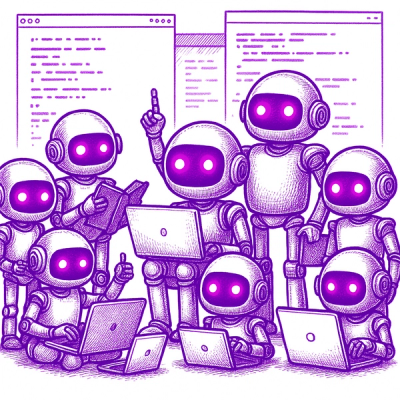
Security News
Open Source CAI Framework Handles Pen Testing Tasks up to 3,600× Faster Than Humans
CAI is a new open source AI framework that automates penetration testing tasks like scanning and exploitation up to 3,600× faster than humans.
A high-performance Rust (via PyO3) implementation of a Binary Indexed Tree (Fenwick Tree), designed for efficient prefix sum queries and point updates in Python.
Fenwick Trees are ideal for problems requiring dynamic prefix sums or frequent updates, where a static array would be too slow. Common use cases include:
Structure | Build Time | Update Time | Prefix Sum Time | Space | Use Case |
---|---|---|---|---|---|
Binary Indexed Tree | O(n log n) | O(log n) | O(log n) | O(n) | Dynamic prefix sums |
Prefix Sum Array | O(n) | O(n) | O(1) | O(n) | Static arrays (no updates) |
Segment Tree | O(n) | O(log n) | O(log n) | O(4n) | Flexible range operations but heavier |
pip install bit_ds
There are 2 classes defined by the library:
BIT
: A class for creating a Binary Indexed Tree (Fenwick Tree) using a list of integers.NdBIT
: A class for creating a multi-dimensional Binary Indexed Tree (Fenwick Tree) using a numpy list of integers.Optional:
numpy
(for NdBIT)from bit_ds import BIT
# Initialize a BIT using a list of integers
bit = BIT([1, 2, 3, 4, 5])
# Point update: Add 5 to index 2 (0-based)
bit.update(2, 5)
# Prefix sum: Sum from index 0 to 2 (inclusive, 0-based)
print(bit.query(2)) # Output: 6
# Range sum: Sum from index 2 to 4 (inclusive, 0-based)
print(bit.range_query(2, 4)) # Output: 12
BIT(input: list)
Method | Description | Time Complexity |
---|---|---|
update(index: int, delta: int) | Updates the value at index (0-based) by delta . | O(log n) |
query(index: int) -> int | Returns the sum from [0, index] (inclusive). | O(log n) |
range_query(l: int, r: int) -> int | Returns the sum from [l, r] (inclusive). | O(log n) |
update(0, x)
affects the first element.query(3)
returns the sum from the first to the fourth element.range_query(l, r)
is equivalent to query(r) - query(l-1)
(handles bounds automatically).update(i, -5)
to subtract values.WIP
Pull requests welcome! For major changes, open an issue first.
FAQs
A Python library for the Binary Indexed Tree data structure.
We found that bit-ds demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
CAI is a new open source AI framework that automates penetration testing tasks like scanning and exploitation up to 3,600× faster than humans.
Security News
Deno 2.4 brings back bundling, improves dependency updates and telemetry, and makes the runtime more practical for real-world JavaScript projects.
Security News
CVEForecast.org uses machine learning to project a record-breaking surge in vulnerability disclosures in 2025.