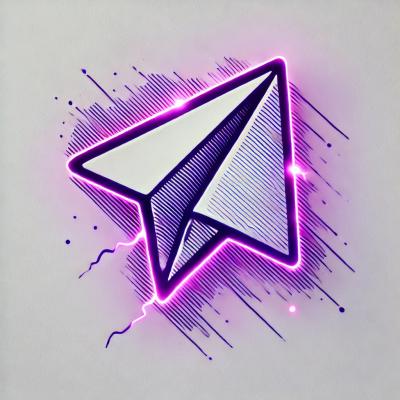
Research
npm Malware Targets Telegram Bot Developers with Persistent SSH Backdoors
Malicious npm packages posing as Telegram bot libraries install SSH backdoors and exfiltrate data from Linux developer machines.
basic-api-client-python
Advanced tools
This library helps you easily create a python ' 'application to connect the WhatsApp API using service api.basis-api.com
Python library for intagration with WhatsAPP messanger via API of api.basis-api.com service. To use the library you have to get a registration token and an account id in the personal area. There is a free developer account tariff plan.
You can find REST API documentation by url. The library is a wrapper for REST API, so the documentation at the above url applies to the library as well.
pip install basic-api-client-python
from basic_api_client_python import API
To send a message or to exacute some other Basic-API method, you have to have the WhatsApp account in the phone application to be authorized. To authorize your account please go to the personal area and scan a QR-code using the WhatsApp application.
basicAPI = API.BasicApi(ID_INSTANCE, API_TOKEN_INSTANCE)
result = basicAPI.sending.sendMessage('79001234567@g.us', 'Message text')
Example url: sendTextMessage.py
Please note that keys can be obtained from environment variables:
from os import environ
ID_INSTANCE = environ['ID_INSTANCE']
API_TOKEN_INSTANCE = environ['API_TOKEN_INSTANCE']
result = basicAPI.sending.sendFileByUrl('120363025955348359@g.us',
'https://www.google.ru/images/branding/googlelogo/1x/googlelogo_color_272x92dp.png',
'googlelogo_color_272x92dp.png', 'Google logo')
Example url: sendPictureByLink.py
result = basicAPI.sending.sendFileByUpload('120363025955348359@g.us',
'C:\Games\PicFromDisk.png',
'PicFromDisk.png', 'Picture from disk')
Example url: sendPictureByUpload.py
chatIds = [
"79001234567@c.us"
]
resultCreate = basicAPI.groups.createGroup('GroupName',
chatIds)
if resultCreate.code == 200:
resultSend = basicAPI.sending.sendMessage(resultCreate.data['chatId'],
'Message text')
IMPORTANT: If one tries to create a group with a non-existent number, WhatsApp may block the sender's number. The number in the example is non-existent.
Example url: createGroupAndSendMessage.py
The general concept of receiving data in the Basic API is described here To start receiving messages by the HTTP API you need to execute the library method:
basicAPI.webhooks.startReceivingNotifications(onEvent)
onEvent - your method which should contain parameters:
Parameter | Description |
---|---|
typewebhook | received message type (string) |
body | message body (json) |
Message body types and formats here
This method will be called when an incoming message is received. Next, process messages according to the business logic of your system.
Description | Module |
---|---|
Example of sending text | sendTextMessage.py |
Example of sending a picture by URL | sendPictureByLink.py |
Example of sending a picture by uploading from the disk | sendPictureByUpload.py |
Example of a group creation and sending a message to the group | createGroupAndSendMessage.py |
Example of incoming webhooks receiving | receiveNotification.py |
Method | Description | Documentation |
---|---|---|
account.getSettings | The method is aimed for getting the current settings of the account | GetSettings.md |
account.getStateInstance | The method is aimed for getting the account state | GetStateInstance.md |
account.getStatusInstance | The method is aimed for getting the status of the account instance socket connection with WhatsApp | GetStatusInstance.md |
account.logout | The method is aimed for logging out an account | Logout.md |
account.qr | The method is aimed for getting QR code | QR.md |
account.reboot | The method is aimed for rebooting an account | Reboot.md |
account.setProfilePicture | The method is aimed for setting an account picture | SetProfilePicture.md |
account.setSettings | The method is aimed for setting an account settings | SetSettings.md |
account.setSystemProxy | The method is aimed for setting a system proxy. Use the method when you need to reset custom proxy settings to system ones | SetSystemProxy.md |
groups.addGroupParticipant | Метод добавляет участника в групповой чат | AddGroupParticipant.md |
groups.createGroup | The method adds a participant to a group chat. IMPORTANT: If one tries to create a group with a non-existent number, WhatsApp may block the sender's number. | CreateGroup.md |
groups.getGroupData | The method gets group chat data | GetGroupData.md |
groups.leaveGroup | The method makes the current account user leave the group chat | LeaveGroup.md |
groups.removeAdmin | he method removes a participant from group chat administration rights | RemoveAdmin.md |
groups.removeGroupParticipant | The method removes a participant from a group chat | RemoveGroupParticipant.md |
groups.setGroupAdmin | The method sets a group chat participant as an administrator | SetGroupAdmin.md |
groups.setGroupPicture | The method sets a group picture | SetGroupPicture.md |
groups.updateGroupName | The method changes a group chat name | UpdateGroupName.md |
journals.getChatHistory | The method returns the chat message history | GetChatHistory.md |
journals.lastIncomingMessages | The method returns the last incoming messages of the account. In the default mode the incoming messages for 24 hours are returned | GetChatHistory.md |
journals.lastOutgoingMessages | The method returns the last outgoing messages of the account. In the default mode the last messages for 24 hours are returned | LastOutgoingMessages.md |
marking.readChat | The method is aimed for marking messages in a chat as read. Either all messages or a specified message in a chat can be marked as read | ReadChat.md |
device.getDeviceInfo | The method is aimed for getting information about the device (phone) running WhatsApp Business application | GetDeviceInfo.md |
queues.clearMessagesQueue | The method is aimed for clearing the queue of messages to be sent | ClearMessagesQueue.md |
queues.showMessagesQueue | The method is aimed for getting a list of messages in the queue to be sent | ShowMessagesQueue.md |
receiving.deleteNotification | The method is aimed for deleting an incoming notification from the notification queue | DeleteNotification.md |
receiving.receiveNotification | The method is aimed for receiving one incoming notification from the notifications queue | ReceiveNotification.md |
sending.sendButtons | The method is aimed for sending a button message to a personal or a group chat | SendButtons.md |
sending.sendContact | The method is aimed for sending a contact message | SendContact.md |
sending.sendFileByUpload | The method is aimed for sending a file uploaded by form | SendFileByUpload.md |
sending.sendFileByUrl | The method is aimed for sending a file uploaded by Url | SendFileByUrl.md |
sending.sendLink | The method is aimed for sending a message with a link, by which an image preview, title and description will be added | SendLink.md |
sending.sendListMessage | The method is aimed for sending a message with a select button from a list of values to a personal or a group chat | SendListMessage.md |
sending.sendLocation | The method is aimed for sending a location message | SendLocation.md |
sending.sendMessage | The method is aimed for sending a text message to a personal or a group chat | SendMessage.md |
sending.sendTemplateButtons | The method is aimed for sending a message with template list interactive buttons to a personal or a group chat | SendTemplateButtons.md |
sending.forwardMessages | The method is intended for forwarding messages to a personal or group chat | ForwardMessages.md |
serviceMethods.checkWhatsapp | The method checks WhatsApp account availability on a phone number | CheckWhatsapp.md |
serviceMethods.getAvatar | The method returns a user or a group chat avatar | GetAvatar.md |
serviceMethods.getContactInfo | The method is aimed for getting information on a contact | GetContactInfo.md |
serviceMethods.getContacts | The method is aimed for getting a list of the current account contacts | GetContacts.md |
serviceMethods.setDisappearingChat | The method is aimed for changing settings of disappearing messages in chats. The standard settings of the application are to be used: 0 (off), 86400 (24 hours), 604800 (7 days), 7776000 (90 days) | SetDisappearingChat.md |
serviceMethods.archiveChat | The method archives a chat. One can archive chats that have at least one incoming message | ArchiveChat.md |
serviceMethods.deleteMessage | The method deletes a message from a chat | DeleteMessage.md |
serviceMethods.unarchiveChat | The method unarchives a chat | UnarchiveChat.md |
serviceMethods.setDisappearingChat | The method is aimed for changing settings of disappearing messages in chats | SetDisappearingChat.md |
webhooks.startReceivingNotifications | The method is aimed for starting to receive webhooks | |
webhooks.stopReceivingNotifications | The method is aimed for stopping to receive webhooks |
https://api.basis-api.com/en/docs/api/
Licensed under MIT terms. Please see file LICENSE
FAQs
This library helps you easily create a python ' 'application to connect the WhatsApp API using service api.basis-api.com
We found that basic-api-client-python demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Malicious npm packages posing as Telegram bot libraries install SSH backdoors and exfiltrate data from Linux developer machines.
Security News
pip, PDM, pip-audit, and the packaging library are already adding support for Python’s new lock file format.
Product
Socket's Go support is now generally available, bringing automatic scanning and deep code analysis to all users with Go projects.