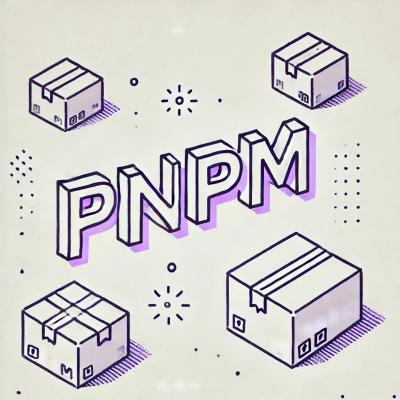
Security News
pnpm 10.12 Introduces Global Virtual Store and Expanded Version Catalogs
pnpm 10.12.1 introduces a global virtual store for faster installs and new options for managing dependencies with version catalogs.
A lightweight Python library for rendering Vega-Lite JSON charts in Jupyter/Colab.
ChartSpan is a Python library designed for rendering Vega-Lite JSON specifications directly within Google Colab. It simplifies the visualization of interactive charts and supports customization for chart dimensions, text color, and background color.
To install ChartSpan, use pip:
pip install chartspan
Here is an example of how to use ChartSpan to render a Vega-Lite JSON chart:
from chartspan import ChartSpan
import json
# Load Vega-Lite JSON specification
with open("example.json", "r") as f:
spec = json.load(f)
# Render chart inline
chart = ChartSpan(width=800, height=600)
chart.render_inline(spec)
You can specify custom dimensions for the chart:
chart = ChartSpan(width=1000, height=800)
chart.render_inline(spec)
You can also customize the text color for labels, titles, legends, and axes:
chart = ChartSpan(width=800, height=600, text_color="blue")
chart.render_inline(spec)
Alternatively, you can override the default text color for a specific chart:
chart = ChartSpan(width=800, height=600, text_color="black")
chart.render_inline(spec, text_color="red")
You can also customize the background color of the chart:
chart = ChartSpan(width=800, height=600, text_color="black")
chart.render_inline(spec, background_color="lightgray")
This modifies the chart's width, height, text color, and background color, ensuring it fits your specific needs and visual style.
Save the following Vega-Lite JSON to a file named example.json
:
{
"$schema": "https://vega.github.io/schema/vega-lite/v5.json",
"mark": "point",
"data": {
"values": [
{"x": 1, "y": 2},
{"x": 2, "y": 3}
]
},
"encoding": {
"x": {"field": "x", "type": "quantitative"},
"y": {"field": "y", "type": "quantitative"}
}
}
Run the following Python code:
from chartspan import ChartSpan
import json
with open("example.json", "r") as f:
spec = json.load(f)
chart = ChartSpan(width=800, height=600, text_color="#dcdfe1")
chart.render_inline(spec, background_color="#353535")
This will display an interactive scatter plot in your Google Colab environment, with labels and titles rendered in green and a light blue background.
IPython
Install dependencies using:
pip install IPython
ChartSpan is licensed under the MIT License.
Contributions are welcome! If you'd like to contribute, please fork the repository and submit a pull request.
ChartSpan uses the Vega-Lite and Vega-Embed libraries for rendering charts. Special thanks to the developers of these powerful visualization tools.
FAQs
A lightweight Python library for rendering Vega-Lite JSON charts in Jupyter/Colab.
We found that chartspan demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
pnpm 10.12.1 introduces a global virtual store for faster installs and new options for managing dependencies with version catalogs.
Security News
Amaro 1.0 lays the groundwork for stable TypeScript support in Node.js, bringing official .ts loading closer to reality.
Research
A deceptive PyPI package posing as an Instagram growth tool collects user credentials and sends them to third-party bot services.