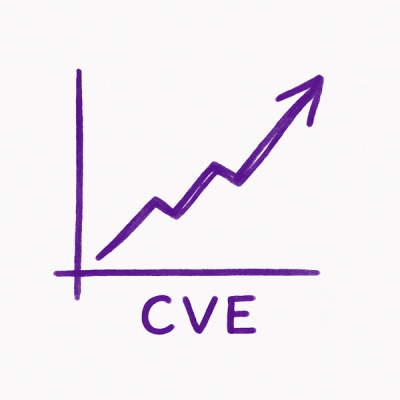
Security News
New CVE Forecasting Tool Predicts 47,000 Disclosures in 2025
CVEForecast.org uses machine learning to project a record-breaking surge in vulnerability disclosures in 2025.
A collection of Python functions designed to validate various types of input. These functions can be used to ensure that user inputs such as passwords, emails, and other required fields meet specific criteria.
This repository contains a collection of Python functions designed to validate various types of input. These functions can be used to ensure that user inputs such as passwords, emails, and other required fields meet specific criteria.
You can install this package using pip:
pip install checkBest
Here is how you can use the provided validation functions:
from checkBest import checkPassword
password = "YourP@ssw0rd"
if checkPassword(password):
print("Password is valid!")
else:
print("Password is invalid.")
from checkBest import checkEmail
email = "example@example.com"
if checkEmail(email):
print("Email is valid!")
else:
print("Email is invalid.")
from checkBest import checkInput
input_value = ["test", 2, "test2", null]
if checkInput(input_value):
print("Inputs is not null!")
else:
print("Inputs is null.")
from checkBest import checkPhone
input_value = "09123456789"
if checkPhone(input_value, "phone"):
print("Inputs is valid!")
else:
print("Inputs is invalid.")
input_value = "01234567890"
if checkPhone(input_value, "line"):
print("Input is valid!")
else:
print("Input is invalid.")
from checkBest import checkInputInt
input_value = "524155"
if checkInputInt(input_value) is False:
print("Input is not Int!")
else:
print("Input is Int.")
True
if the password is valid based on predefined criteria.True
if the email is valid.True
if the all inputs is not null or empty.True
if the input is a phone or live valid.False
if the input is not Int.If you would like to contribute to this project, please fork the repository and submit a pull request. We welcome any improvements or additional validators.
For any questions or inquiries, feel free to open an issue or contact me at senator136019@gmail.com.
FAQs
A collection of Python functions designed to validate various types of input. These functions can be used to ensure that user inputs such as passwords, emails, and other required fields meet specific criteria.
We found that checkBest demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
CVEForecast.org uses machine learning to project a record-breaking surge in vulnerability disclosures in 2025.
Security News
Browserslist-rs now uses static data to reduce binary size by over 1MB, improving memory use and performance for Rust-based frontend tools.
Research
Security News
Eight new malicious Firefox extensions impersonate games, steal OAuth tokens, hijack sessions, and exploit browser permissions to spy on users.