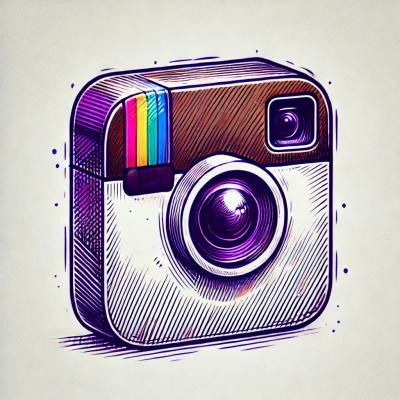
Research
PyPI Package Disguised as Instagram Growth Tool Harvests User Credentials
A deceptive PyPI package posing as an Instagram growth tool collects user credentials and sends them to third-party bot services.
Core integration package for building AI agents with CrewAI, providing configuration management, memory systems, and tool integration
Core integration package for building AI agents with CrewAI, providing configuration management, memory systems, tool integration, and API capabilities.
cognition-core/
├── src/
│ └── cognition_core/
│ ├── api.py # Core API implementation
│ ├── crew.py # Enhanced CrewAI base
│ ├── agent.py # Enhanced Agent class
│ ├── task.py # Enhanced Task class
│ ├── llm.py # Portkey LLM integration
│ ├── logger.py # Logging system
│ ├── config.py # Configuration management
│ ├── memory/ # Memory implementations
│ │ ├── entity.py # Entity memory with Chroma
│ │ ├── long_term.py # PostgreSQL long-term memory
│ │ ├── short_term.py # Chroma short-term memory
│ │ ├── storage.py # ChromaDB storage implementation
│ │ └── mem_svc.py # Memory service orchestration
│ └── tools/ # Tool management
│ ├── custom_tool.py # Base for custom tools
│ └── tool_svc.py # Dynamic tool service
@CognitionCoreCrewBase
decoratorRequired:
PORTKEY_API_KEY
: API key for Portkey LLM routingPORTKEY_VIRTUAL_KEY
: Virtual key for PortkeyCOGNITION_CONFIG_DIR
: Path to your configuration directory (e.g., "/home/user/.cognition/cognition-config-demo/config")Optional:
COGNITION_CONFIG_SOURCE
: Git repository URL to clone configuration (e.g., "git@github.com:user/config-repo.git")
COGNITION_CONFIG_DIR
should then point to the config directory within the cloned repoCONFIG_RELOAD_TIMEOUT
: Config reload timeout (default: 0.1)LONG_TERM_DB_PASSWORD
: PostgreSQL database passwordCHROMA_PASSWORD
: ChromaDB passwordAPP_LOG_LEVEL
: Logging level (default: INFO)Note: When using remote configuration:
COGNITION_CONFIG_DIR
to point to the config directory within the cloned repositoryfrom cognition_core import CognitionCoreCrewBase
from crewai import Agent, Task
@CognitionCoreCrewBase
class YourCrew:
@agent
def researcher(self) -> Agent:
return self.get_cognition_agent(
config=self.agents_config["researcher"],
llm=self.init_portkey_llm(
model="gpt-4",
portkey_config=self.portkey_config
)
)
@task
def research_task(self) -> Task:
return CognitionTask(
config=self.tasks_config["research"],
tools=["calculator", "search"]
)
@crew
def crew(self) -> Crew:
return CognitionCrew(
agents=self.agents,
tasks=self.tasks,
process=Process.sequential,
memory=True,
tool_service=self.tool_service
)
# Access API
app = YourCrew().api
short_term_memory:
enabled: true
external: true
host: "localhost"
port: 8000
collection_name: "short_term"
long_term_memory:
enabled: true
external: true
connection_string: "postgresql://user:${LONG_TERM_DB_PASSWORD}@localhost:5432/db"
entity_memory:
enabled: true
external: true
host: "localhost"
port: 8000
collection_name: "entities"
embedder:
provider: "ollama"
config:
model: "nomic-embed-text"
vector_dimension: 384
tool_services:
- name: "primary_service"
enabled: true
base_url: "http://localhost:8080/api/v1"
endpoints:
- path: "/tools"
method: "GET"
settings:
cache:
enabled: true
ttl: 3600
validation:
response_timeout: 30
MIT
FAQs
Core integration package for building AI agents with CrewAI, providing configuration management, memory systems, and tool integration
We found that cognition-core demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
A deceptive PyPI package posing as an Instagram growth tool collects user credentials and sends them to third-party bot services.
Product
Socket now supports pylock.toml, enabling secure, reproducible Python builds with advanced scanning and full alignment with PEP 751's new standard.
Security News
Research
Socket uncovered two npm packages that register hidden HTTP endpoints to delete all files on command.