

An improved Canvas widget for tkinter with more functionality to display graphical elements like lines or text.
Tkinter's Canvas widget has some limitations which are adressed in this package.
Simmilar to the default tkinter Canvas widget, e.g canvas.create_rectangle, other canvas objects can be created.
Availability
To start, make sure you have CanvasPlus installed or cloned. You can do this with one of two methods.
Option 1: Pip
Pip install this package
pip install CanvasPlus
Option 2: Github
Download a zip file containing all files.
git clone https://github.com/Luke-zhang-04/CanvasPlus.git
or
Clone the latest stable Release


Importing
It is advised that you do not import the entire module.
from CanvasPlus import CanvasPlus
CanvasPlus()
import CanvasPlus
CanvasPlus.CanvasPlus()
Usage
Usage is very simple, especially for those with experience using tkinter canvas.
For complete documentation, head over the the wiki
Example:
from CanvasPlus import CanvasPLus
from tkinter import Tk, StringVar, DoubleVar
root = Tk()
canvas = CanvasPlus(root, width=800, height=800, background = "white")
canvas.pack()
canvas.create_circle(300, 600, 100, fill = "black", outline = "green", width = 3)
canvas.create_round_rectangle(
400, 550, 500, 650, radius = 75, fill = "blue", outline = "orange", width = 5
)
arrow = canvas.create_arrow(600, 600, 50, 50, 150, 20, fill = "grey", outline = "black")
canvas.rotate(arrow, 600, 600, 310, unit="deg")
rect = canvas.create_rectangle(100, 550, 200, 650, fill = "#f7a8c6", width = 0)
canvas.clone(rect)
rect = canvas.poly(rect)
canvas.rotate(rect, 150, 600, math.pi/4)
content = StringVar()
canvas.create_entry(0, 0, anchor = "nw", textvariable = content, fg = "blue", bg = "gold")
content.set("This is CanvasPlus %s" % _canvasPlusVersion)
canvas.create_button(
5, 25, anchor = "nw", text = "button", width = 50, highlightbackground = "red",
command = lambda e = content: print(e.get())
)
_, checkbutton = canvas.create_checkbutton(
5, 50, anchor = "nw", bg = "brown", fg = "white", text = "My Checkbutton"
)
checkbutton.toggle()
canvas.create_label(
5, 75, font = ("Times", "24"), fg = "black", bg = "green", text = "By Luke-zhang-04", anchor = "nw"
)
aPrime = canvas.create_polygon(500, 10, 500, 20, 550, 25, 600, 20, 600, 10, fill = "yellow", outline = "black")
a = canvas.clone(aPrime)
canvas.flip(a, m = .5, b = -200)
canvas.update()
canvas.mainloop()
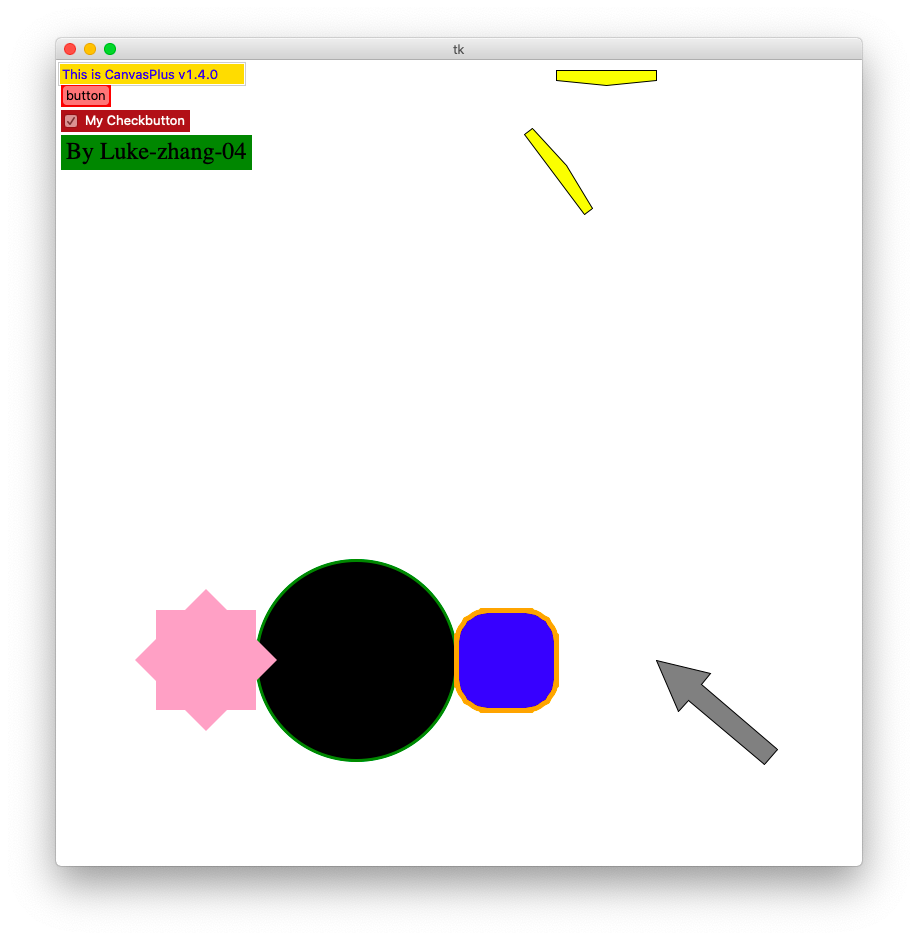