)
# Adverbs, ๋ถ์ฌ
ass = tokenized.adverbs()
print(ass)
ss = tokenized.symbols()
print(ss)
from bareunpy import Corrector
# You can get an API-KEY from https://bareun.ai/
# Please note that you need to sign up and verify your email.
# ์๋์ "https://bareun.ai/"์์ ์ด๋ฉ์ผ ์ธ์ฆ ํ ๋ฐ๊ธ๋ฐ์ API KEY("koba-...")๋ฅผ ์
๋ ฅํด์ฃผ์ธ์. "๋ก๊ทธ์ธ-๋ด์ ๋ณด ํ์ธ"
API_KEY = "koba-ABCDEFG-1234567-LMNOPQR-7654321" # <- ๋ณธ์ธ์ API KEY๋ก ๊ต์ฒด(Replace this with your own API KEY)
# Initialize Corrector
corrector = Corrector(API_KEY)
# Single sentence correction
response = corrector.correct_error("์์ ๋ ์ค๊ธฐ๊ฐ ์์ด์ ์๋ค์ ๊ฒ ๊ฐ์ ๊ฝ์๋ฌผ์ ์ฃผ์๋ค.")
print(f"Original: {response.origin}")
print(f"Corrected: {response.revised}")
corrector.print_results(response)
# Multiple sentences correction
responses = corrector.correct_error_list([
"์ด๋จธ๋ ๊ป์ ๋ง๋ค์ด์ฃผ์ ๊น์น์ฐ๊ฒ๊ฐ๋๋ฌด๋งต๋ค๋ฉฐ๋์์ด์ธ์ด๋ฒ๋ ธ๋ค.",
"์์ ๋ ์ค๊ธฐ๊ฐ ์์ด์ ์๋ค์ ๊ฒ ๊ฐ์ ๊ฝ์๋ฌผ์ ์ฃผ์๋ค."
])
for res in responses:
print(f"Original: {res.origin}")
print(f"Corrected: {res.revised}")
corrector.print_results(responses)
# JSON output
corrector.print_as_json(response)
FAQs
The bareun python library using grpc
We found that bareunpy demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago.ย It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Browserslist-rs now uses static data to reduce binary size by over 1MB, improving memory use and performance for Rust-based frontend tools.
Research
Security News
Eight new malicious Firefox extensions impersonate games, steal OAuth tokens, hijack sessions, and exploit browser permissions to spy on users.
Security News
The official Go SDK for the Model Context Protocol is in development, with a stable, production-ready release expected by August 2025.