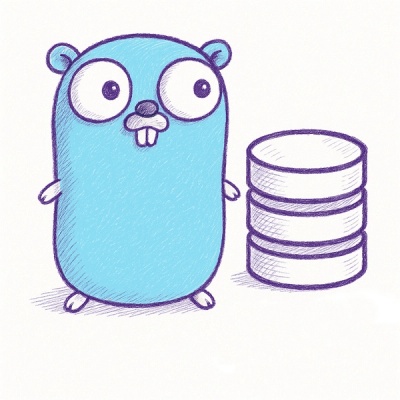
Security News
Official Go SDK for MCP in Development, Stable Release Expected in August
The official Go SDK for the Model Context Protocol is in development, with a stable, production-ready release expected by August 2025.
Colab Print is a Python library that enhances the display capabilities of Jupyter and Google Colab notebooks, providing beautiful, customizable HTML outputs for text, lists, dictionaries, tables, pandas DataFrames, and progress bars.
pip install colab-print
from colab_print import Printer, header, success, progress
import pandas as pd
import time
# Create a printer with default styles
printer = Printer()
# Use pre-configured styling functions
header("Colab Print Demo")
success("Library loaded successfully!")
# Display styled text
printer.display("Hello, World!", style="highlight")
# Display a list with nested elements (automatically detected and styled)
my_list = ['apple', 'banana', ['nested', 'item'], 'cherry', {'key': 'value'}]
printer.display_list(my_list, ordered=True, style="info")
# Show a progress bar
for i in progress(range(10), desc="Processing"):
time.sleep(0.2) # Simulate work
# Display a dictionary
my_dict = {
'name': 'Alice',
'age': 30,
'address': {'street': '123 Main St', 'city': 'Anytown'}
}
printer.display_dict(my_dict, style="success")
# Display a simple table
headers = ["Name", "Age", "City"]
rows = [
["Alice", 28, "New York"],
["Bob", 34, "London"],
["Charlie", 22, "Paris"]
]
printer.display_table(headers, rows, style="default")
# Display a pandas DataFrame with styling
df = pd.DataFrame({
'Name': ['Alice', 'Bob', 'Charlie'],
'Age': [28, 34, 22],
'City': ['New York', 'London', 'Paris']
})
printer.display_df(df,
highlight_cols=['Name'],
highlight_cells={(0, 'Age'): "background-color: #FFEB3B;"},
caption="Sample DataFrame")
Colab Print provides convenient shortcut functions with pre-configured styling:
from colab_print import (
header, title, subtitle, section_divider, subheader,
code, card, quote, badge, data_highlight, footer,
highlight, info, success, warning, error, muted, primary, secondary,
dfd, table, list_, dict_, progress
)
# Display styled text with a single function call
header("Main Section")
title("Document Title")
subtitle("Supporting information")
success("Operation completed!")
warning("Proceed with caution")
error("An error occurred")
code("print('Hello World')")
# Display different content types with shortcuts
table(headers, rows)
list_(my_list, ordered=True)
dict_(my_dict)
dfd(df, highlight_cols=["Name"])
default
- Clean, professional stylinghighlight
- Stand-out text with emphasisinfo
- Informational blue textsuccess
- Positive green messagewarning
- Attention-grabbing yellow alerterror
- Critical red messagemuted
- Subtle gray textprimary
- Primary blue-themed textsecondary
- Secondary purple-themed textcode
- Code-like display with monospace fontcard
- Card-like container with shadowquote
- Styled blockquotenotice
- Attention-drawing noticebadge
- Compact badge-style displayYou can add your own styles:
printer = Printer()
printer.add_style("custom", "color: purple; font-size: 20px; font-weight: bold;")
printer.display("Custom styled text", style="custom")
printer.display(text, style="default", **inline_styles)
: Displays styled text.printer.display_list(items, ordered=False, style="default", item_style=None, **inline_styles)
: Displays lists/tuples.printer.display_dict(data, style="default", key_style=None, value_style=None, **inline_styles)
: Displays dictionaries.printer.display_table(headers, rows, style="default", **table_options)
: Displays basic tables.printer.display_df(df, style="default", **df_options)
: Displays pandas DataFrames with many options.printer.display_progress(total, desc="", style="default", **progress_options)
: Displays a progress bar.Colab Print offers powerful progress tracking with tqdm compatibility:
from colab_print import progress, Printer
import time
# Simple progress bar using iterable
for i in progress(range(100), desc="Processing"):
time.sleep(0.01) # Do some work
# Manual progress
printer = Printer()
progress_id = printer.display_progress(total=50, desc="Manual progress")
for i in range(50):
time.sleep(0.05) # Do some work
printer.update_progress(progress_id, i+1)
# Progress with customization
for i in progress(range(100),
desc="Custom progress",
color="#9C27B0",
height="25px",
style="card"):
time.sleep(0.01)
# Undetermined progress (loading indicator)
progress_id = printer.display_progress(total=None, desc="Loading...", animated=True)
time.sleep(3) # Do some work with unknown completion time
The display_df
method supports numerous customization options:
printer.display_df(df,
style='default', # Base style
max_rows=20, # Max rows to display
max_cols=10, # Max columns to display
precision=2, # Decimal precision for floats
header_style="...", # Custom header styling
odd_row_style="...", # Custom odd row styling
even_row_style="...", # Custom even row styling
index=True, # Show index
width="100%", # Table width
caption="My DataFrame", # Table caption
highlight_cols=["col1"], # Highlight columns
highlight_rows=[0, 2], # Highlight rows
highlight_cells={(0,0): "..."}, # Highlight specific cells
font_size="14px", # Custom font size for all cells
text_align="center") # Text alignment for all cells
Colab Print automatically detects and optimally displays complex data structures:
from colab_print import list_
import numpy as np
import pandas as pd
# Nested lists are visualized with hierarchical styling
nested_list = [1, 2, [3, 4, [5, 6]], 7]
list_(nested_list)
# Matrices are displayed as tables automatically
matrix = [[1, 2, 3], [4, 5, 6], [7, 8, 9]]
list_(matrix)
# NumPy arrays are handled seamlessly
np_array = np.array([[1, 2, 3], [4, 5, 6]])
list_(np_array)
# Pandas data structures work too
series = pd.Series([1, 2, 3, 4])
list_(series)
# Control display format manually if needed
list_(matrix, matrix_mode=False) # Force list display for a matrix
custom_themes = {
'dark': 'color: white; background-color: #333; font-size: 16px;',
'fancy': 'color: #8A2BE2; font-family: "Brush Script MT", cursive; font-size: 20px;'
}
printer = Printer(additional_styles=custom_themes)
printer.display("Dark theme", style="dark")
printer.display("Fancy theme", style="fancy")
# Create a function with predefined styling
my_header = printer.create_styled_display("header", color="#FF5722", font_size="24px")
# Use it multiple times with consistent styling
my_header("First Section")
my_header("Second Section")
# Still allows overrides at call time
my_header("Special Section", color="#9C27B0")
The library gracefully handles non-IPython environments by printing fallback text representations:
# This will work in regular Python scripts
printer.display_list([1, 2, 3])
printer.display_dict({'a': 1})
printer.display_df(df)
Colab Print includes a comprehensive exception hierarchy for robust error handling:
from colab_print import (
ColabPrintError, # Base exception
StyleNotFoundError, # When a style isn't found
DataFrameError, # DataFrame-related issues
InvalidParameterError, # Parameter validation failures
HTMLRenderingError # HTML rendering problems
)
try:
printer.display("Some text", style="non_existent_style")
except StyleNotFoundError as e:
print(f"Style error: {e}")
For a comprehensive demonstration of all features, please see the example script:
This script covers:
Contributions are welcome! Please feel free to submit a Pull Request.
This project is licensed under the MIT License - see the LICENSE file for details.
FAQs
Enhanced display utilities for Jupyter/Colab notebooks with customizable styles
We found that colab-print demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
The official Go SDK for the Model Context Protocol is in development, with a stable, production-ready release expected by August 2025.
Security News
New research reveals that LLMs often fake understanding, passing benchmarks but failing to apply concepts or stay internally consistent.
Security News
Django has updated its security policies to reject AI-generated vulnerability reports that include fabricated or unverifiable content.