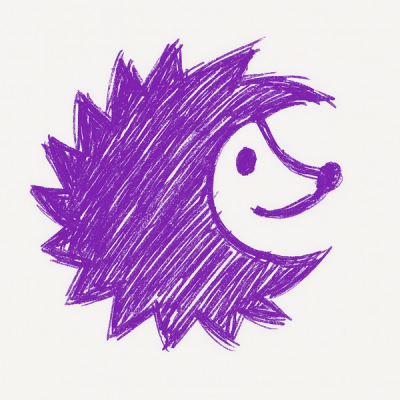
Security News
Browserslist-rs Gets Major Refactor, Cutting Binary Size by Over 1MB
Browserslist-rs now uses static data to reduce binary size by over 1MB, improving memory use and performance for Rust-based frontend tools.
A graph-based data structure designed for querying CSV files in Joern format in Python
cpg2py
is a Python library that provides a lightweight graph-based query engine for analyzing Code Property Graphs (CPG) extracted from Joern CSV files. The library offers an abstract base class (ABC) architecture, allowing users to extend and implement their own custom graph queries.
nodes.csv
and rels.csv
to construct a graph structure.AbcGraphQuerier
for implementing custom graph queries.AbcNodeQuerier
for interacting with nodes.AbcEdgeQuerier
for interacting with edges.**) and outgoing (**
) edges of a node.**) and predecessors (**
).To install the package, use:
pip install git+https://github.com/YichaoXu/cpg2py.git
Or clone the pip repository:
pip install cpg2py
nodes.csv
(Example):id:int labels:label type flags:string_array lineno:int code childnum:int funcid:int classname namespace endlineno:int name doccomment
0 Filesystem Directory "input"
1 Filesystem File "example.php"
2 AST AST_TOPLEVEL TOPLEVEL_FILE 1 "" 25 "/input/example.php"
rels.csv
(Example):start end type
2 3 ENTRY
2 4 EXIT
6 7 ENTRY
6 9 PARENT_OF
from cpg2py import cpg_graph
# Load graph from CSV files
graph = cpg_graph("nodes.csv", "rels.csv")
# Get a specific node
node = graph.node("2")
print(node.name, node.type) # Example output: "/tmp/example.php" AST_TOPLEVEL
# Get a specific edge
edge = graph.edge("2", "3", "ENTRY")
print(edge.type) # Output: ENTRY
# Get all outgoing edges from a node
outgoing_edges = graph.succ(node)
for out_node in outgoing_edges:
print(out_node.id, out_node.name)
# Get all incoming edges to a node
incoming_edges = graph.prev(node)
for in_node in incoming_edges:
print(in_node.id, in_node.name)
# Get top-level file node for a given node
top_file = graph.topfile_node("5")
print(top_file.name) # Output: "example.php"
# Get child nodes in the AST hierarchy
children = graph.children(node)
print([child.id for child in children])
# Get data flow successors
flow_successors = graph.flow_to(node)
print([succ.id for succ in flow_successors])
The following abstract base classes (ABC
) provide interfaces for extending node, edge, and graph querying behavior.
This class defines how nodes interact with the graph storage.
from cpg2py.abc import AbcNodeQuerier
class MyNodeQuerier(AbcNodeQuerier):
def __init__(self, graph, nid):
super().__init__(graph, nid)
@property
def name(self):
return self.get_property("name")
Defines the querying mechanisms for edges in the graph.
from cpg2py.abc import AbcEdgeQuerier
class MyEdgeQuerier(AbcEdgeQuerier):
def __init__(self, graph, f_nid, t_nid, e_type):
super().__init__(graph, f_nid, t_nid, e_type)
@property
def type(self):
return self.get_property("type")
This class provides an interface for implementing custom graph query mechanisms.
from cpg2py.abc import AbcGraphQuerier
class MyGraphQuerier(AbcGraphQuerier):
def node(self, nid: str):
return MyNodeQuerier(self.storage, nid)
def edge(self, fid, tid, eid):
return MyEdgeQuerier(self.storage, fid, tid, eid)
After implementing the abstract classes, you can perform advanced queries:
graph = MyGraphQuerier(storage)
# Query node properties
node = graph.node("5")
print(node.name) # Example Output: "main"
# Query edge properties
edge = graph.edge("5", "6", "FLOWS_TO")
print(edge.type) # Output: "FLOWS_TO"
For a more detail APIs document please see our APIs doc
cpg_graph(node_csv, edge_csv)
: Loads graph from CSV files.graph.node(nid)
: Retrieves a node by ID.graph.edge(fid, tid, eid)
: Retrieves an edge.graph.succ(node)
: Gets successor nodes.graph.prev(node)
: Gets predecessor nodes..name
: Node name..type
: Node type..line_num
: Source code line number..start
: Edge start node..end
: Edge end node..type
: Edge type.This project is licensed under the MIT License.
FAQs
A graph-based data structure designed for querying CSV files in Joern format in Python
We found that cpg2py demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Browserslist-rs now uses static data to reduce binary size by over 1MB, improving memory use and performance for Rust-based frontend tools.
Research
Security News
Eight new malicious Firefox extensions impersonate games, steal OAuth tokens, hijack sessions, and exploit browser permissions to spy on users.
Security News
The official Go SDK for the Model Context Protocol is in development, with a stable, production-ready release expected by August 2025.