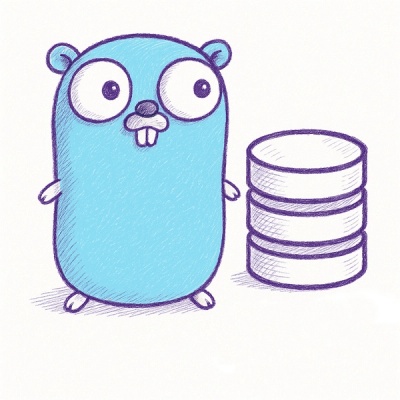
Security News
Official Go SDK for MCP in Development, Stable Release Expected in August
The official Go SDK for the Model Context Protocol is in development, with a stable, production-ready release expected by August 2025.
Code Procrastinator is a Python package that humanizes your code by giving it the ability to procrastinate—just like you! :D
Instead of executing tasks efficiently, it gets distracted, mindlessly browses the internet, or justifies its procrastination with excuses.
Bryant To Andrew Bao Jasmine Zhang Imran Ahmed
You can install Code Procrastinator from PyPI using pip
:
This package requires Python 3.7 or higher
pip install code-procrastinator
Want to improve Code Procrastinator? Follow these steps to set up your development environment!
git clone https://github.com/software-students-spring2025/3-python-package-jeff-bezos.git
cd 3-python-package-jeff-bezos
pip install pipenv
pipenv shell
We automatically upload our package to PyPi using twine.
Before merging anything, ensure your contributions pass the unit tests
Important: PyPI will not let you upload a duplicate version, make sure to increment your version in your pyproject.toml file before pushing any changes. (Ex: 0.1.6 -> 0.1.7)
pytest procrastinator.py
The procrastinate
function simulates what a human might do when procrastinating—whether it's scrolling the web, making excuses, or delaying execution.
To use Code Procrastinator in your own Python projects, simply import and call the procrastinate function:
from code_procrastinator import procrastinate
# Make your program procrastinate for up to 10 seconds, with 3 random delays
procrastinate(10, 3)
0
and max_time
.Argument | Type | Description |
---|---|---|
max_time | int | The maximum number of seconds for any delay. Must be greater than 0 . |
delay_count | int | The number of procrastination delays. Must be greater than 0 . |
ValueError
: Raised if max_time
or delay_count
is less than or equal to 0
.set
: A set of unique delay times used during procrastination.>>> procrastinate(10, 3)
Procrastinating...
Let's take an internet break! :D https://en.wikipedia.org/wiki/Special:Random
Procrastinating...
Wow, this 4-hour video essay looks interesting...
Procrastinating...
I'll do it tomorrow
The excuse_wrapper
function will also procrasinate on providing correct output, will relay an excuse, and has a chance to completely fail on the task.
Import excuse_wrapper, and use it as a decorator for the original function
from code_procrastinator import excuse_wrapper
@procrastinator.excuse_wrapper
def add(x, y):
return x + y
add(1, 2)
>>> add(1. 2)
Optimizing best possible answer
Processing... 25% done.
Still thinking... 50% done.
Someone just deleted my work... I need to start over again...
TBH... forget this I'll do it tomorrow.
The reaffirm_program
function checks if the input message contains any positive keywords. When it detects encouragement, it prints a motivational message and confirms that the program is ready to continue running. This function is useful when you want your application to "get back on track" after a period of procrastination.
Example Usage
from code_procrastinator import reaffirm_program
# Provide a message with positive keywords to trigger the function
result = reaffirm_program("You're awesome and brilliant!")
print(result) # Expected output: "Program is now running! Let's do this!"
Argument | Type | Description |
---|---|---|
message | str | The input message which is checked for positive keywords. |
str
: Returns "Program is now running! Let's do this!" if the input contains positive keywords.None
:Returns None if no positive keywords are found.The random_fail_wrapper
function decorator randomly determines whether the decorated function will execute normally or fail by raising an IllDoItLaterException
. In both cases, a randomly chosen message prints to the console
Import random_fail_wrapper
from Code Procrastinator
from code_procrastinator import random_fail_wrapper
@random_fail_wrapper
def compute_sum(a, b):
return a + b
compute_sum(3, 4)
IllDoItLaterException
: Raised if the randomly generated number is between 1 and 50.wrapper
: A wrapper of the original function with the additional random failure "functionality">>> compute_sum(3, 4)
Ugh, I guess I'll run this now.
7
>>> compute_sum(3, 4)
IllDoItLaterException: Oh wait, I left my oven running. Sorry, but I cant run this code right now, I have to go...
FAQs
Python Package for functions that will procrastinate on their outputs
We found that code-procrastinator demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
The official Go SDK for the Model Context Protocol is in development, with a stable, production-ready release expected by August 2025.
Security News
New research reveals that LLMs often fake understanding, passing benchmarks but failing to apply concepts or stay internally consistent.
Security News
Django has updated its security policies to reject AI-generated vulnerability reports that include fabricated or unverifiable content.