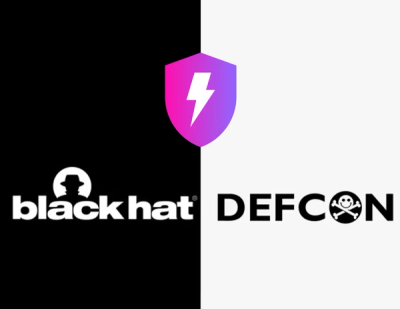
Security News
Meet Socket at Black Hat and DEF CON 2025 in Las Vegas
Meet Socket at Black Hat & DEF CON 2025 for 1:1s, insider security talks at Allegiant Stadium, and a private dinner with top minds in software supply chain security.
Confflow is a robust configuration management library designed for Python projects. It provides seamless management of configurations using YAML files and integrates validation via Pydantic models. Confflow ensures that your configurations are logically consistent and simplifies the process of creating, loading, and saving configuration files.
pip
(Recommended)The easiest way to install Confflow is via pip:
pip install confflow
This will install Confflow and its dependencies.
If you prefer to install from source, you can clone the repository and install the dependencies manually:
Clone the repository
git clone https://github.com/pedrojosemoragallegos/confflow.git
Navigate to the project directory:
cd confflow
Install dependencies
If you use Poetry:
poetry install
Or, if you prefer pip:
pip isntall .
Define configuration schemas using BaseConfig:
from confflow import BaseConfig, Field
from typing import Optional, Literal
class CommonSettings(BaseConfig):
name: str = Field(..., min_length=3, max_length=50, description="The name of the configuration.")
enabled: bool = Field(default=True, description="Indicates if this configuration is enabled.")
priority: int = Field(default=1, ge=1, le=10, description="Priority level, must be between 1 and 10.")
class DatabaseConfig(BaseConfig):
db_url: str = Field(..., pattern=r"^(postgres|mysql|sqlite)://", description="Database connection URL.")
max_connections: int = Field(default=10, ge=1, le=100, description="Max number of connections.")
timeout: Optional[int] = Field(default=30, ge=10, le=300, description="Timeout in seconds.")
class APIConfig(BaseConfig):
endpoint: str = Field(..., pattern=r"^https?://", description="API endpoint URL.")
auth_token: Optional[str] = Field(None, description="Optional authentication token.")
retries: int = Field(default=3, ge=0, le=10, description="Number of retries in case of failure.")
class FeatureFlags(BaseConfig):
experimental_feature: bool = Field(default=False, description="Toggle for experimental feature.")
legacy_mode: bool = Field(default=False, description="Enable legacy mode for backward compatibility.")
# Mutually Exclusive Models
class FileStorageConfig(BaseConfig):
storage_path: str = Field(..., description="Path to the storage directory.")
max_size_mb: int = Field(default=100, ge=10, le=1024, description="Maximum storage size in MB.")
backup_enabled: bool = Field(default=True, description="Enable backup for stored files.")
class CloudStorageConfig(BaseConfig):
provider: Literal['aws', 'gcp', 'azure'] = Field(..., description="Cloud storage provider.")
bucket_name: str = Field(..., min_length=3, description="Name of the cloud storage bucket.")
region: Optional[str] = Field(None, description="Optional region of the cloud storage bucket.")
from confflow import confflow_manager
# No initialisation needed as `confflow_manager`is a module instance (Singelton Module Pattern)
confflow_manager.register_schemas(CommonSettings, FeatureFlags, CloudStorageConfig, DatabaseConfig)
# Set mutually exclusive groups
confflow_manager.set_mutual_exclusive_groups([CloudStorageConfig, DatabaseConfig])
confflow_manager.create_template('template_config.yaml')
This will generate a configuration template like:
CommonSettings:
name: # Type: string Description: The name of the configuration.
enabled: True # Type: boolean Description: Indicates if this configuration is enabled.
priority: 1 # Type: integer Description: Priority level, must be between 1 and 10.
FeatureFlags:
experimental_feature: # Type: boolean Description: Toggle for experimental feature.
legacy_mode: # Type: boolean Description: Enable legacy mode for backward compatibility.
# -------------------------------------
# Mutual exclusive group: Pick only one
# -------------------------------------
CloudStorageConfig:
provider: # Type: string ['aws', 'gcp', 'azure'] Description: Cloud storage provider.
bucket_name: # Type: string Description: Name of the cloud storage bucket.
region: # Types: ['string', 'null'] Description: Optional region of the cloud storage bucket.
DatabaseConfig:
db_url: # Type: string Description: Database connection URL.
max_connections: 10 # Type: integer Description: Max number of connections.
timeout: 30 # Types: ['integer', 'null'] Description: Timeout in seconds.
# -------------------------------------
Once the configuration file is populated, load and access the data:
confflow_manager.load_yaml('filled_config.yaml')
# Access specific configurations
common_settings = confflow_manager["CommonSettings"]
print(f"Configuration Name: {common_settings.name}")
print(f"Priority: {common_settings.priority}")
database_config = confflow_manager["DatabaseConfig"]
print(f"Database URL: {database_config.db_url}")
Save the current configurations back to a YAML file:
confflow_manager.to_yaml('output_config.yaml')
Clone the repository and install dependencies:
git clone https://github.com/pedrojosemoragallegos/confflow.git
cd confflow
poetry install
Run tests:
poetry run pytest
Contributions are welcome! Please follow these steps:
This project is licensed under the MIT License. See the LICENSE file for details.
Developed by Pedro José Mora Gallegos.
FAQs
A configuration manager for Python projects
We found that confflow demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Meet Socket at Black Hat & DEF CON 2025 for 1:1s, insider security talks at Allegiant Stadium, and a private dinner with top minds in software supply chain security.
Security News
CAI is a new open source AI framework that automates penetration testing tasks like scanning and exploitation up to 3,600× faster than humans.
Security News
Deno 2.4 brings back bundling, improves dependency updates and telemetry, and makes the runtime more practical for real-world JavaScript projects.